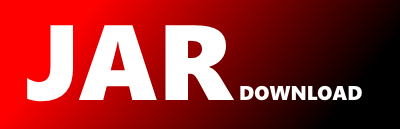
com.meliorbis.numerics.generic.primitives.impl.DoubleBlockedArrayData Maven / Gradle / Ivy
package com.meliorbis.numerics.generic.primitives.impl;
import com.meliorbis.numerics.generic.MultiDimensionalArrayException;
import com.meliorbis.numerics.generic.impl.BlockedArrayData;
import com.meliorbis.numerics.generic.primitives.DoubleSettableIndexedIterator;
import com.meliorbis.numerics.generic.primitives.DoubleSettableIterator;
import com.meliorbis.numerics.generic.primitives.DoubleSubspaceSplitIterator;
import com.meliorbis.numerics.index.SkippingLinearIterator;
import com.meliorbis.numerics.index.SubIndexIterator;
import com.meliorbis.numerics.index.impl.Index.SubSpaceSplitIterator;
/**
* Specialisation of DoubleBlockedArrayData for primitive doubles
*/
public final class DoubleBlockedArrayData extends BlockedArrayData
{
final double[][] _data;
public DoubleBlockedArrayData(int[] dimensions_)
{
super(dimensions_);
_data = allocateData();
}
/**
* @return The array of allocated data
*/
protected double[][] allocateData()
{
// double[][] data = new double[_numBlocks][];
return (double[][]) java.lang.reflect.Array.newInstance(double.class, _numBlocks, _blockSize );
// for(int blockNum = 0; blockNum < data.length; blockNum++)
// {
// data[blockNum] = new double[getBlockSize(blockNum)];
// }
// return data;
}
@Override
protected BlockedDataSubSpaceSplitIterator createSubSpacedIterator(SubIndexIterator logicalIterator_)
{
return new BlockedDoubleDataSubspaceSplitIterator(logicalIterator_);
}
@Override
protected BlockedDataSettableIterator createBlockedDataSettableIterator(SkippingLinearIterator indexIterator_)
{
return new BlockedDoubleDataSettableIterator(indexIterator_);
}
protected BlockedDoubleDataIterator iterator()
{
return new BlockedDoubleDataIterator();
}
protected BlockedDoubleDataIterator rangeIterator(int from_, int to_)
{
return new BlockedDoubleDataIterator(from_, to_);
}
public DoubleBlockedArrayData(int blockSize_, int[] dimensions_)
{
super(blockSize_, dimensions_);
_data = allocateData();
}
@Override
protected Double get(int block_, int index_)
{
throw new UnsupportedOperationException("Use getDouble");
}
@Override
protected void set(int i, int i1, Double val_)
{
throw new UnsupportedOperationException("Use set(...,double)");
}
public void set(double val_, int[] indices_)
{
final int[] blockAndElement = logicalToPhysical(indices_);
set(blockAndElement[0], blockAndElement[1], val_);
}
public void setLinear(int linearIndex_, double val_)
{
int[] physicalIndex = _physicalIndex.toLogicalIndex(linearIndex_);
set(physicalIndex[0],physicalIndex[1], val_);
}
public double getLinear(int linearIndex_)
{
int[] physicalIndex = _physicalIndex.toLogicalIndex(linearIndex_);
return getDouble(physicalIndex[0],physicalIndex[1]);
}
public double get(int[] indices_) throws MultiDimensionalArrayException
{
int[] physicalIndex = logicalToPhysical(indices_);
return getDouble(physicalIndex[0],physicalIndex[1]);
}
/**
* Retrieves the double stored at a given physical address
*
* @param block_ The block in which the element is
* @param index_ The index within the block of the required element
*
* @return The element stored at the provided index
*/
protected double getDouble(int block_, int index_)
{
return _data[block_][index_];
}
/**
* Sets the item at the indicated physical position to the provided value
*
* @param blockNum The block in which the item is
* @param index The index of the item in the block
*
* @param val_ The value to set
*/
protected void set(int blockNum, int index, double val_)
{
_data[blockNum][index] = val_;
}
private class BlockedDoubleDataIterator extends BlockedDataIterator implements DoubleSettableIndexedIterator
{
public BlockedDoubleDataIterator() {}
public BlockedDoubleDataIterator(int from_, int to_)
{
super(from_, to_);
}
@Override
public double nextDouble()
{
// need to keep track of the number of elements
// because the physical iterator doesn't know
// when to stop
_count = _iterator.nextInt();
return getDouble();
}
@Override
public void set(double val_)
{
int[] index = _iterator.getCurrentIndex();
DoubleBlockedArrayData.this.set(index[0],index[1],val_);
}
final public double getDouble()
{
int[] index = _iterator.getCurrentIndex();
return DoubleBlockedArrayData.this.getDouble(index[0], index[1]);
}
}
private class BlockedDoubleDataSettableIterator extends BlockedDataSettableIterator implements DoubleSettableIterator
{
BlockedDoubleDataSettableIterator(SkippingLinearIterator indexIterator_)
{
super(indexIterator_);
}
@Override
public double nextDouble()
{
moveToNext();
return getCurrentDouble();
}
private double getCurrentDouble()
{
return DoubleBlockedArrayData.this.getDouble(_physIndex[0], _physIndex[1]);
}
@Override
public void set(double val_)
{
DoubleBlockedArrayData.this.set(_physIndex[0],_physIndex[1],val_);
}
}
private class BlockedDoubleDataSubspaceSplitIterator extends BlockedDataSubSpaceSplitIterator implements DoubleSubspaceSplitIterator
{
BlockedDoubleDataSubspaceSplitIterator(SubIndexIterator logicalIterator_)
{
super(logicalIterator_);
}
@Override
public double nextDouble()
{
if(!moveToNext()) return 0d;
return getCurrentDouble();
}
private double getCurrentDouble()
{
return DoubleBlockedArrayData.this.getDouble(_physIndex[0], _physIndex[1]);
}
@Override
public void set(double val_)
{
DoubleBlockedArrayData.this.set(_physIndex[0],_physIndex[1],val_);
}
@Override
public DoubleSubspaceSplitIterator getOrthogonalIterator()
{
// Create an orthogonal iterator
return new BlockedDoubleDataSubspaceSplitIterator(((SubSpaceSplitIterator)_indexIterator).getOrthogonalIterator());
}
}
public double[] toArray()
{
double[] data = new double[_logicalIndex.numberOfElements()];
int blockStart = 0;
for(int block = 0; block < _numBlocks; block++)
{
int currentBlockSize = getBlockSize(block);
System.arraycopy(_data[block], 0, data, blockStart, currentBlockSize);
blockStart += currentBlockSize;
}
return data;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy