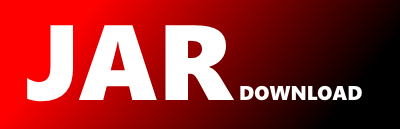
com.meliorbis.numerics.generic.IntegerArray Maven / Gradle / Ivy
package com.meliorbis.numerics.generic;
/**
* Interface for classes that represent a multi-dimensional array of primitive doubles
*
* @param The type returned by operations on this array
*
* @author Tobias Grasl
*/
public interface IntegerArray> extends MultiDimensionalArray, Acrossable
{
/**
* Gets the element at the specified position
*
* @param indices_ The index to get the value from
*
* @return The double value at the specified index
*/
Integer get(int... indices_);
/**
* Gets the first element in the array
*
* @return The element at linear index 0
*/
Integer first();
/**
* Gets the last element in the array, in linear index order
*
* @return The element with the highest linear index
*/
Integer last();
/**
* Sets the element at the specified position to the specified value
*
* @param val_ The value to set
* @param indices_ The index at which the value is to be set
*/
void set(Integer val_,int... indices_);
/**
* Performs a point-by-point multiplication of this array against the provided number
*
* @param other_ Number to multiply each element with
*
* @return A pointwise product of this and other
*/
R multiply(Integer other_);
/**
* The point-wise sum of this multi-dimensional array and other
*
* @param other_ The number to add to each element
*
* @return The sum of the two arrays
*/
@SuppressWarnings("all")
R add(Integer other_);
/**
* Performs a point-by-point division of this array by the provided number
*
* @param other_ Number to divide each element by
*
* @return A pointwise quotient of this and other
*/
R divide(Integer other_);
/**
* The point-wise difference of this multi-dimensional array and other
*
* @param other_ The number to subtract from each element
*
* @return The pointwise difference of this array and other_
*/
R subtract(Integer other_);
/**
* Fill the array with the provided values
*
* @param values_ The values to fill the array with
*
* @return A reference to this
*/
R fill(Integer... values_);
/**
* Returns a one-dimensional array of the underlying type, with the data in linear indexed form
*
* @return The array containing all the data
*/
Integer[] toArray();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy