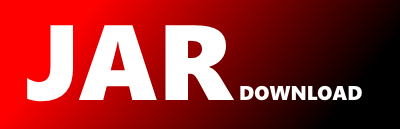
com.meliorbis.economics.utils.FileUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelSolver Show documentation
Show all versions of ModelSolver Show documentation
A library for solving economic models, particularly
macroeconomic models with heterogeneous agents who have model-consistent
expectations
/**
*
*/
package com.meliorbis.economics.utils;
import java.io.File;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
/**
* Some utility functions for dealing with files
*
* @author toby
*/
public abstract class FileUtils
{
private static final File DATA_DIR = new File(
System.getProperty("com.meliorbis.files",
System.getProperty("user.home")+"/Documents/Research/PhD/LifeCycle/uncertainty/data"));
public static final DateFormat DATE_FORMAT = new SimpleDateFormat("dd_MM_HH_mm");
/**
* Gets the path to a file in the application's data directory
*
* @param fileName_ The path to the file, relative to the data directory
*
* @return The absolute path to the file
*/
public static String getDataFilePath(String fileName_)
{
return new File(DATA_DIR,fileName_).getAbsolutePath();
}
/**
* Creates a directory of the form '<prefix>_<date_time>' in the directory '<parent>'
*
* @param parent_ The directory in which to place the new directory
* @param prefix_ The prefix to use
*
* @return A reference to the new directory, which will have been created
*/
public static File createDatedDirectory(File parent_, String prefix_)
{
// Create the file object
File directoryToWrite = createDatedFile(parent_,prefix_);
// And make the directory
directoryToWrite.mkdirs();
// Then return it
return directoryToWrite;
}
/**
* Creates a file with a name of the form '<prefix>_<date_time>[.<extension>]'
* in the directory '<parent>'. The extension is optional and, if not present, the '.' will also not be placed
*
* @param parent_ The directory in which to place the new directory
* @param prefix_ The prefix to use
* @param extension_ The extension to use
*
* @return A file with the specified path and name
*/
public static File createDatedFile(File parent_, String prefix_, String extension_)
{
File dateFile = new File(parent_, createDatedFileName(prefix_, extension_));
dateFile.getParentFile().mkdirs();
return dateFile;
}
/**
* Creates a name of the form '<prefix>_<date_time>[.<extension>]'. The extension is optional
* and, if not present, the '.' will also not be placed
*
* @param prefix_ The part before the date
* @param extension_ The extension to use
*
* @return The created name
*/
public static String createDatedFileName(String prefix_, String extension_)
{
String fileName;
final String dateString = formattedCurrentDate();
if(extension_ == null || extension_.length() == 0)
{
fileName = String.format("%s_%s", prefix_, dateString);;
}
else
{
fileName = String.format("%s_%s.%s", prefix_, dateString, extension_);
}
return fileName;
}
/**
* Creates a name of the form '<prefix>_<date_time>'
*
* @param prefix_ The part before the date
*
* @return The created name
*/
public static String createDatedFileName(String prefix_)
{
return createDatedFileName(prefix_, null);
}
private static String formattedCurrentDate()
{
return DATE_FORMAT.format(new Date());
}
/**
* Creates a file with a name of the form '<prefix>_<date_time>' in the directory '<parent>'
*
* @param parent_ The directory in which to place the new file
* @param prefix_ The prefix to use
*
* @return A file with the specified path and name
*/
public static File createDatedFile(File parent_, String prefix_)
{
return createDatedFile(parent_, prefix_,null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy