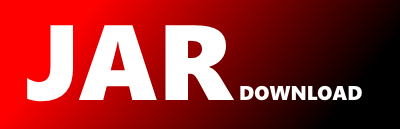
com.mesour.intellij.php.NettePhpIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of intellij-utils Show documentation
Show all versions of intellij-utils Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
The newest version!
package com.mesour.intellij.php;
import com.intellij.codeInsight.completion.PrefixMatcher;
import com.intellij.openapi.project.Project;
import com.jetbrains.php.PhpIndex;
import com.jetbrains.php.lang.psi.elements.Function;
import com.jetbrains.php.lang.psi.elements.PhpClass;
import com.jetbrains.php.lang.psi.elements.PhpNamedElement;
import com.jetbrains.php.lang.psi.elements.PhpNamespace;
import gnu.trove.THashSet;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.ArrayList;
import java.util.Collection;
public class NettePhpIndex {
@NotNull
public static Collection getAllClassNamesAndInterfaces(
final @NotNull Project project,
final @NotNull Collection classNames
) {
Collection variants = new THashSet<>();
PhpIndex phpIndex = getPhpIndex(project);
for (String name : classNames) {
variants.addAll(filterClasses(phpIndex.getClassesByName(name), null));
variants.addAll(filterClasses(phpIndex.getInterfacesByName(name), null));
}
return variants;
}
@NotNull
public static Collection getAllFunctions(
final @NotNull Project project,
final @NotNull Collection functionNames
) {
Collection variants = new THashSet<>();
PhpIndex phpIndex = getPhpIndex(project);
for (String name : functionNames) {
variants.addAll(phpIndex.getFunctionsByName(name));
}
return variants;
}
@NotNull
private static Collection filterClasses(
final @NotNull Collection classes,
final @Nullable String namespace
) {
if (namespace == null) {
return classes;
}
String normalized = "\\" + namespace + "\\";
Collection result = new ArrayList<>();
for (PhpClass cls : classes) {
String classNs = cls.getNamespaceName();
if (classNs.equals(normalized) || classNs.startsWith(normalized)) {
result.add(cls);
}
}
return result;
}
@NotNull
public static Collection getClassesByFQN(final @NotNull Project project, final @NotNull String className) {
return getPhpIndex(project).getAnyByFQN(className);
}
@NotNull
public static Collection getFunctionByName(
final @NotNull Project project,
final @NotNull String functionName
) {
return getPhpIndex(project).getFunctionsByName(functionName);
}
@NotNull
public static Collection getNamespacesByName(
final @NotNull Project project,
final @NotNull String className
) {
return getPhpIndex(project).getNamespacesByName(className);
}
@NotNull
public static Collection getAllExistingFunctionNames(
final @NotNull Project project,
final @NotNull PrefixMatcher prefixMatcher
) {
return getPhpIndex(project).getAllFunctionNames(prefixMatcher);
}
@NotNull
public static Collection getAllExistingClassNames(
final @NotNull Project project,
final @NotNull PrefixMatcher prefixMatcher
) {
return getPhpIndex(project).getAllClassNames(prefixMatcher);
}
@NotNull
private static PhpIndex getPhpIndex(final @NotNull Project project) {
return PhpIndex.getInstance(project);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy