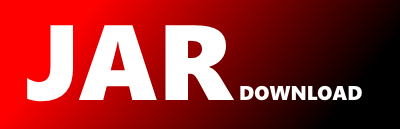
com.mercateo.eventstore.data.ImmutableEventInitiatorData Maven / Gradle / Ivy
Show all versions of client-common Show documentation
package com.mercateo.eventstore.data;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.mercateo.eventstore.domain.Reference;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.UUID;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link EventInitiatorData}.
*
* Use the builder to create immutable instances:
* {@code ImmutableEventInitiatorData.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "EventInitiatorData"})
@Immutable
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutableEventInitiatorData implements EventInitiatorData {
private final @Nullable UUID id;
private final @Nullable String type;
private final @Nullable ReferenceData initiator;
private final List agent;
private ImmutableEventInitiatorData(
@Nullable UUID id,
@Nullable String type,
@Nullable ReferenceData initiator,
List agent) {
this.id = id;
this.type = type;
this.initiator = initiator;
this.agent = agent;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public @Nullable UUID id() {
return id;
}
/**
* @return The value of the {@code type} attribute
*/
@JsonProperty("type")
@Override
public @Nullable String type() {
return type;
}
/**
* @return The value of the {@code initiator} attribute
*/
@JsonProperty("initiator")
@Override
public Optional initiator() {
return Optional.ofNullable(initiator);
}
/**
* @return The value of the {@code agent} attribute
*/
@JsonProperty("agent")
@Override
public List agent() {
return agent;
}
/**
* Copy the current immutable object by setting a value for the {@link EventInitiatorData#id() id} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableEventInitiatorData withId(@Nullable UUID value) {
if (this.id == value) return this;
return new ImmutableEventInitiatorData(value, this.type, this.initiator, this.agent);
}
/**
* Copy the current immutable object by setting a value for the {@link EventInitiatorData#type() type} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableEventInitiatorData withType(@Nullable String value) {
if (Objects.equals(this.type, value)) return this;
return new ImmutableEventInitiatorData(this.id, value, this.initiator, this.agent);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link EventInitiatorData#initiator() initiator} attribute.
* @param value The value for initiator
* @return A modified copy of {@code this} object
*/
public final ImmutableEventInitiatorData withInitiator(ReferenceData value) {
@Nullable ReferenceData newValue = Objects.requireNonNull(value, "initiator");
if (this.initiator == newValue) return this;
return new ImmutableEventInitiatorData(this.id, this.type, newValue, this.agent);
}
/**
* Copy the current immutable object by setting an optional value for the {@link EventInitiatorData#initiator() initiator} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for initiator
* @return A modified copy of {@code this} object
*/
public final ImmutableEventInitiatorData withInitiator(Optional extends ReferenceData> optional) {
@Nullable ReferenceData value = optional.orElse(null);
if (this.initiator == value) return this;
return new ImmutableEventInitiatorData(this.id, this.type, value, this.agent);
}
/**
* Copy the current immutable object with elements that replace the content of {@link EventInitiatorData#agent() agent}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableEventInitiatorData withAgent(ReferenceData... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableEventInitiatorData(this.id, this.type, this.initiator, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link EventInitiatorData#agent() agent}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of agent elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableEventInitiatorData withAgent(Iterable extends ReferenceData> elements) {
if (this.agent == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableEventInitiatorData(this.id, this.type, this.initiator, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableEventInitiatorData} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableEventInitiatorData
&& equalTo((ImmutableEventInitiatorData) another);
}
private boolean equalTo(ImmutableEventInitiatorData another) {
return Objects.equals(id, another.id)
&& Objects.equals(type, another.type)
&& Objects.equals(initiator, another.initiator)
&& agent.equals(another.agent);
}
/**
* Computes a hash code from attributes: {@code id}, {@code type}, {@code initiator}, {@code agent}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + Objects.hashCode(type);
h += (h << 5) + Objects.hashCode(initiator);
h += (h << 5) + agent.hashCode();
return h;
}
/**
* Prints the immutable value {@code EventInitiatorData} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("EventInitiatorData{");
if (id != null) {
builder.append("id=").append(id);
}
if (type != null) {
if (builder.length() > 19) builder.append(", ");
builder.append("type=").append(type);
}
if (initiator != null) {
if (builder.length() > 19) builder.append(", ");
builder.append("initiator=").append(initiator);
}
if (builder.length() > 19) builder.append(", ");
builder.append("agent=").append(agent);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements EventInitiatorData {
@Nullable UUID id;
@Nullable String type;
@Nullable Optional initiator = Optional.empty();
@Nullable List agent = Collections.emptyList();
@JsonProperty("id")
public void setId(@Nullable UUID id) {
this.id = id;
}
@JsonProperty("type")
public void setType(@Nullable String type) {
this.type = type;
}
@JsonProperty("initiator")
public void setInitiator(Optional initiator) {
this.initiator = initiator;
}
@JsonProperty("agent")
public void setAgent(List agent) {
this.agent = agent;
}
@Override
public UUID id() { throw new UnsupportedOperationException(); }
@Override
public String type() { throw new UnsupportedOperationException(); }
@Override
public Optional initiator() { throw new UnsupportedOperationException(); }
@Override
public List agent() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableEventInitiatorData fromJson(Json json) {
ImmutableEventInitiatorData.Builder builder = ImmutableEventInitiatorData.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.type != null) {
builder.type(json.type);
}
if (json.initiator != null) {
builder.initiator(json.initiator);
}
if (json.agent != null) {
builder.addAllAgent(json.agent);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link EventInitiatorData} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable EventInitiatorData instance
*/
public static ImmutableEventInitiatorData copyOf(EventInitiatorData instance) {
if (instance instanceof ImmutableEventInitiatorData) {
return (ImmutableEventInitiatorData) instance;
}
return ImmutableEventInitiatorData.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableEventInitiatorData ImmutableEventInitiatorData}.
* @return A new ImmutableEventInitiatorData builder
*/
public static ImmutableEventInitiatorData.Builder builder() {
return new ImmutableEventInitiatorData.Builder();
}
/**
* Builds instances of type {@link ImmutableEventInitiatorData ImmutableEventInitiatorData}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private @Nullable UUID id;
private @Nullable String type;
private @Nullable ReferenceData initiator;
private List agent = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.mercateo.eventstore.domain.Reference} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Reference instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.mercateo.eventstore.data.EventInitiatorData} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(EventInitiatorData instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
long bits = 0;
if (object instanceof Reference) {
Reference instance = (Reference) object;
if ((bits & 0x2L) == 0) {
@Nullable String typeValue = instance.type();
if (typeValue != null) {
type(typeValue);
}
bits |= 0x2L;
}
if ((bits & 0x1L) == 0) {
@Nullable UUID idValue = instance.id();
if (idValue != null) {
id(idValue);
}
bits |= 0x1L;
}
}
if (object instanceof EventInitiatorData) {
EventInitiatorData instance = (EventInitiatorData) object;
addAllAgent(instance.agent());
if ((bits & 0x1L) == 0) {
@Nullable UUID idValue = instance.id();
if (idValue != null) {
id(idValue);
}
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
@Nullable String typeValue = instance.type();
if (typeValue != null) {
type(typeValue);
}
bits |= 0x2L;
}
Optional initiatorOptional = instance.initiator();
if (initiatorOptional.isPresent()) {
initiator(initiatorOptional);
}
}
}
/**
* Initializes the value for the {@link EventInitiatorData#id() id} attribute.
* @param id The value for id (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("id")
public final Builder id(@Nullable UUID id) {
this.id = id;
return this;
}
/**
* Initializes the value for the {@link EventInitiatorData#type() type} attribute.
* @param type The value for type (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("type")
public final Builder type(@Nullable String type) {
this.type = type;
return this;
}
/**
* Initializes the optional value {@link EventInitiatorData#initiator() initiator} to initiator.
* @param initiator The value for initiator
* @return {@code this} builder for chained invocation
*/
public final Builder initiator(ReferenceData initiator) {
this.initiator = Objects.requireNonNull(initiator, "initiator");
return this;
}
/**
* Initializes the optional value {@link EventInitiatorData#initiator() initiator} to initiator.
* @param initiator The value for initiator
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("initiator")
public final Builder initiator(Optional extends ReferenceData> initiator) {
this.initiator = initiator.orElse(null);
return this;
}
/**
* Adds one element to {@link EventInitiatorData#agent() agent} list.
* @param element A agent element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAgent(ReferenceData element) {
this.agent.add(Objects.requireNonNull(element, "agent element"));
return this;
}
/**
* Adds elements to {@link EventInitiatorData#agent() agent} list.
* @param elements An array of agent elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAgent(ReferenceData... elements) {
for (ReferenceData element : elements) {
this.agent.add(Objects.requireNonNull(element, "agent element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link EventInitiatorData#agent() agent} list.
* @param elements An iterable of agent elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("agent")
public final Builder agent(Iterable extends ReferenceData> elements) {
this.agent.clear();
return addAllAgent(elements);
}
/**
* Adds elements to {@link EventInitiatorData#agent() agent} list.
* @param elements An iterable of agent elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllAgent(Iterable extends ReferenceData> elements) {
for (ReferenceData element : elements) {
this.agent.add(Objects.requireNonNull(element, "agent element"));
}
return this;
}
/**
* Builds a new {@link ImmutableEventInitiatorData ImmutableEventInitiatorData}.
* @return An immutable instance of EventInitiatorData
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableEventInitiatorData build() {
return new ImmutableEventInitiatorData(id, type, initiator, createUnmodifiableList(true, agent));
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList();
} else {
list = new ArrayList();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}