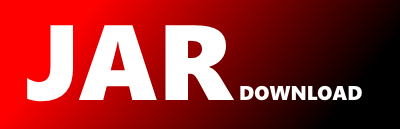
com.meliorbis.economics.model.ModelConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelSolver Show documentation
Show all versions of ModelSolver Show documentation
A library for solving economic models, particularly
macroeconomic models with heterogeneous agents who have model-consistent
expectations
/**
*
*/
package com.meliorbis.economics.model;
import java.io.File;
import java.io.IOException;
import java.util.List;
import com.meliorbis.economics.aggregate.AggregateProblemSolver;
import com.meliorbis.economics.individual.IndividualProblemSolver;
import com.meliorbis.economics.infrastructure.simulation.SimState;
import com.meliorbis.numerics.generic.MultiDimensionalArray;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.io.NumericsReader;
import com.meliorbis.numerics.io.NumericsWriter;
/**
* An interface to be implemented by classes that hold model configuration information
*
* @author Tobias Grasl
*/
public interface ModelConfig
{
static final int[] EMPTY_ARRAY = new int[0];
/**
* Indicates whether individuals in the model are constrained at the lower bound of the individual state array
*
* @return True if constrained, false otherwise
*/
boolean isConstrained();
/**
* @return The list of arrays of potential values for exogenous individual shocks, one for
* each such shock in the model
*/
List> getIndividualExogenousStates();
/**
* @return The list of arrays of potential values for endogenous individual states, one for
* each such state in the model
*/
List> getIndividualEndogenousStates();
/**
* @return The list of arrays of potential values for endogenous individual states to be used in
* simulation, one for each such state in the model
*/
List> getIndividualEndogenousStatesForSimulation();
/**
* @return The list of arrays of potential values for exogenous aggregate shocks, one for each
* such shock in the model
*/
List> getAggregateExogenousStates();
/**
* @return The list of arrays of potential values for aggregate controls, one for each
* such variable in the model
*/
List> getAggregateControls();
/**
* Gets the target value for the control determinant by value of the control, for each control.
* Note that by implication the determinants can't be mutually dependent.
*
* The default assumes that the determinant is just the implied value of the control, and hence
* the targets are the assumed value of the control.
*
* @return The target values that the determinants must equal at each possible value for each control
*/
List> getControlTargets();
/**
* @return The list of arrays of potential values for endogenous aggregate states, one for
* each such state in the model
*/
List> getAggregateEndogenousStates();
/**
* @return The list of arrays of potential values for exogenous aggregate states used for normalisation,
* commonly permanent shocks, one for each such state in the model
*/
List> getAggregateNormalisingExogenousStates();
/**
* @return Array with probabilities of moving from any given individual state in any given aggregate state to any
* combination of future individual, aggregate and normalising states.
*/
DoubleArray> getExogenousStateTransition();
/**
* @return The directory in which to store the solution to the model
*/
File getSolutionDirectory();
/**
* @return The number of aggregate exogenous states configured
*/
int getAggregateExogenousStateCount();
/**
* @return The number of aggregate exogenous states configured
*/
int getAggregateControlCount();
/**
* @return The number of aggregate endogenous states configured
*/
int getAggregateEndogenousStateCount();
/**
* @return The number of normalising aggregate exogenous states configured
*/
int getAggregateNormalisingStateCount();
/**
* @return The number of individual endogenous states configured
*/
int getIndividualEndogenousStateCount();
/**
* @return The number of individual exogenous states configured
*/
int getIndividualExogenousStateCount();
/**
* Writes the parameters of the model to the writer. This should include any of the information not already written
* by GridSolver.writeState
*
* @param writer_ The writer to write to
*
* @throws IOException If there is a problem writing the parameters
*/
void writeParameters(NumericsWriter writer_) throws IOException;
/**
* Reads model parameters from the reader provided. This should include all information necessary to run the model
* that is not already read by GridSolver.readState
*
* @param reader_ The reader to read from
*
* @throws IOException If there is a problem reading the parameters
*/
void readParameters(NumericsReader reader_) throws IOException;
/**
* @return The solver to be used for solving the aggregate problem
*/
Class extends IndividualProblemSolver>> getIndividualSolver();
/**
* @return The solver to be used for solving the aggregate problem
*/
Class extends AggregateProblemSolver>> getAggregateProblemSolver();
/**
* @return The initial simulation state
*/
SimState getInitialSimState();
/**
* @return The initial exogenous states to use in simulation
*
* @param The numeric type of aggregate shocks used in this model
*/
MultiDimensionalArray getInitialExogenousStates();
/**
* Indicates whether this configuration includes aggregate uncertainty
*
* @return True if there is aggregate uncertainty, false otherwise
*/
boolean hasAggUncertainty();
/**
* Indicates whether this configuration includes individual uncertainty
*
* @return True if there is individual uncertainty, false otherwise
*/
boolean hasIndUncertainty();
/**
* The array of indexes of aggregate states which are known with certainty one period in
* advance, given current aggregate states and controls (which affect expectations).
*
* By default no aggregates have this feature, so an empty array is returned.
*
* @return The array of indexes
*/
default int[] getAggregatesKnownWithCertainty() { return EMPTY_ARRAY; };
/**
* The array of indexes of aggregate controls which affect expectations.
*
* By default no controls affect expectations, so an empty array is returned.
*
* @return The array of indexes
*/
default int[] getControlsAffectingExpectations() { return EMPTY_ARRAY; };
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy