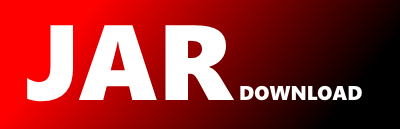
com.meliorbis.numerics.generic.GenericArray Maven / Gradle / Ivy
package com.meliorbis.numerics.generic;
/**
* Interface for generic arrays with generic accessors
*
* @param The numeric type of the array
* @param The result type from operations performed on the array
*/
public interface GenericArray> extends MultiDimensionalArray
{
/**
* Gets the element at the specified index
*
* @param indices_ The index of the element to get
*
* @return The requested element
*/
public T get(int... indices_);
/**
* Gets the first element in the array
*
* @return The element in the linear index 0 position
*/
public T first();
/**
* Gets the last element of the array
*
* @return The element with the highest linear index
*/
public T last();
/**
* Sets the element at the specified position to the specified value
*
* @param val_ The value to set
* @param indices_ The index to set the value at
*/
void set(T val_,int... indices_);
/**
* Sets the elements that match the provided value
*
* @param matching_ The value to match with
* @param val_ The new value to assign
*/
void setMatching(T matching_, T val_);
/**
* Sets the element at the specified position to the specified value
*
* @param matcher_ The matcher to match with
* @param val_ The new value to set
*/
void setMatching(Matcher matcher_, T val_);
/**
* Fill the array with the given data in iteration order
*
* @param data_ The data to fill with
*
* @return Itself, for chaining
*/
R fill(@SuppressWarnings("unchecked") T... data_);
/**
* Fills along the given dimension with the provided values, which must be
* the same size as that dimension
*
* @param values_ The values to fill with, which must be of the correct size
* @param dimensions_ The dimensions along which to fill, e.g. {1} would replicate
* the given values across each row
*/
void fillDimensions(T[] values_, int... dimensions_);
/**
* Calculate the sum over all elements in the array
*
* @return The sum of values held in the array
*/
public T sum();
/**
* Calculates the mean under the assumption that the array holds a density fn and the
* levels passed are the domain of that density function.
*
* @param levels_ The values to assign to each point in the specific dimension
* @param dimension_ The dimension along with to perform the calculation
*
* @return The mean value given the inputs
*/
public T mean(MultiDimensionalArray extends T, ?> levels_, int dimension_);
/**
* Calculates the second moment under the assumption that the array holds a density fn and the
* levels passed are the domain of that density function.
*
* @param levels_ The values to assign to each point in the specific dimension
* @param dimension_ The dimension along with to perform the calculation
*
* @return The second moment given the inputs
*/
public T secondMoment(MultiDimensionalArray extends T, ?> levels_, int dimension_);
/**
* Multiply the array by the specified scalar value
*
* @param multiplicand_ The scalar to multiply by
*
* @return The multiplied array
*/
public R multiply(T multiplicand_);
/**
* Add to the array the specified scalar value
*
* @param summand_ The scalar to add
*
* @return The resulting array
*/
public R add(T summand_);
/**
* Multiply the array by the provided scalar value
*
* @param denominator_ The scalar to divide by
*
* @return The multiplied array
*/
public R divide(T denominator_);
/**
* Add to the array the specified scalar value
*
* @param subtrahend_ The scalar to subtract
*
* @return The resulting array
*/
public R subtract(T subtrahend_);
/**
* Returns a one-dimensional array of the underlying type, with the data in linear indexed form
*
* @return The array containing all the data
*/
T[] toArray();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy