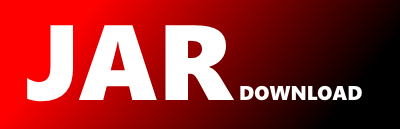
com.mercateo.eventstore.data.ImmutableSerializableMetadata Maven / Gradle / Ivy
Show all versions of client-common Show documentation
package com.mercateo.eventstore.data;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.UUID;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link SerializableMetadata}.
*
* Use the builder to create immutable instances:
* {@code ImmutableSerializableMetadata.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "SerializableMetadata"})
@Immutable
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutableSerializableMetadata implements SerializableMetadata {
private final UUID eventId;
private final @Nullable UUID correlationId;
private final @Nullable JsonNode schema;
private final @Nullable String schemaRef;
private final @Nullable Integer version;
private final @Nullable String eventType;
private final @Nullable CausalityData[] causality;
private final @Nullable EventInitiatorData eventInitiator;
private ImmutableSerializableMetadata(
UUID eventId,
@Nullable UUID correlationId,
@Nullable JsonNode schema,
@Nullable String schemaRef,
@Nullable Integer version,
@Nullable String eventType,
@Nullable CausalityData[] causality,
@Nullable EventInitiatorData eventInitiator) {
this.eventId = eventId;
this.correlationId = correlationId;
this.schema = schema;
this.schemaRef = schemaRef;
this.version = version;
this.eventType = eventType;
this.causality = causality;
this.eventInitiator = eventInitiator;
}
/**
* @return The value of the {@code eventId} attribute
*/
@JsonProperty("eventId")
@Override
public UUID eventId() {
return eventId;
}
/**
* @return The value of the {@code correlationId} attribute
*/
@JsonProperty("correlationId")
@Override
public @Nullable UUID correlationId() {
return correlationId;
}
/**
* @return The value of the {@code schema} attribute
*/
@JsonProperty("schema")
@Override
public @Nullable JsonNode schema() {
return schema;
}
/**
* @return The value of the {@code schemaRef} attribute
*/
@JsonProperty("schemaRef")
@Override
public @Nullable String schemaRef() {
return schemaRef;
}
/**
* @return The value of the {@code version} attribute
*/
@JsonProperty("version")
@Override
public @Nullable Integer version() {
return version;
}
/**
* @return The value of the {@code eventType} attribute
*/
@JsonProperty("eventType")
@Override
public @Nullable String eventType() {
return eventType;
}
/**
* @return A cloned {@code causality} array
*/
@JsonProperty("causality")
@Override
public @Nullable CausalityData[] causality() {
return causality;
}
/**
* @return The value of the {@code eventInitiator} attribute
*/
@JsonProperty("eventInitiator")
@Override
public @Nullable EventInitiatorData eventInitiator() {
return eventInitiator;
}
/**
* Copy the current immutable object by setting a value for the {@link SerializableMetadata#eventId() eventId} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventId
* @return A modified copy of the {@code this} object
*/
public final ImmutableSerializableMetadata withEventId(UUID value) {
if (this.eventId == value) return this;
UUID newValue = Objects.requireNonNull(value, "eventId");
return new ImmutableSerializableMetadata(
newValue,
this.correlationId,
this.schema,
this.schemaRef,
this.version,
this.eventType,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link SerializableMetadata#correlationId() correlationId} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for correlationId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableSerializableMetadata withCorrelationId(@Nullable UUID value) {
if (this.correlationId == value) return this;
return new ImmutableSerializableMetadata(
this.eventId,
value,
this.schema,
this.schemaRef,
this.version,
this.eventType,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link SerializableMetadata#schema() schema} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for schema (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableSerializableMetadata withSchema(@Nullable JsonNode value) {
if (this.schema == value) return this;
return new ImmutableSerializableMetadata(
this.eventId,
this.correlationId,
value,
this.schemaRef,
this.version,
this.eventType,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link SerializableMetadata#schemaRef() schemaRef} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for schemaRef (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableSerializableMetadata withSchemaRef(@Nullable String value) {
if (Objects.equals(this.schemaRef, value)) return this;
return new ImmutableSerializableMetadata(
this.eventId,
this.correlationId,
this.schema,
value,
this.version,
this.eventType,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link SerializableMetadata#version() version} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for version (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableSerializableMetadata withVersion(@Nullable Integer value) {
if (Objects.equals(this.version, value)) return this;
return new ImmutableSerializableMetadata(
this.eventId,
this.correlationId,
this.schema,
this.schemaRef,
value,
this.eventType,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link SerializableMetadata#eventType() eventType} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventType (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableSerializableMetadata withEventType(@Nullable String value) {
if (Objects.equals(this.eventType, value)) return this;
return new ImmutableSerializableMetadata(
this.eventId,
this.correlationId,
this.schema,
this.schemaRef,
this.version,
value,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SerializableMetadata#causality() causality}.
* The array is cloned before being saved as attribute values.
* @param elements The non-null elements for causality
* @return A modified copy of {@code this} object
*/
public final ImmutableSerializableMetadata withCausality(@Nullable CausalityData... elements) {
@Nullable CausalityData[] newValue = elements == null ? null : elements.clone();
return new ImmutableSerializableMetadata(
this.eventId,
this.correlationId,
this.schema,
this.schemaRef,
this.version,
this.eventType,
newValue,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link SerializableMetadata#eventInitiator() eventInitiator} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventInitiator (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableSerializableMetadata withEventInitiator(@Nullable EventInitiatorData value) {
if (this.eventInitiator == value) return this;
return new ImmutableSerializableMetadata(
this.eventId,
this.correlationId,
this.schema,
this.schemaRef,
this.version,
this.eventType,
this.causality,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableSerializableMetadata} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableSerializableMetadata
&& equalTo((ImmutableSerializableMetadata) another);
}
private boolean equalTo(ImmutableSerializableMetadata another) {
return eventId.equals(another.eventId)
&& Objects.equals(correlationId, another.correlationId)
&& Objects.equals(schema, another.schema)
&& Objects.equals(schemaRef, another.schemaRef)
&& Objects.equals(version, another.version)
&& Objects.equals(eventType, another.eventType)
&& Arrays.equals(causality, another.causality)
&& Objects.equals(eventInitiator, another.eventInitiator);
}
/**
* Computes a hash code from attributes: {@code eventId}, {@code correlationId}, {@code schema}, {@code schemaRef}, {@code version}, {@code eventType}, {@code causality}, {@code eventInitiator}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + eventId.hashCode();
h += (h << 5) + Objects.hashCode(correlationId);
h += (h << 5) + Objects.hashCode(schema);
h += (h << 5) + Objects.hashCode(schemaRef);
h += (h << 5) + Objects.hashCode(version);
h += (h << 5) + Objects.hashCode(eventType);
h += (h << 5) + Arrays.hashCode(causality);
h += (h << 5) + Objects.hashCode(eventInitiator);
return h;
}
/**
* Prints the immutable value {@code SerializableMetadata} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "SerializableMetadata{"
+ "eventId=" + eventId
+ ", correlationId=" + correlationId
+ ", schema=" + schema
+ ", schemaRef=" + schemaRef
+ ", version=" + version
+ ", eventType=" + eventType
+ ", causality=" + Arrays.toString(causality)
+ ", eventInitiator=" + eventInitiator
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SerializableMetadata {
@Nullable UUID eventId;
@Nullable UUID correlationId;
@Nullable JsonNode schema;
@Nullable String schemaRef;
@Nullable Integer version;
@Nullable String eventType;
@Nullable CausalityData[] causality;
@Nullable EventInitiatorData eventInitiator;
@JsonProperty("eventId")
public void setEventId(UUID eventId) {
this.eventId = eventId;
}
@JsonProperty("correlationId")
public void setCorrelationId(@Nullable UUID correlationId) {
this.correlationId = correlationId;
}
@JsonProperty("schema")
public void setSchema(@Nullable JsonNode schema) {
this.schema = schema;
}
@JsonProperty("schemaRef")
public void setSchemaRef(@Nullable String schemaRef) {
this.schemaRef = schemaRef;
}
@JsonProperty("version")
public void setVersion(@Nullable Integer version) {
this.version = version;
}
@JsonProperty("eventType")
public void setEventType(@Nullable String eventType) {
this.eventType = eventType;
}
@JsonProperty("causality")
public void setCausality(@Nullable CausalityData[] causality) {
this.causality = causality;
}
@JsonProperty("eventInitiator")
public void setEventInitiator(@Nullable EventInitiatorData eventInitiator) {
this.eventInitiator = eventInitiator;
}
@Override
public UUID eventId() { throw new UnsupportedOperationException(); }
@Override
public UUID correlationId() { throw new UnsupportedOperationException(); }
@Override
public JsonNode schema() { throw new UnsupportedOperationException(); }
@Override
public String schemaRef() { throw new UnsupportedOperationException(); }
@Override
public Integer version() { throw new UnsupportedOperationException(); }
@Override
public String eventType() { throw new UnsupportedOperationException(); }
@Override
public CausalityData[] causality() { throw new UnsupportedOperationException(); }
@Override
public EventInitiatorData eventInitiator() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableSerializableMetadata fromJson(Json json) {
ImmutableSerializableMetadata.Builder builder = ImmutableSerializableMetadata.builder();
if (json.eventId != null) {
builder.eventId(json.eventId);
}
if (json.correlationId != null) {
builder.correlationId(json.correlationId);
}
if (json.schema != null) {
builder.schema(json.schema);
}
if (json.schemaRef != null) {
builder.schemaRef(json.schemaRef);
}
if (json.version != null) {
builder.version(json.version);
}
if (json.eventType != null) {
builder.eventType(json.eventType);
}
if (json.causality != null) {
builder.causality(json.causality);
}
if (json.eventInitiator != null) {
builder.eventInitiator(json.eventInitiator);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link SerializableMetadata} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SerializableMetadata instance
*/
public static ImmutableSerializableMetadata copyOf(SerializableMetadata instance) {
if (instance instanceof ImmutableSerializableMetadata) {
return (ImmutableSerializableMetadata) instance;
}
return ImmutableSerializableMetadata.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableSerializableMetadata ImmutableSerializableMetadata}.
* @return A new ImmutableSerializableMetadata builder
*/
public static ImmutableSerializableMetadata.Builder builder() {
return new ImmutableSerializableMetadata.Builder();
}
/**
* Builds instances of type {@link ImmutableSerializableMetadata ImmutableSerializableMetadata}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_EVENT_ID = 0x1L;
private long initBits = 0x1L;
private @Nullable UUID eventId;
private @Nullable UUID correlationId;
private @Nullable JsonNode schema;
private @Nullable String schemaRef;
private @Nullable Integer version;
private @Nullable String eventType;
private @Nullable CausalityData[] causality;
private @Nullable EventInitiatorData eventInitiator;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code SerializableMetadata} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SerializableMetadata instance) {
Objects.requireNonNull(instance, "instance");
eventId(instance.eventId());
@Nullable UUID correlationIdValue = instance.correlationId();
if (correlationIdValue != null) {
correlationId(correlationIdValue);
}
@Nullable JsonNode schemaValue = instance.schema();
if (schemaValue != null) {
schema(schemaValue);
}
@Nullable String schemaRefValue = instance.schemaRef();
if (schemaRefValue != null) {
schemaRef(schemaRefValue);
}
@Nullable Integer versionValue = instance.version();
if (versionValue != null) {
version(versionValue);
}
@Nullable String eventTypeValue = instance.eventType();
if (eventTypeValue != null) {
eventType(eventTypeValue);
}
@Nullable CausalityData[] causalityValue = instance.causality();
if (causalityValue != null) {
causality(causalityValue);
}
@Nullable EventInitiatorData eventInitiatorValue = instance.eventInitiator();
if (eventInitiatorValue != null) {
eventInitiator(eventInitiatorValue);
}
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#eventId() eventId} attribute.
* @param eventId The value for eventId
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("eventId")
public final Builder eventId(UUID eventId) {
this.eventId = Objects.requireNonNull(eventId, "eventId");
initBits &= ~INIT_BIT_EVENT_ID;
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#correlationId() correlationId} attribute.
* @param correlationId The value for correlationId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("correlationId")
public final Builder correlationId(@Nullable UUID correlationId) {
this.correlationId = correlationId;
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#schema() schema} attribute.
* @param schema The value for schema (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("schema")
public final Builder schema(@Nullable JsonNode schema) {
this.schema = schema;
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#schemaRef() schemaRef} attribute.
* @param schemaRef The value for schemaRef (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("schemaRef")
public final Builder schemaRef(@Nullable String schemaRef) {
this.schemaRef = schemaRef;
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#version() version} attribute.
* @param version The value for version (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("version")
public final Builder version(@Nullable Integer version) {
this.version = version;
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#eventType() eventType} attribute.
* @param eventType The value for eventType (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("eventType")
public final Builder eventType(@Nullable String eventType) {
this.eventType = eventType;
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#causality() causality} attribute.
* @param causality The elements for causality
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("causality")
public final Builder causality(CausalityData... causality) {
this.causality = causality;
return this;
}
/**
* Initializes the value for the {@link SerializableMetadata#eventInitiator() eventInitiator} attribute.
* @param eventInitiator The value for eventInitiator (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("eventInitiator")
public final Builder eventInitiator(@Nullable EventInitiatorData eventInitiator) {
this.eventInitiator = eventInitiator;
return this;
}
/**
* Builds a new {@link ImmutableSerializableMetadata ImmutableSerializableMetadata}.
* @return An immutable instance of SerializableMetadata
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableSerializableMetadata build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableSerializableMetadata(eventId, correlationId, schema, schemaRef, version, eventType, causality, eventInitiator);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList();
if ((initBits & INIT_BIT_EVENT_ID) != 0) attributes.add("eventId");
return "Cannot build SerializableMetadata, some of required attributes are not set " + attributes;
}
}
}