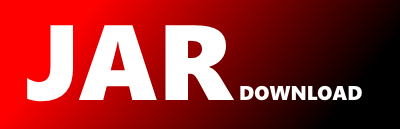
org.apache.commons.configuration2.plist.PropertyListParser Maven / Gradle / Ivy
/* PropertyListParser.java */
/* Generated By:JavaCC: Do not edit this line. PropertyListParser.java */
package org.apache.commons.configuration2.plist;
import java.util.Date;
import java.util.List;
import java.util.ArrayList;
import org.apache.commons.configuration2.HierarchicalConfiguration;
import org.apache.commons.configuration2.tree.ImmutableNode;
import org.apache.commons.codec.binary.Hex;
/**
* JavaCC based parser for the PropertyList format.
*
* @author Emmanuel Bourg
*/
class PropertyListParser implements PropertyListParserConstants {
/**
* Remove the quotes at the beginning and at the end of the specified String.
*/
protected String removeQuotes(String s)
{
if (s == null)
{
return null;
}
if (s.startsWith("\"") && s.endsWith("\"") && s.length() >= 2)
{
s = s.substring(1, s.length() - 1);
}
return s;
}
protected String unescapeQuotes(String s)
{
return s.replaceAll("\\\\\"", "\"");
}
/**
* Remove the white spaces and the data delimiters from the specified
* string and parse it as a byte array.
*/
protected byte[] filterData(String s) throws ParseException
{
if (s == null)
{
return null;
}
// remove the delimiters
if (s.startsWith("<") && s.endsWith(">") && s.length() >= 2)
{
s = s.substring(1, s.length() - 1);
}
// remove the white spaces
s = s.replaceAll("\\s", "");
// add a leading 0 to ensure well formed bytes
if (s.length() % 2 != 0)
{
s = "0" + s;
}
// parse and return the bytes
try
{
return Hex.decodeHex(s.toCharArray());
}
catch (Exception e)
{
throw (ParseException) new ParseException("Unable to parse the byte[] : " + e.getMessage());
}
}
/**
* Parse a date formatted as <*D2002-03-22 11:30:00 +0100>
*/
protected Date parseDate(String s) throws ParseException
{
return PropertyListConfiguration.parseDate(s);
}
final public PropertyListConfiguration parse() throws ParseException {PropertyListConfiguration configuration = null;
configuration = Dictionary();
jj_consume_token(0);
{if ("" != null) return configuration;}
throw new Error("Missing return statement in function");
}
final public PropertyListConfiguration Dictionary() throws ParseException {ImmutableNode.Builder builder = new ImmutableNode.Builder();
ImmutableNode child = null;
jj_consume_token(DICT_BEGIN);
label_1:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case STRING:
case QUOTED_STRING:{
;
break;
}
default:
jj_la1[0] = jj_gen;
break label_1;
}
child = Property();
if (child.getValue() instanceof HierarchicalConfiguration)
{
// prune & graft the nested configuration to the parent configuration
@SuppressWarnings("unchecked") // we created this configuration
HierarchicalConfiguration conf =
(HierarchicalConfiguration) child.getValue();
ImmutableNode root = conf.getNodeModel().getNodeHandler().getRootNode();
ImmutableNode.Builder childBuilder = new ImmutableNode.Builder();
childBuilder.name(child.getNodeName()).value(root.getValue())
.addChildren(root.getChildren());
builder.addChild(childBuilder.create());
}
else
{
builder.addChild(child);
}
}
jj_consume_token(DICT_END);
{if ("" != null) return new PropertyListConfiguration(builder.create());}
throw new Error("Missing return statement in function");
}
final public ImmutableNode Property() throws ParseException {String key = null;
Object value = null;
ImmutableNode.Builder node = new ImmutableNode.Builder();
key = String();
node.name(key);
jj_consume_token(EQUAL);
value = Element();
node.value(value);
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case DICT_SEPARATOR:{
jj_consume_token(DICT_SEPARATOR);
break;
}
default:
jj_la1[1] = jj_gen;
;
}
{if ("" != null) return node.create();}
throw new Error("Missing return statement in function");
}
final public Object Element() throws ParseException {Object value = null;
if (jj_2_1(2)) {
value = Array();
{if ("" != null) return value;}
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case DICT_BEGIN:{
value = Dictionary();
{if ("" != null) return value;}
break;
}
case STRING:
case QUOTED_STRING:{
value = String();
{if ("" != null) return value;}
break;
}
case DATA:{
value = Data();
{if ("" != null) return value;}
break;
}
case DATE:{
value = Date();
{if ("" != null) return value;}
break;
}
default:
jj_la1[2] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
throw new Error("Missing return statement in function");
}
final public List Array() throws ParseException {List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy