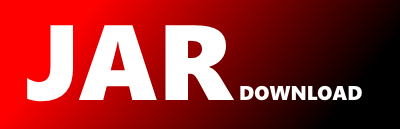
com.mercateo.eventstore.domain.ImmutableEventMetadata Maven / Gradle / Ivy
Show all versions of client-common Show documentation
package com.mercateo.eventstore.domain;
import io.vavr.collection.List;
import io.vavr.control.Option;
import java.util.ArrayList;
import java.util.Objects;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link EventMetadata}.
*
* Use the builder to create immutable instances:
* {@code ImmutableEventMetadata.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "EventMetadata"})
@Immutable
public final class ImmutableEventMetadata implements EventMetadata {
private final EventStreamId eventStreamId;
private final EventNumber eventNumber;
private final EventType eventType;
private final EventId eventId;
private final Option eventSchemaRef;
private final EventVersion version;
private final List causality;
private final Option eventInitiator;
private ImmutableEventMetadata(
EventStreamId eventStreamId,
EventNumber eventNumber,
EventType eventType,
EventId eventId,
Option eventSchemaRef,
EventVersion version,
List causality,
Option eventInitiator) {
this.eventStreamId = eventStreamId;
this.eventNumber = eventNumber;
this.eventType = eventType;
this.eventId = eventId;
this.eventSchemaRef = eventSchemaRef;
this.version = version;
this.causality = causality;
this.eventInitiator = eventInitiator;
}
/**
* @return The value of the {@code eventStreamId} attribute
*/
@Override
public EventStreamId eventStreamId() {
return eventStreamId;
}
/**
* @return The value of the {@code eventNumber} attribute
*/
@Override
public EventNumber eventNumber() {
return eventNumber;
}
/**
* @return The value of the {@code eventType} attribute
*/
@Override
public EventType eventType() {
return eventType;
}
/**
* @return The value of the {@code eventId} attribute
*/
@Override
public EventId eventId() {
return eventId;
}
/**
* @return The value of the {@code eventSchemaRef} attribute
*/
@Override
public Option eventSchemaRef() {
return eventSchemaRef;
}
/**
* @return The value of the {@code version} attribute
*/
@Override
public EventVersion version() {
return version;
}
/**
* @return The value of the {@code causality} attribute
*/
@Override
public List causality() {
return causality;
}
/**
* @return The value of the {@code eventInitiator} attribute
*/
@Override
public Option eventInitiator() {
return eventInitiator;
}
/**
* Copy the current immutable object by setting a value for the {@link EventMetadata#eventStreamId() eventStreamId} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventStreamId
* @return A modified copy of the {@code this} object
*/
public final ImmutableEventMetadata withEventStreamId(EventStreamId value) {
if (this.eventStreamId == value) return this;
EventStreamId newValue = Objects.requireNonNull(value, "eventStreamId");
return new ImmutableEventMetadata(
newValue,
this.eventNumber,
this.eventType,
this.eventId,
this.eventSchemaRef,
this.version,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link EventMetadata#eventNumber() eventNumber} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventNumber
* @return A modified copy of the {@code this} object
*/
public final ImmutableEventMetadata withEventNumber(EventNumber value) {
if (this.eventNumber == value) return this;
EventNumber newValue = Objects.requireNonNull(value, "eventNumber");
return new ImmutableEventMetadata(
this.eventStreamId,
newValue,
this.eventType,
this.eventId,
this.eventSchemaRef,
this.version,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link EventMetadata#eventType() eventType} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventType
* @return A modified copy of the {@code this} object
*/
public final ImmutableEventMetadata withEventType(EventType value) {
if (this.eventType == value) return this;
EventType newValue = Objects.requireNonNull(value, "eventType");
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
newValue,
this.eventId,
this.eventSchemaRef,
this.version,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link EventMetadata#eventId() eventId} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventId
* @return A modified copy of the {@code this} object
*/
public final ImmutableEventMetadata withEventId(EventId value) {
if (this.eventId == value) return this;
EventId newValue = Objects.requireNonNull(value, "eventId");
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
this.eventType,
newValue,
this.eventSchemaRef,
this.version,
this.causality,
this.eventInitiator);
}
public ImmutableEventMetadata withEventSchemaRef(Option value) {
Option newValue = Objects.requireNonNull(value);
if (this.eventSchemaRef == newValue) return this;
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
this.eventType,
this.eventId,
newValue,
this.version,
this.causality,
this.eventInitiator);
}
public ImmutableEventMetadata withEventSchemaRef(EventSchemaRef value) {
Option newValue = Option.some(value);
if (this.eventSchemaRef == newValue) return this;
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
this.eventType,
this.eventId,
newValue,
this.version,
this.causality,
this.eventInitiator);
}
/**
* Copy the current immutable object by setting a value for the {@link EventMetadata#version() version} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for version
* @return A modified copy of the {@code this} object
*/
public final ImmutableEventMetadata withVersion(EventVersion value) {
if (this.version == value) return this;
EventVersion newValue = Objects.requireNonNull(value, "version");
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
this.eventType,
this.eventId,
this.eventSchemaRef,
newValue,
this.causality,
this.eventInitiator);
}
public ImmutableEventMetadata withCausality(List value) {
List newValue = causality_from(value);
if (this.causality == newValue) return this;
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
this.eventType,
this.eventId,
this.eventSchemaRef,
this.version,
newValue,
this.eventInitiator);
}
public ImmutableEventMetadata withEventInitiator(Option value) {
Option newValue = Objects.requireNonNull(value);
if (this.eventInitiator == newValue) return this;
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
this.eventType,
this.eventId,
this.eventSchemaRef,
this.version,
this.causality,
newValue);
}
public ImmutableEventMetadata withEventInitiator(EventInitiator value) {
Option newValue = Option.some(value);
if (this.eventInitiator == newValue) return this;
return new ImmutableEventMetadata(
this.eventStreamId,
this.eventNumber,
this.eventType,
this.eventId,
this.eventSchemaRef,
this.version,
this.causality,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableEventMetadata} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableEventMetadata
&& equalTo((ImmutableEventMetadata) another);
}
private boolean equalTo(ImmutableEventMetadata another) {
return eventStreamId.equals(another.eventStreamId)
&& eventNumber.equals(another.eventNumber)
&& eventType.equals(another.eventType)
&& eventId.equals(another.eventId)
&& this.eventSchemaRef().equals(another.eventSchemaRef())
&& version.equals(another.version)
&& this.causality().equals(another.causality())
&& this.eventInitiator().equals(another.eventInitiator());
}
/**
* Computes a hash code from attributes: {@code eventStreamId}, {@code eventNumber}, {@code eventType}, {@code eventId}, {@code eventSchemaRef}, {@code version}, {@code causality}, {@code eventInitiator}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + eventStreamId.hashCode();
h += (h << 5) + eventNumber.hashCode();
h += (h << 5) + eventType.hashCode();
h += (h << 5) + eventId.hashCode();
h += (h << 5) + (eventSchemaRef().hashCode());
h += (h << 5) + version.hashCode();
h += (h << 5) + (causality().hashCode());
h += (h << 5) + (eventInitiator().hashCode());
return h;
}
/**
* Prints the immutable value {@code EventMetadata} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "EventMetadata{"
+ "eventStreamId=" + eventStreamId
+ ", eventNumber=" + eventNumber
+ ", eventType=" + eventType
+ ", eventId=" + eventId
+ ", eventSchemaRef=" + (eventSchemaRef().toString())
+ ", version=" + version
+ ", causality=" + (causality().toString())
+ ", eventInitiator=" + (eventInitiator().toString())
+ "}";
}
/**
* Creates an immutable copy of a {@link EventMetadata} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable EventMetadata instance
*/
public static ImmutableEventMetadata copyOf(EventMetadata instance) {
if (instance instanceof ImmutableEventMetadata) {
return (ImmutableEventMetadata) instance;
}
return ImmutableEventMetadata.builder()
.from(instance)
.build();
}
private static Option eventSchemaRef_from(Option value) {
return value;
}
private static List causality_from(List value) {
return value;
}
private static Option eventInitiator_from(Option value) {
return value;
}
/**
* Creates a builder for {@link ImmutableEventMetadata ImmutableEventMetadata}.
* @return A new ImmutableEventMetadata builder
*/
public static ImmutableEventMetadata.Builder builder() {
return new ImmutableEventMetadata.Builder();
}
/**
* Builds instances of type {@link ImmutableEventMetadata ImmutableEventMetadata}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_EVENT_STREAM_ID = 0x1L;
private static final long INIT_BIT_EVENT_NUMBER = 0x2L;
private static final long INIT_BIT_EVENT_TYPE = 0x4L;
private static final long INIT_BIT_EVENT_ID = 0x8L;
private static final long INIT_BIT_VERSION = 0x10L;
private long initBits = 0x1fL;
private Option eventSchemaRef_optional = Option.none();
private List causality_list = List.empty();
private Option eventInitiator_optional = Option.none();
private @Nullable EventStreamId eventStreamId;
private @Nullable EventNumber eventNumber;
private @Nullable EventType eventType;
private @Nullable EventId eventId;
private @Nullable EventVersion version;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code EventMetadata} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(EventMetadata instance) {
Objects.requireNonNull(instance, "instance");
eventStreamId(instance.eventStreamId());
eventNumber(instance.eventNumber());
eventType(instance.eventType());
eventId(instance.eventId());
eventSchemaRef(instance.eventSchemaRef());
version(instance.version());
causality(instance.causality());
eventInitiator(instance.eventInitiator());
return this;
}
/**
* Initializes the value for the {@link EventMetadata#eventStreamId() eventStreamId} attribute.
* @param eventStreamId The value for eventStreamId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder eventStreamId(EventStreamId eventStreamId) {
this.eventStreamId = Objects.requireNonNull(eventStreamId, "eventStreamId");
initBits &= ~INIT_BIT_EVENT_STREAM_ID;
return this;
}
/**
* Initializes the value for the {@link EventMetadata#eventNumber() eventNumber} attribute.
* @param eventNumber The value for eventNumber
* @return {@code this} builder for use in a chained invocation
*/
public final Builder eventNumber(EventNumber eventNumber) {
this.eventNumber = Objects.requireNonNull(eventNumber, "eventNumber");
initBits &= ~INIT_BIT_EVENT_NUMBER;
return this;
}
/**
* Initializes the value for the {@link EventMetadata#eventType() eventType} attribute.
* @param eventType The value for eventType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder eventType(EventType eventType) {
this.eventType = Objects.requireNonNull(eventType, "eventType");
initBits &= ~INIT_BIT_EVENT_TYPE;
return this;
}
/**
* Initializes the value for the {@link EventMetadata#eventId() eventId} attribute.
* @param eventId The value for eventId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder eventId(EventId eventId) {
this.eventId = Objects.requireNonNull(eventId, "eventId");
initBits &= ~INIT_BIT_EVENT_ID;
return this;
}
public Builder eventSchemaRef(Option opt) {
this.eventSchemaRef_optional = opt;
return this;
}
public Builder setValueEventSchemaRef(EventSchemaRef x) {
this.eventSchemaRef_optional = Option.of(x);
return this;
}
public Builder unsetEventSchemaRef() {
this.eventSchemaRef_optional = Option.none();
return this;
}
/**
* Initializes the value for the {@link EventMetadata#version() version} attribute.
* @param version The value for version
* @return {@code this} builder for use in a chained invocation
*/
public final Builder version(EventVersion version) {
this.version = Objects.requireNonNull(version, "version");
initBits &= ~INIT_BIT_VERSION;
return this;
}
public Builder addCausality(Causality element) {
this.causality_list = this.causality_list.append(element);
return this;
}
public Builder addAllCausality(Iterable element) {
this.causality_list = this.causality_list.appendAll(element);
return this;
}
public Builder causality(List elements) {
this.causality_list = elements;
return this;
}
public Builder setIterableCausality(Iterable elements) {
this.causality_list = List.ofAll(elements);
return this;
}
public Builder eventInitiator(Option opt) {
this.eventInitiator_optional = opt;
return this;
}
public Builder setValueEventInitiator(EventInitiator x) {
this.eventInitiator_optional = Option.of(x);
return this;
}
public Builder unsetEventInitiator() {
this.eventInitiator_optional = Option.none();
return this;
}
/**
* Builds a new {@link ImmutableEventMetadata ImmutableEventMetadata}.
* @return An immutable instance of EventMetadata
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableEventMetadata build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableEventMetadata(
eventStreamId,
eventNumber,
eventType,
eventId,
this.eventSchemaRef_optional,
version,
this.causality_list,
this.eventInitiator_optional);
}
private String formatRequiredAttributesMessage() {
java.util.List attributes = new ArrayList();
if ((initBits & INIT_BIT_EVENT_STREAM_ID) != 0) attributes.add("eventStreamId");
if ((initBits & INIT_BIT_EVENT_NUMBER) != 0) attributes.add("eventNumber");
if ((initBits & INIT_BIT_EVENT_TYPE) != 0) attributes.add("eventType");
if ((initBits & INIT_BIT_EVENT_ID) != 0) attributes.add("eventId");
if ((initBits & INIT_BIT_VERSION) != 0) attributes.add("version");
return "Cannot build EventMetadata, some of required attributes are not set " + attributes;
}
}
}