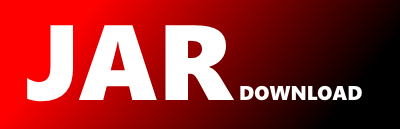
com.meliorbis.numerics.generic.impl.GenericBlockedArrayData Maven / Gradle / Ivy
package com.meliorbis.numerics.generic.impl;
import java.util.Arrays;
import com.meliorbis.numerics.generic.ArrayFactory1D;
import com.meliorbis.numerics.generic.ArrayFactory2D;
import com.meliorbis.numerics.generic.MultiDimensionalArrayException;
/**
* Parent class for generic implementations of BlockedArrayData
*/
public class GenericBlockedArrayData extends BlockedArrayData
{
T[][] _data;
final private ArrayFactory1D _oneDArrayFactory;
final private ArrayFactory2D _twoDArrayFactory;
final T _zero;
/**
* Copy-constructor
*
* @param other_ The array-data to copy from
* @param zero_ The zero-value of this array
*/
protected GenericBlockedArrayData(GenericBlockedArrayData other_, T zero_)
{
this(other_._data, other_._logicalIndex.getSizes(), zero_, other_._oneDArrayFactory, other_._twoDArrayFactory);
}
protected GenericBlockedArrayData(T[] data_, int[] dimensions_, T zero_,
ArrayFactory1D oneDArrayFactory_, ArrayFactory2D twoDArrayFactory_)
{
this(DEFAULT_BLOCK_SIZE, dimensions_, zero_, oneDArrayFactory_, twoDArrayFactory_);
for(int i = 0; i < _data.length; i++)
{
_data[i] = Arrays.copyOfRange(data_, i*_blockSize, i*_blockSize + getBlockSize(i));
}
}
protected GenericBlockedArrayData(int[] dimensions_,T zero_, ArrayFactory1D oneDArrayFactory_, ArrayFactory2D twoDArrayFactory_)
{
this(DEFAULT_BLOCK_SIZE, dimensions_, zero_, oneDArrayFactory_, twoDArrayFactory_);
}
protected GenericBlockedArrayData(int blockSize_, int[] dimensions_, T zero_,
ArrayFactory1D oneDArrayFactory_, ArrayFactory2D twoDArrayFactory_)
{
super(blockSize_, dimensions_);
_zero = zero_;
_oneDArrayFactory = oneDArrayFactory_;
_twoDArrayFactory = twoDArrayFactory_;
_data = _twoDArrayFactory.createArray(_numBlocks);
for(int blockNum = 0; blockNum < _data.length; blockNum++)
{
_data[blockNum] = _data[blockNum] = _oneDArrayFactory.createArray(getBlockSize(blockNum));
}
}
private GenericBlockedArrayData(T[][] data_, int[] sizes_, T zero_,
ArrayFactory1D oneDArrayFactory_, ArrayFactory2D twoDArrayFactory_) {
this(DEFAULT_BLOCK_SIZE, sizes_, zero_, oneDArrayFactory_, twoDArrayFactory_);
for(int i = 0; i < _data.length; i++)
{
_data[i] = Arrays.copyOf(data_[i], data_[i].length);
}
}
public void setLinear(T val_, int linearIndex_)
{
int[] physicalIndex = _physicalIndex.toLogicalIndex(linearIndex_);
set(physicalIndex[0], physicalIndex[1], val_);
}
public T getLinear(int linearIndex_)
{
int[] physicalIndex = _physicalIndex.toLogicalIndex(linearIndex_);
return get(physicalIndex[0],physicalIndex[1]);
}
protected T get(int[] indices_) throws MultiDimensionalArrayException
{
int[] physicalIndex = logicalToPhysical(indices_);
return get(physicalIndex[0],physicalIndex[1]);
}
/**
* Retrieves the value at the given physical index
*
* @param block_ The block the item is in
* @param index_ The index the item is at
*
* @return The value at the given index. Never null.
*/
protected T get(int block_, int index_)
{
// Check whether the relevant block exists
if(_data[block_][index_] == null)
{
// It does not - return a zero
return _zero;
}
return _data[block_][index_];
}
public void set(T val_, int[] indices_)
{
int[] physicalIndex = logicalToPhysical(indices_);
set(physicalIndex[0], physicalIndex[1], val_);
}
/**
* Sets the item at the indicated physical position to the provided value
*
* @param blockNum The block in which the item is
* @param index The index of the item in the block
*
* @param val_ The value to set
*/
protected void set(int blockNum, int index, T val_)
{
_data[blockNum][index] = val_;
}
public T[] toArray()
{
T[] data = _oneDArrayFactory.createArray(_logicalIndex.numberOfElements());
int blockStart = 0;
for(int block = 0; block < _numBlocks; block++)
{
int currentBlockSize = getBlockSize(block);
System.arraycopy(_data[block], 0, data, blockStart, currentBlockSize);
blockStart += currentBlockSize;
}
// Need to replace nulls with 0s
for (int i = 0; i < data.length; i++)
{
if(data[i] == null) data[i] = _zero;
}
return data;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy