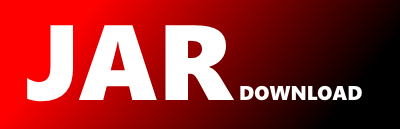
com.meliorbis.numerics.generic.MultiDimensionalArray Maven / Gradle / Ivy
/**
*
*/
package com.meliorbis.numerics.generic;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
/**
* Interface for classes that represent a multi-dimensional array
*
* @author Tobias Grasl
*/
public interface MultiDimensionalArray> extends MappableReducible
{
/**
* @return The total number of elements in the array, i.e. the
* product of the dimensions
*/
int numberOfElements();
/**
* @return The number of dimensions in this array
*/
int numberOfDimensions();
/**
* @return The size of the array along each dimension
*/
int[] size();
/**
* Returns a new array with the same structure and data as this one, all deep copied,
* but does not maintain knowledge about whether this is a subarray or not
*
* @return The new array with the copied data
*/
R copy();
/**
* Transposes the specified dimensions
*
* @param dimA_ The first dimension to transpose with...
* @param dimB_ ... the second dimension
*
* @return The same array, but transposed
*/
R transpose(int dimA_, int dimB_);
/**
* Arranges the dimensions in the newly specified order
*
* @param order_ The order in which the dimensions are to be arranged, e.g. [3 2 1] would reverse the order of a three-d array
*
* @return This array but with the dimensions rearranged
*/
R arrangeDimensions(int[] order_);
/**
* Fills the entire array with the given data, which must be of the same size
*
* @param values_ The values to fill with, which must be of the correct size
*
* @return This array (for chaining)
*/
R fill(Iterator extends T> values_);
/**
* Fills the entire array with the given data, which must be of the same size
*
* @param values_ The values to fill with, which must be of the correct size
*
* @return This array (for chaining)
*/
R fill(MultiDimensionalArray extends T, ?> values_);
/**
* Fills along the given dimension with the provided values, which must be
* the same size as that dimension
*
* @param values_ The values to fill with, which must be of the correct size
* @param dimensions_ The dimensions along which to fill, e.g. {1} would replicate
* the given values across each row
*/
void fillDimensions(MultiDimensionalArray extends T, ?> values_, int... dimensions_);
/**
* Fills along the unspecified dimensions at the points for which an index is specified. Dimensions to be filled are indicated
* with -1 or dropped if they come at the end
*
* @param values_ The values to fill with, which must be of the correct size
* @param index_ The index of the subarray at which to fill
*/
void fillAt(MultiDimensionalArray extends T, ?> values_, int... index_);
/**
* Right-multiplies this matrix by the other (i.e. this * other).
*
* @param other_ The matrix to multiply by
*
* @return The result of the multiplication
*/
R matrixMultiply(MultiDimensionalArray extends T, ?> other_);
/**
* Right-multiplies this matrix by other, transforming the outcome of each individual multiplication as it progesses
*
* @param other_ Matrix to multiply by
* @param transformer_ Transformation to apply to individual element products
*
* @return The product of the matrices, with the transformation subsequently applied
*/
R matrixMultiply(MultiDimensionalArray extends T, ?> other_, UnaryOp transformer_);
/**
* @return Returns an appropriate comparator for type T
*/
Comparator getComparator();
/**
* Provides a sub-array view on the same underlying data (i.e. does not copy the data) that covers all the
* points matching the indicated subIndex; the subIndex has the same dimenion order as the main one, non-set dimensions (ie. ones
* returned in the result) are indicated with -1 or dropped off the end
*
* @param subIndex_ The position at which to get a subarray
*
* @return An Implementation of
*/
R at(int... subIndex_);
/**
* Selects the subarray at a single index in the highest dimension. This is particularly useful if the array holds
* different variables, which are indexed across the highest dimension
*
* @param index_ The index along the highest dimension at which to choose the slice
*
* @return The relevant slice
*/
R lastDimSlice(int index_);
/**
* Returns an iterator over the entire array in index order (i.e. (0,0,...,0,0),(0,0,...,0,1),...)
*
* @return An iterator over the array
*/
SettableIndexedIterator iterator();
/**
* Returns an iterator that will iterate across the given dimensions but leave
* the others untouched. To be used with an orthogonal iterator
*
* @param dimensions_ The dimensions to iterate over
*
* @return An appropriate iterator
*/
SubSpaceSplitIterator iteratorAcross(int[] dimensions_);
/**
* Returns an iterator that will iterate over the dimensions orthogonal to those for
* which a value is provided in index. For example, in a three-dimensional array with
* index given as {2,-1,3}, the iterator would iterate over the second dimension, with the
* first and third dimension at the given index: {2,0,3},{2,1,3},...,{2,n2,3}.
*
* @param index_ The index at which to iterate
*
* @return An appropriate iterator
*/
SubSpaceSplitIterator iteratorAt(int... index_);
/**
* See IMultiDimensionalArray.iteratorAt This function does the same but with a
* static rather than dynamic array
*
* @param index_ The index at which to iterate
* @return An appropriate iterator
*/
SubSpaceSplitIterator iteratorAtArray(int[] index_);
/**
* Provides an iterator over the provided range of linear indexes
*
* @param from_ The linear index at which to start (inclusive)
* @param to_ The linear index to which to run (exclusive)
*
* @return A iterator over the specified range
*/
SettableIndexedIterator rangeIterator(int from_, int to_);
/**
* Returns an array of iterators, each of which iterates over the provided dimensions, but using a different point in
* the other dimensions. One iterator is returned for each point in the other dimensions
*
* @param dimensions_ The dimensions to iterate over in parallel
*
* @return The iterators as described
*/
List extends SettableIterator> parallelIterators(int[] dimensions_);
/**
* Returns a new array which combines all the arrays in the provided order, with this one first. The new array has one extra
* dimension over this of size 1+others_.length
*
* @param others_ The other arrays to stack with this one, which must all be of the same size as this
*
* @return The combined array
*
* @throws MultiDimensionalArrayException If there are problems performing the operation
*/
R stack(@SuppressWarnings("unchecked") MultiDimensionalArray extends T, ?>... others_);
/**
* Returns a new array which combines all the arrays in the provided order, with this one first. The new array has the same number of dimensions
* as this, but the highest dimension is the sum of the highest dimension of this and all the input arrays
*
* @param others_ The other arrays to stack with this one, which must all be of the same size as this (except in the highest dimension)
*
* @return The combined array
*/
R stackFinal(@SuppressWarnings("unchecked") MultiDimensionalArray extends T, ?>... others_);
/**
* Returns an reference to itself but when when appropriate operations are performed on that reference, the
* array itself is updated rather than returning a new result
*
* @return A modifying self-reference
*/
MappableWithSimpleOps> modifying();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy