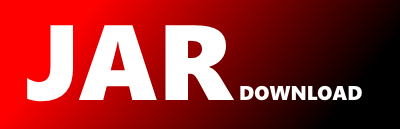
com.mengweifeng.springsupport.PropertyConfig Maven / Gradle / Ivy
package com.mengweifeng.springsupport;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashSet;
import java.util.List;
import java.util.Properties;
import java.util.Set;
import org.springframework.beans.factory.config.PropertyPlaceholderConfigurer;
import org.springframework.stereotype.Component;
@Component
public class PropertyConfig extends PropertyPlaceholderConfigurer {
private Properties properties;
private Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy