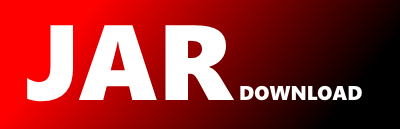
com.meliorbis.numerics.generic.primitives.DoubleArray Maven / Gradle / Ivy
package com.meliorbis.numerics.generic.primitives;
import java.util.List;
import com.meliorbis.numerics.NumericsException;
import com.meliorbis.numerics.generic.Acrossable;
import com.meliorbis.numerics.generic.MappableReducible;
import com.meliorbis.numerics.generic.MultiDimensionalArray;
import com.meliorbis.numerics.generic.primitives.impl.DoubleArray.IDoubleModifyableMappable;
/**
* Interface for classes that represent a multi-dimensional array of primitive doubles
*
* @param The type returned by operations on this array
*
* @author Tobias Grasl
*/
public interface DoubleArray> extends MultiDimensionalArray, Acrossable
{
/**
* Gets the element at the specified position
*
* @param indices_ The index to get the value from
*
* @return The double value at the specified index
*/
double get(int... indices_);
/**
* Gets the first element in the array
*
* @return The element at linear index 0
*/
double first();
/**
* Gets the last element in the array, in linear index order
*
* @return The element with the highest linear index
*/
double last();
/**
* Sets the element at the specified position to the specified value
*
* @param val_ The value to set
* @param indices_ The index at which the value is to be set
*/
void set(double val_,int... indices_);
/**
* Sets the elements that match the provided value
*
* @param matching_ The value to match
* @param val_ The value to replace matching elements by
*/
void setMatching(double matching_, double val_);
/**
* Performs a point-by-point multiplication of this array against the provided number
*
* @param other_ Number to multiply each element with
*
* @return A pointwise product of this and other
*/
R multiply(double other_);
/**
* The point-wise sum of this multi-dimensional array and other
*
* @param other_ The number to add to each element
*
* @return The sum of the two arrays
*/
R add(double other_);
/**
* Performs a point-by-point division of this array by the provided number
*
* @param other_ Number to divide each element by
*
* @return A pointwise quotient of this and other
*/
R divide(double other_);
/**
* The point-wise difference of this multi-dimensional array and other
*
* @param other_ The number to subtract from each element
*
* @return The pointwise difference of this array and other_
*/
R subtract(double other_);
/**
* Fill the array with the given data in iteration order
*
* @param data_ The data to fill with
*
* @return Itself, for chaining
*/
R fill(double... data_);
/**
* Fills along the given dimension with the provided values, which must be
* the same size as that dimension
*
* @param values_ The values to fill with, which must be of the correct size
* @param dimensions_ The dimensions along which to fill, e.g. {1} would replicate
* the given values across each row
*/
void fillDimensions(double[] values_, int... dimensions_);
/**
* Under the assumption that the array is a distribution, calculates the mean of the distribution
* assuming levels along the given dimension
*
* @param levels_ The level at each point along a the given dimension
* @param dimension_ The dimension along which to calculate the mean
*
* @return The mean
*/
double mean(final DoubleArray> levels_, int dimension_);
/**
* Under the assumption that the array is a distribution, calculates the mean of the distribution
* assuming levels along the given dimension
*
* @param levels_ The level at each point along a the given dimension
* @param dimension_ The dimension along which to calculate the mean
*
* @return The mean
*/
double secondMoment(final DoubleArray> levels_, int dimension_);
/**
* Calculates the inverse matrix for a two dimensional array.
*
* @return The inverse matrix
*
* @throws NumericsException If an error occurs or the matrix is not 2 dimensional
*/
DoubleArray inverseMatrix() throws NumericsException;
/**
* Additional reduce method yielding a primitive double
*
* @param reduction_ The reduction to perform
*
* @param The type of exception the reduction throws
*
* @return The result of the reduction
*
* @throws E If there are errors performing the reduction
*/
double reduce(DoubleReduction reduction_) throws E;
/**
* Returns a one-dimensional array of the underlying type, with the data in linear indexed form
*
* @return The array containing all the data
*/
double[] toArray();
/*
* Return type specialisations
*/
IDoubleModifyableMappable modifying();
DoubleSettableIndexedIterator iterator();
DoubleSubspaceSplitIterator iteratorAcross(int[] dimensions_);
List parallelIterators(int[] dimensions_);
DoubleSubspaceSplitIterator iteratorAt(int... index_);
DoubleSubspaceSplitIterator iteratorAtArray(int[] index_);
DoubleSettableIndexedIterator rangeIterator(int from_, int to_);
//IMappable with(IDoubleArray>... otherArrays_);
R at(int... index_);
MappableReducible across(int... dimensions_);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy