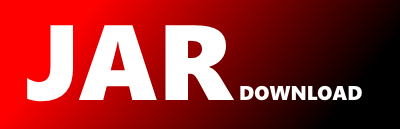
com.meliorbis.numerics.generic.MappableWithSimpleOps Maven / Gradle / Ivy
package com.meliorbis.numerics.generic;
/**
* An extension of IMappable that provides basic arithmetic operations
*
* @param The individual element type
* @param The return type of mapping operations, which will commonly be the most derived type. This parameter is
* used to avoid the need to cast
*/
public interface MappableWithSimpleOps> extends Mappable
{
/**
* @return A reference to this mappable that causes the results of any mappings
* applied to be stored in this object itself
*/
MappableWithSimpleOps> modifying();
/**
* @return A reference to this mappable that does not cause the results of any mappings
* applied to be stored in this object
*/
MappableWithSimpleOps> nonModifying();
/**
* Performs a point-by-point multiplication of two equally sized arrays and
* returns the result
*
* @param other_ Array to multiply with
*
* @param The type of the array being multiplied in
*
* @return A pointwise product of this and other
*/
RM multiply(MultiDimensionalArray other_);
/**
* The point-wise quotient of this multi-dimensional array and other
*
* @param other_ The operand to divide this one by
*
* @param The type of the array being divided by
*
* @return The quotient of this operand divided by other
*/
RM divide(MultiDimensionalArray other_);
/**
* The point-wise sum of this multi-dimensional array and other
*
* @param other_ The operand to add to this one
*
* @param The type of the array being added
*
* @return The sum of the two operands
*/
RM add(MultiDimensionalArray other_);
/**
* The point-wise difference between this multi-dimensional array and other
*
* @param other_ The operand to subtract from this one
*
* @param The type of the array being subtracted
*
* @return The difference between this operand and other
*/
RM subtract(MultiDimensionalArray other_);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy