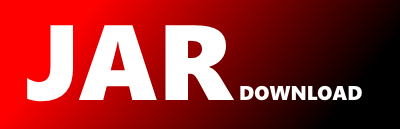
com.meliorbis.utils.Utils Maven / Gradle / Ivy
package com.meliorbis.utils;
import java.util.Arrays;
import java.util.List;
import java.util.Random;
import com.meliorbis.numerics.generic.MultiDimensionalArray;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.generic.primitives.DoubleSettableIndexedIterator;
public abstract class Utils {
// prevent construction
private Utils(){}
/**
* Returns a pair containing the maximum value (left) and the index at which it occurs of the
* given int array
*
* @param integers_ An array of integers. Must not be null or of 0 length
*
* @return A Pair with the maximum value and the index it occurs at in the left and right position respectively
*/
public static Pair max(int[] integers_)
{
int maxVal = integers_[0];
int maxIndex = 0;
for(int index = 1;index < integers_.length; index++)
{
if(integers_[index] > maxVal)
{
maxIndex = index;
maxVal = integers_[index];
}
}
return new Pair(maxVal,maxIndex);
}
public static int[] repeatArray(int i_, int length_) {
int[] result = new int[length_];
Arrays.fill(result, i_);
return result;
}
public static double[] repeatArray(double i_, int length_) {
double[] result = new double[length_];
Arrays.fill(result, i_);
return result;
}
/**
* Returns a sequence from start to stop (both inclusive) in count steps
*
* @param start_ The starting value
* @param stop_ The end value
* @param count_ The nunber of values to return
*
* @return The values in the sequence
*/
public static double[] sequence(double start_, double stop_, int count_) {
double[] sequence = new double[count_];
double step = (stop_-start_)/(count_-1);
sequence[0] = start_;
for(int i = 1; i < count_; i++)
{
sequence[i] = sequence[i-1]+step;
}
return sequence;
}
/**
* Returns a sequence from start (inclusive) so stop (exclusive)
*
* @param start_ The starting value
* @param stop_ The end value. Must be greater than or equal to start.
*
* @return The values in the sequence
*/
public static int[] sequence(int start_, int stop_) {
int range = stop_-start_;
// For reverse sequences, it could negative; figure out the sign
int step = (range) > 0 ? 1 : -1;
int[] sequence = new int[step*range];
for(int current = 0; current != range; current += step)
{
// Also need to multiply by step here to be sure of a positive index
sequence[step*current] = start_+current;
}
return sequence;
}
static public int[] toIntArray(List dimensions_) {
int[] dims = new int[dimensions_.size()];
for(int index = 0;index < dims.length; index++)
{
dims[index] = dimensions_.get(index);
}
return dims;
}
/**
* Given a 1-D array that represents a probability distribution over discrete states,
* draw a random value
*
* @param stateProbs_ The probability of each state occurring
* @param random_ The random number generator to use
*
* @return The drawn state
*/
static public int[] drawRandomState(DoubleArray> stateProbs_, Random random_)
{
double draw = random_.nextDouble();
DoubleSettableIndexedIterator probIter = stateProbs_.iterator();
while (probIter.hasNext() && (draw -= probIter.nextDouble()) > 0);
return probIter.getIndex();
}
/**
* Combines the provided arrays into one
*
* @param sequences_ The arrays to combine
*
* @return The combined array
*/
public static int[] combine(int[]... sequences_)
{
/* First we need to determine the length, which is the sum of the individual lengths
*/
int length = 0;
for (int i = 0; i < sequences_.length; i++)
{
length += sequences_[i].length;
}
/* Createe the array to hold the results
*/
int[] result = new int[length];
int startPosition = 0;
for (int i = 0; i < sequences_.length; i++)
{
System.arraycopy(sequences_[i], 0, result, startPosition, sequences_[i].length);
startPosition += sequences_[i].length;
}
return result;
}
/**
* Extracts the values from array at the indices indicated, returning the resulting array
*
* @param array_ The array to extract values from
* @param indices_ The indices of the values to extract
*
* @return The extracted values
*/
public static int[] extract(int[] array_, int[] indices_)
{
int[] results = new int[indices_.length];
/* Iterate over the desired indices, extracting the indicated index
* for each and placing it in results
*/
for (int indexIndex = 0; indexIndex < indices_.length; indexIndex++)
{
results[indexIndex] = array_[indices_[indexIndex]];
}
return results;
}
/**
* Adds the length, taken to be the size of the first dimension, of each of the arrays in the second argument to the
* list in the first argument
*
* @param dimensions List to add sizes to
* @param dimensionList Arrays of which the length (first dimension) is to be added to the list
*/
public static void addLengthsToList(List dimensions,
List extends MultiDimensionalArray,?>> dimensionList) {
for(MultiDimensionalArray,?> array : dimensionList)
{
dimensions.add(array.size()[0]);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy