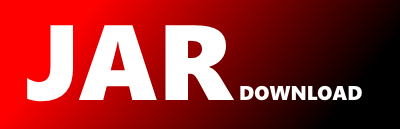
com.mercateo.common.rest.schemagen.parameter.Parameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common.rest.schemagen Show documentation
Show all versions of common.rest.schemagen Show documentation
Jersey add-on for dynamic link and schema building
package com.mercateo.common.rest.schemagen.parameter;
import static com.google.common.base.Preconditions.checkState;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.function.Function;
public class Parameter {
private final CallContext context;
private final List allowedValues;
private final T defaultValue;
private Parameter(CallContext context, List allowedValues, T defaultValue) {
this.context = context;
this.allowedValues = allowedValues;
this.defaultValue = defaultValue;
checkState(get() != null,
"parameter should have at least one allowed or default value set");
checkState(!context.hasParameter(get()), "parameter values can only be used once");
context.addParameter(get(), this);
}
public static CallContext createContext() {
return new CallContext();
}
public static Builder builderFor(Class parameterClass) {
return createContext().builderFor(parameterClass);
}
public CallContext context() {
return context;
}
public T get() {
return allowedValues.isEmpty() ? defaultValue : allowedValues.get(0);
}
public boolean hasAllowedValues() {
return !allowedValues.isEmpty();
}
public List getAllowedValues() {
return allowedValues;
}
public boolean hasDefaultValue() {
return defaultValue != null;
}
public Object getDefaultValue() {
checkState(defaultValue != null, "default value null can not be used");
return defaultValue;
}
public static class Builder {
private final Class parameterClass;
private final CallContext context;
private final List allowedValues;
private boolean nothingAllowed;
private T defaultValue;
Builder(Class parameterClass, CallContext context) {
this.parameterClass = parameterClass;
this.context = context;
this.allowedValues = new ArrayList<>();
this.nothingAllowed = false;
}
@SafeVarargs
public final Builder allowValues(T... allowedValues) {
if (allowedValues.length == 0) {
nothingAllowed = true;
}
for (T allowedValue : allowedValues) {
if (allowedValue != null) {
this.allowedValues.add(allowedValue);
}
}
return this;
}
public Builder allowValues(Collection values, Function mapper) {
allowValues(values.stream().map(mapper).toArray(this::createArray));
return this;
}
@SuppressWarnings("unchecked")
private T[] createArray(int size) {
// noinspection unchecked
return (T[]) Array.newInstance(parameterClass, size);
}
public Builder defaultValue(T defaultValue) {
this.defaultValue = defaultValue;
return this;
}
public Parameter build() {
return new Parameter<>(context, nothingAllowed ? Collections.emptyList()
: allowedValues, defaultValue);
}
public boolean isEmpty() {
return nothingAllowed || (allowedValues.isEmpty() && defaultValue == null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy