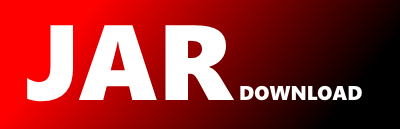
com.mercateo.spring.security.jwt.token.claim.ImmutableJWTClaim Maven / Gradle / Ivy
Show all versions of spring-security-jwt Show documentation
package com.mercateo.spring.security.jwt.token.claim;
import com.google.common.base.MoreObjects;
import com.google.common.collect.Lists;
import com.google.common.primitives.Booleans;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link JWTClaim}.
*
* Use the builder to create immutable instances:
* {@code ImmutableJWTClaim.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "JWTClaim"})
@Immutable
@CheckReturnValue
public final class ImmutableJWTClaim implements JWTClaim {
private final String name;
private final String value;
private final String issuer;
private final boolean verified;
private final @Nullable JWTClaim innerClaim;
private final int depth;
private ImmutableJWTClaim(ImmutableJWTClaim.Builder builder) {
this.name = builder.name;
this.value = builder.value;
this.innerClaim = builder.innerClaim;
if (builder.issuer != null) {
initShim.issuer(builder.issuer);
}
if (builder.verifiedIsSet()) {
initShim.verified(builder.verified);
}
if (builder.depthIsSet()) {
initShim.depth(builder.depth);
}
this.issuer = initShim.issuer();
this.verified = initShim.verified();
this.depth = initShim.depth();
this.initShim = null;
}
private ImmutableJWTClaim(
String name,
String value,
String issuer,
boolean verified,
@Nullable JWTClaim innerClaim,
int depth) {
this.name = name;
this.value = value;
this.issuer = issuer;
this.verified = verified;
this.innerClaim = innerClaim;
this.depth = depth;
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private String issuer;
private int issuerBuildStage;
String issuer() {
if (issuerBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (issuerBuildStage == STAGE_UNINITIALIZED) {
issuerBuildStage = STAGE_INITIALIZING;
this.issuer = Objects.requireNonNull(issuerInitialize(), "issuer");
issuerBuildStage = STAGE_INITIALIZED;
}
return this.issuer;
}
void issuer(String issuer) {
this.issuer = issuer;
issuerBuildStage = STAGE_INITIALIZED;
}
private boolean verified;
private int verifiedBuildStage;
boolean verified() {
if (verifiedBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (verifiedBuildStage == STAGE_UNINITIALIZED) {
verifiedBuildStage = STAGE_INITIALIZING;
this.verified = verifiedInitialize();
verifiedBuildStage = STAGE_INITIALIZED;
}
return this.verified;
}
void verified(boolean verified) {
this.verified = verified;
verifiedBuildStage = STAGE_INITIALIZED;
}
private int depth;
private int depthBuildStage;
int depth() {
if (depthBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (depthBuildStage == STAGE_UNINITIALIZED) {
depthBuildStage = STAGE_INITIALIZING;
this.depth = depthInitialize();
depthBuildStage = STAGE_INITIALIZED;
}
return this.depth;
}
void depth(int depth) {
this.depth = depth;
depthBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
ArrayList attributes = Lists.newArrayList();
if (issuerBuildStage == STAGE_INITIALIZING) attributes.add("issuer");
if (verifiedBuildStage == STAGE_INITIALIZING) attributes.add("verified");
if (depthBuildStage == STAGE_INITIALIZING) attributes.add("depth");
return "Cannot build JWTClaim, attribute initializers form cycle" + attributes;
}
}
private String issuerInitialize() {
return JWTClaim.super.issuer();
}
private boolean verifiedInitialize() {
return JWTClaim.super.verified();
}
private int depthInitialize() {
return JWTClaim.super.depth();
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String name() {
return name;
}
/**
* @return The value of the {@code value} attribute
*/
@Override
public String value() {
return value;
}
/**
* @return The value of the {@code issuer} attribute
*/
@Override
public String issuer() {
InitShim shim = this.initShim;
return shim != null
? shim.issuer()
: this.issuer;
}
/**
* @return The value of the {@code verified} attribute
*/
@Override
public boolean verified() {
InitShim shim = this.initShim;
return shim != null
? shim.verified()
: this.verified;
}
/**
* @return The value of the {@code innerClaim} attribute
*/
@Override
public Optional innerClaim() {
return Optional.ofNullable(innerClaim);
}
/**
* @return The value of the {@code depth} attribute
*/
@Override
public int depth() {
InitShim shim = this.initShim;
return shim != null
? shim.depth()
: this.depth;
}
/**
* Copy the current immutable object by setting a value for the {@link JWTClaim#name() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableJWTClaim withName(String value) {
if (this.name.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "name");
return new ImmutableJWTClaim(newValue, this.value, this.issuer, this.verified, this.innerClaim, this.depth);
}
/**
* Copy the current immutable object by setting a value for the {@link JWTClaim#value() value} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for value
* @return A modified copy of the {@code this} object
*/
public final ImmutableJWTClaim withValue(String value) {
if (this.value.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "value");
return new ImmutableJWTClaim(this.name, newValue, this.issuer, this.verified, this.innerClaim, this.depth);
}
/**
* Copy the current immutable object by setting a value for the {@link JWTClaim#issuer() issuer} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for issuer
* @return A modified copy of the {@code this} object
*/
public final ImmutableJWTClaim withIssuer(String value) {
if (this.issuer.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "issuer");
return new ImmutableJWTClaim(this.name, this.value, newValue, this.verified, this.innerClaim, this.depth);
}
/**
* Copy the current immutable object by setting a value for the {@link JWTClaim#verified() verified} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for verified
* @return A modified copy of the {@code this} object
*/
public final ImmutableJWTClaim withVerified(boolean value) {
if (this.verified == value) return this;
return new ImmutableJWTClaim(this.name, this.value, this.issuer, value, this.innerClaim, this.depth);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link JWTClaim#innerClaim() innerClaim} attribute.
* @param value The value for innerClaim
* @return A modified copy of {@code this} object
*/
public final ImmutableJWTClaim withInnerClaim(JWTClaim value) {
@Nullable JWTClaim newValue = Objects.requireNonNull(value, "innerClaim");
if (this.innerClaim == newValue) return this;
return new ImmutableJWTClaim(this.name, this.value, this.issuer, this.verified, newValue, this.depth);
}
/**
* Copy the current immutable object by setting an optional value for the {@link JWTClaim#innerClaim() innerClaim} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for innerClaim
* @return A modified copy of {@code this} object
*/
public final ImmutableJWTClaim withInnerClaim(Optional extends JWTClaim> optional) {
@Nullable JWTClaim value = optional.orElse(null);
if (this.innerClaim == value) return this;
return new ImmutableJWTClaim(this.name, this.value, this.issuer, this.verified, value, this.depth);
}
/**
* Copy the current immutable object by setting a value for the {@link JWTClaim#depth() depth} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for depth
* @return A modified copy of the {@code this} object
*/
public final ImmutableJWTClaim withDepth(int value) {
if (this.depth == value) return this;
return new ImmutableJWTClaim(this.name, this.value, this.issuer, this.verified, this.innerClaim, value);
}
/**
* This instance is equal to all instances of {@code ImmutableJWTClaim} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableJWTClaim
&& equalTo((ImmutableJWTClaim) another);
}
private boolean equalTo(ImmutableJWTClaim another) {
return name.equals(another.name)
&& value.equals(another.value)
&& issuer.equals(another.issuer)
&& verified == another.verified
&& Objects.equals(innerClaim, another.innerClaim)
&& depth == another.depth;
}
/**
* Computes a hash code from attributes: {@code name}, {@code value}, {@code issuer}, {@code verified}, {@code innerClaim}, {@code depth}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + name.hashCode();
h += (h << 5) + value.hashCode();
h += (h << 5) + issuer.hashCode();
h += (h << 5) + Booleans.hashCode(verified);
h += (h << 5) + Objects.hashCode(innerClaim);
h += (h << 5) + depth;
return h;
}
/**
* Prints the immutable value {@code JWTClaim} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("JWTClaim")
.omitNullValues()
.add("name", name)
.add("value", value)
.add("issuer", issuer)
.add("verified", verified)
.add("innerClaim", innerClaim)
.add("depth", depth)
.toString();
}
/**
* Creates an immutable copy of a {@link JWTClaim} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable JWTClaim instance
*/
public static ImmutableJWTClaim copyOf(JWTClaim instance) {
if (instance instanceof ImmutableJWTClaim) {
return (ImmutableJWTClaim) instance;
}
return ImmutableJWTClaim.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableJWTClaim ImmutableJWTClaim}.
* @return A new ImmutableJWTClaim builder
*/
public static ImmutableJWTClaim.Builder builder() {
return new ImmutableJWTClaim.Builder();
}
/**
* Builds instances of type {@link ImmutableJWTClaim ImmutableJWTClaim}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_VALUE = 0x2L;
private static final long OPT_BIT_VERIFIED = 0x1L;
private static final long OPT_BIT_DEPTH = 0x2L;
private long initBits = 0x3L;
private long optBits;
private @Nullable String name;
private @Nullable String value;
private @Nullable String issuer;
private boolean verified;
private @Nullable JWTClaim innerClaim;
private int depth;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code JWTClaim} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(JWTClaim instance) {
Objects.requireNonNull(instance, "instance");
name(instance.name());
value(instance.value());
issuer(instance.issuer());
verified(instance.verified());
Optional innerClaimOptional = instance.innerClaim();
if (innerClaimOptional.isPresent()) {
innerClaim(innerClaimOptional);
}
depth(instance.depth());
return this;
}
/**
* Initializes the value for the {@link JWTClaim#name() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link JWTClaim#value() value} attribute.
* @param value The value for value
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder value(String value) {
this.value = Objects.requireNonNull(value, "value");
initBits &= ~INIT_BIT_VALUE;
return this;
}
/**
* Initializes the value for the {@link JWTClaim#issuer() issuer} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link JWTClaim#issuer() issuer}.
* @param issuer The value for issuer
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder issuer(String issuer) {
this.issuer = Objects.requireNonNull(issuer, "issuer");
return this;
}
/**
* Initializes the value for the {@link JWTClaim#verified() verified} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link JWTClaim#verified() verified}.
* @param verified The value for verified
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder verified(boolean verified) {
this.verified = verified;
optBits |= OPT_BIT_VERIFIED;
return this;
}
/**
* Initializes the optional value {@link JWTClaim#innerClaim() innerClaim} to innerClaim.
* @param innerClaim The value for innerClaim
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder innerClaim(JWTClaim innerClaim) {
this.innerClaim = Objects.requireNonNull(innerClaim, "innerClaim");
return this;
}
/**
* Initializes the optional value {@link JWTClaim#innerClaim() innerClaim} to innerClaim.
* @param innerClaim The value for innerClaim
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder innerClaim(Optional extends JWTClaim> innerClaim) {
this.innerClaim = innerClaim.orElse(null);
return this;
}
/**
* Initializes the value for the {@link JWTClaim#depth() depth} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link JWTClaim#depth() depth}.
* @param depth The value for depth
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder depth(int depth) {
this.depth = depth;
optBits |= OPT_BIT_DEPTH;
return this;
}
/**
* Builds a new {@link ImmutableJWTClaim ImmutableJWTClaim}.
* @return An immutable instance of JWTClaim
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableJWTClaim build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableJWTClaim(this);
}
private boolean verifiedIsSet() {
return (optBits & OPT_BIT_VERIFIED) != 0;
}
private boolean depthIsSet() {
return (optBits & OPT_BIT_DEPTH) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_VALUE) != 0) attributes.add("value");
return "Cannot build JWTClaim, some of required attributes are not set " + attributes;
}
}
}