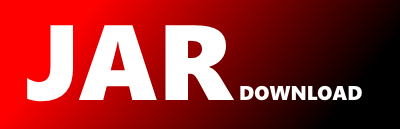
com.metaeffekt.artifact.analysis.mojo.ExtractMojo Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.mojo;
import com.metaeffekt.artifact.extractors.configuration.DirectoryScanExtractorConfiguration;
import com.metaeffekt.artifact.extractors.flow.ExtractorFlow;
import com.metaeffekt.artifact.extractors.flow.ExtractorParam;
import com.metaeffekt.artifact.extractors.flow.ExtractorResult;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.metaeffekt.core.inventory.InventoryUtils;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.metaeffekt.core.inventory.processor.writer.InventoryWriter;
import org.metaeffekt.core.maven.kernel.AbstractProjectAwareMojo;
import java.io.File;
import java.io.IOException;
/**
* This goal creates a DirectoryScanExtractorConfiguration
, which:
*
* - Read component patterns from reference inventory.
* -
* For every artifact, checks if there are component patterns available.
* Recursively copies all files that match/do not match the component pattern include/exclude paths to a tmp
* directory.
* During that scan, archives are attempted to be unarchived.
*
* - The artifact target directories are being zipped.
* - Remove all artifacts from the result inventory file that were not contained in the extraction.
*
*/
@Mojo(name = "extract-artifacts", defaultPhase = LifecyclePhase.PROCESS_RESOURCES)
public class ExtractMojo extends AbstractProjectAwareMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
@Parameter(required = true)
private String id;
/**
* The directory to scan for matching files and structure them into archives.
*/
@Parameter(required = true, defaultValue = "${project.build.directory}/scan")
private File scanBaseDir;
/**
* The reference inventory directory.
*/
@Parameter(required = true)
private File referenceInventoryDir;
/**
* The includes pattern to match the inventory files against.
* By default, this is all .xls
files in the inventory
directory.
*/
@Parameter(defaultValue = "inventory/*.xls*")
private String referenceInventoryIncludes;
/**
* The target inventory directory.
*/
@Parameter(defaultValue = "${project.build.directory}/inventory")
private File targetInventoryDir;
@Parameter(defaultValue = "${project.artifactId}-${project.version}-inventory.xls")
private String targetInventoryPath;
/**
* The directory the packed archives are written to.
*/
@Parameter(defaultValue = "${project.basedir}/.archives")
private File archiveTargetDir;
public ExtractMojo() {
}
@Override
public MavenProject getProject() {
return project;
}
@Override
public void execute() throws MojoFailureException {
try {
extract();
} catch (Exception e) {
throw new MojoFailureException(e.getMessage(), e);
}
}
private void extract() throws IOException {
final Inventory referenceInventory = InventoryUtils.readInventory(referenceInventoryDir, referenceInventoryIncludes);
final File targetInventoryFile = new File(targetInventoryDir, targetInventoryPath);
final DirectoryScanExtractorConfiguration directoryScanExtractorConfiguration =
new DirectoryScanExtractorConfiguration(id, referenceInventory, targetInventoryFile, scanBaseDir);
final ExtractorParam extractorParam = new ExtractorParam()
.using(directoryScanExtractorConfiguration)
.extractArchivesTo(archiveTargetDir);
final ExtractorFlow extractorFlow = new ExtractorFlow();
getLog().info("Extracting archives from " + scanBaseDir);
final ExtractorResult extractorResult = extractorFlow.extract(extractorParam);
new InventoryWriter().writeInventory(extractorResult.getAggregatedInventory(), targetInventoryFile);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy