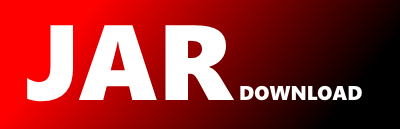
com.metaeffekt.artifact.analysis.maven.AbstractTermsMetaDataBasedMojo Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.maven;
import com.metaeffekt.artifact.analysis.flow.ng.ContentAlgorithmParam;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.licensetexts.provider.EncryptedLicenseTextProvider;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.param.ProviderParameters;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.tmd.EncryptedTermMetaDataProviderNG;
import com.metaeffekt.artifact.terms.model.LicenseTextProvider;
import com.metaeffekt.artifact.terms.model.LocalTermsMetaDataLicenseTextProvider;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import lombok.Getter;
import org.apache.maven.artifact.DefaultArtifact;
import org.apache.maven.artifact.handler.DefaultArtifactHandler;
import org.apache.maven.artifact.repository.ArtifactRepository;
import org.apache.maven.artifact.resolver.ArtifactNotFoundException;
import org.apache.maven.artifact.resolver.ArtifactResolutionException;
import org.apache.maven.artifact.resolver.ArtifactResolver;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import org.metaeffekt.core.maven.kernel.AbstractProjectAwareMojo;
import java.io.File;
import java.security.Security;
import java.util.List;
public abstract class AbstractTermsMetaDataBasedMojo extends AbstractProjectAwareMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
@Component
protected ArtifactResolver artifactResolver;
/**
* The local Maven repository where artifacts are cached during the build process.
*/
@Parameter(defaultValue = "${localRepository}", readonly = true, required = true)
private ArtifactRepository localRepository;
/**
* The file containing keys for use in decryption of the TMD database.
* Requires the corresponding {@link #userPassword} to be useful.
*/
@Parameter
protected File userKeysFile;
/**
* The password for unlocking the {@link #userKeysFile}.
*/
@Parameter
protected String userPassword;
@Parameter
String tmdGroupId;
@Parameter
String tmdArtifactId;
@Parameter
String tmdVersion;
@Getter
private transient NormalizationMetaData normalizationMetaData;
@Getter
private transient LicenseTextProvider licenseTextProvider;
/**
* A list of remote Maven repositories to be used for the compile run.
*/
@Parameter(defaultValue = "${project.remoteArtifactRepositories}")
private List remoteRepositories;
@Override
public MavenProject getProject() {
return project;
}
protected boolean configureProvider() {
final boolean managingProvider = Security.getProvider(BouncyCastleProvider.PROVIDER_NAME) == null;
if (managingProvider) {
Security.addProvider(new BouncyCastleProvider());
}
return managingProvider;
}
protected void removeProviderConfiguration(boolean managingProvider) {
if (managingProvider) {
Security.removeProvider(BouncyCastleProvider.PROVIDER_NAME);
}
}
protected NormalizationMetaData resolveTermsMetadata() throws MojoFailureException {
DefaultArtifact toSearchFor = new DefaultArtifact(
tmdGroupId, tmdArtifactId, tmdVersion, null,"zip", "package",
new DefaultArtifactHandler() {
@Override
public String getExtension() {
return "zip";
}
}
);
try {
// had some weird issue with ArtifactResolutionRequest so using this signature for now
artifactResolver.resolve(toSearchFor, remoteRepositories, localRepository);
} catch (ArtifactResolutionException | ArtifactNotFoundException e) {
throw new MojoFailureException("Could not resolve tmd artifact.", e);
}
if (!toSearchFor.isResolved()) {
throw new MojoFailureException("Tmd artifact could not be resolved.");
}
return decryptTermsMetaData(userPassword, userKeysFile, toSearchFor.getFile());
}
protected LicenseTextProvider resolveLicenseTextProvider() {
DefaultArtifact toSearchFor = new DefaultArtifact(
tmdGroupId, tmdArtifactId, tmdVersion, null, "zip", "licenses",
new DefaultArtifactHandler() {
@Override
public String getExtension() {
return "zip";
}
}
);
try {
// had some weird issue with ArtifactResolutionRequest so using this signature for now
artifactResolver.resolve(toSearchFor, remoteRepositories, localRepository);
} catch (ArtifactResolutionException | ArtifactNotFoundException e) {
// return null on failure
return null;
}
if (!toSearchFor.isResolved()) {
// return null on failure
return null;
}
final ProviderParameters providerParameters = new ProviderParameters(
new ContentAlgorithmParam(), userPassword, userKeysFile, toSearchFor.getFile());
return new EncryptedLicenseTextProvider(providerParameters);
}
public static NormalizationMetaData decryptTermsMetaData(String userPassword, File userKeysFile, File contentZipFile) throws MojoFailureException {
try {
final ProviderParameters providerParam = new ProviderParameters(
new ContentAlgorithmParam(),
userPassword, userKeysFile,
contentZipFile);
final EncryptedTermMetaDataProviderNG provider = new EncryptedTermMetaDataProviderNG();
return provider.process(providerParam);
} catch (IllegalArgumentException e) {
throw new MojoFailureException("Unable to read encrypted Terms Metadata: " + e.getMessage() + ".", e);
} catch (Exception e) {
throw new MojoFailureException("Unable to read encrypted Terms Metadata: " + e.getMessage() + ".", e);
}
}
@Override
public void execute() throws MojoFailureException {
final boolean managingProvider = Security.getProvider(BouncyCastleProvider.PROVIDER_NAME) == null;
if (managingProvider) {
Security.addProvider(new BouncyCastleProvider());
}
try {
// always initialize; as a result normalizationMetaData and LicenseTextProvider are set
initialize();
doRun();
} catch (Exception e) {
throw new MojoFailureException(e.getMessage(), e);
} finally {
if (managingProvider) {
Security.removeProvider(BouncyCastleProvider.PROVIDER_NAME);
}
}
}
private void initialize() throws MojoFailureException {
if (this.tmdArtifactId != null || this.tmdGroupId != null || this.tmdVersion != null) {
reportIfMissing(this.tmdArtifactId, "tmdArtifactId");
reportIfMissing(this.tmdGroupId, "tmdGroupId");
reportIfMissing(this.tmdVersion, "tmdVersion");
reportIfMissing(this.userKeysFile, "userKeysFile");
reportIfMissing(this.userPassword, "userPassword");
this.normalizationMetaData = resolveTermsMetadata();
this.licenseTextProvider = resolveLicenseTextProvider();
} else {
this.normalizationMetaData = NormalizationMetaData.EMPTY_NORMALIZATION_METADATA;
this.licenseTextProvider = new LocalTermsMetaDataLicenseTextProvider(getNormalizationMetaData());
}
}
private void reportIfMissing(String attribute, String attributeName) throws MojoFailureException {
if (attribute == null) {
logMissingOrInvalid(attributeName);
}
}
private void reportIfMissing(File attributeFile, String attributeName) throws MojoFailureException {
if (attributeFile == null || !attributeFile.exists()) {
logMissingOrInvalid(attributeName);
}
}
private void logMissingOrInvalid(String attributeName) throws MojoFailureException {
throw new MojoFailureException("The parameters '" + attributeName + "' is missing or invalid.");
}
protected abstract void doRun() throws Exception;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy