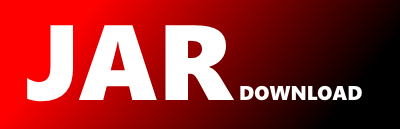
com.mercateo.common.rest.schemagen.link.BeanParamExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common.rest.schemagen Show documentation
Show all versions of common.rest.schemagen Show documentation
Jersey add-on for dynamic link and schema building
/*
* Created on 01.06.2015
*
* author joerg_adler
*/
package com.mercateo.common.rest.schemagen.link;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Collectors;
import javax.ws.rs.PathParam;
import javax.ws.rs.QueryParam;
import org.reflections.ReflectionUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.Multimap;
public class BeanParamExtractor {
private final static Logger logger = LoggerFactory.getLogger(BeanParamExtractor.class);
public Map getQueryParameters(Object bean) {
return getQueryParameters(bean, QueryParam.class, QueryParam::value, false).asMap()
.entrySet().stream().map(this::transformAll).collect(
Collectors.toMap(Param::getName, Param::getValue, (a, b) -> a));
}
private Param
© 2015 - 2025 Weber Informatics LLC | Privacy Policy