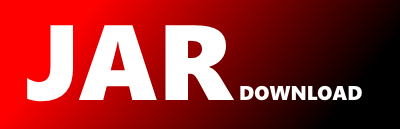
com.meliorbis.numerics.DoubleArrayFactories Maven / Gradle / Ivy
package com.meliorbis.numerics;
import java.util.List;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
/**
* Provides static factory methods for creating double arrays
*
* @author Tobias Grasl
*/
public final class DoubleArrayFactories
{
// Prevent construction
private DoubleArrayFactories() {}
private static final DoubleNumerics _numerics = DoubleNumerics.instance();
/**
* Creates an array with the provided dimensions
*
* @param size_ The dimensions of the array to be created
*
* @return An array with the give size and all values at the default
*/
static public DoubleArray> createArrayOfSize(List size_)
{
return _numerics.getArrayFactory().newArray(size_);
}
/**
* Creates an array with the provided dimensions
*
* @param size_ The dimensions of the array to be created
*
* @return An array with the give size and all values at the default
*/
static public DoubleArray> createArrayOfSize(int... size_)
{
return _numerics.getArrayFactory().newArray(size_);
}
/**
* Creates a one dimensional array of the same length as the input
* arguments, and which is initialised to contain those arguments
*
* @param values_ The values to store in the array
*
* @return The newly created and filled array
*/
static public DoubleArray> createArray(double... values_)
{
return _numerics.getArrayFactory().newArray(values_);
}
// protected IntegerArray> createIntArray(List size_)
// {
// return getNumerics().newIntArray(size_);
//
// }
// protected IntegerArray> createIntArrayBySize(int... size_)
// {
// return getNumerics().newIntArray(size_);
// }
//
// protected IntegerArray> createIntArray(int... values_)
// {
// return getNumerics().newIntArray(values_.length).fill(ArrayUtils.toObject(values_));
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy