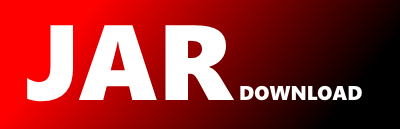
com.mercateo.eventstore.domain.EventSchemaRef Maven / Gradle / Ivy
Show all versions of client-common Show documentation
package com.mercateo.eventstore.domain;
import java.net.URI;
import java.util.Objects;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
/**
* Immutable implementation of {@link _EventSchemaRef}.
*
* Use the static factory method to create immutable instances:
* {@code EventSchemaRef.of()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "_EventSchemaRef"})
@Immutable
public final class EventSchemaRef extends com.mercateo.eventstore.domain._EventSchemaRef {
private final URI value;
private EventSchemaRef(URI value) {
this.value = Objects.requireNonNull(value, "value");
}
/**
* @return The value of the {@code value} attribute
*/
@Override
public URI value() {
return value;
}
/**
* This instance is equal to all instances of {@code EventSchemaRef} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof EventSchemaRef
&& equalTo((EventSchemaRef) another);
}
private boolean equalTo(EventSchemaRef another) {
return value.equals(another.value);
}
/**
* Computes a hash code from attributes: {@code value}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + value.hashCode();
return h;
}
/**
* Construct a new immutable {@code EventSchemaRef} instance.
* @param value The value for the {@code value} attribute
* @return An immutable EventSchemaRef instance
*/
public static EventSchemaRef of(URI value) {
return new EventSchemaRef(value);
}
}