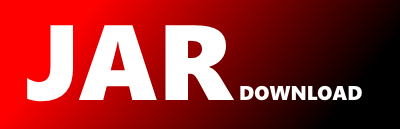
com.metaeffekt.artifact.analysis.mojo.ScanMojo Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.mojo;
import com.metaeffekt.artifact.analysis.flow.ScanFlow;
import com.metaeffekt.artifact.analysis.flow.ScanFlowParam;
import com.metaeffekt.artifact.analysis.flow.ScanFlowResult;
import com.metaeffekt.artifact.analysis.maven.AbstractTermsMetaDataBasedMojo;
import com.metaeffekt.artifact.analysis.model.DefaultPropertyProvider;
import com.metaeffekt.artifact.analysis.utils.PropertyUtils;
import com.metaeffekt.artifact.terms.model.LicenseTextProvider;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import com.metaeffekt.resource.InventoryResource;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.metaeffekt.core.inventory.processor.writer.InventoryWriter;
import java.io.File;
import java.util.Properties;
/**
* Scans the artifacts for licenses using the provided terms metadata.
*/
@Mojo(name = "scan-artifacts", defaultPhase = LifecyclePhase.COMPILE)
public class ScanMojo extends AbstractTermsMetaDataBasedMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
@Parameter(defaultValue = "${project.build.directory}/inventory")
private File targetInventoryDir;
@Parameter(defaultValue = "${project.artifactId}-${project.version}-inventory.xls")
private String targetInventoryPath;
@Parameter(defaultValue = "${project.basedir}/.archives")
private File archiveTargetDir;
@Parameter(defaultValue = "${project.basedir}/.analysis")
private File analysisBaseDir;
@Parameter(defaultValue = "src/analysis/scan-control.properties")
private File scanControlPropertiesFile;
/**
* The directory to write the inventory containing the artifacts and their matched licenses to.
* The inventory file name will contain the current milliseconds to allow for multiple scan results be written to
* the same directory.
* These inventory files can be used to generate a report using the report-artifacts
goal.
*/
@Parameter(defaultValue = "${project.basedir}/.results")
private File resultTargetDir;
@Override
public MavenProject getProject() {
return project;
}
@Override
protected void doRun() throws Exception {
// decrypt from blob
final NormalizationMetaData normalizationMetaData = getNormalizationMetaData();
final LicenseTextProvider licenseTextProvider = getLicenseTextProvider();
final DefaultPropertyProvider propertyProvider = new DefaultPropertyProvider();
final Properties properties = PropertyUtils.loadProperties(scanControlPropertiesFile);
propertyProvider.putAll(properties);
// the process uses the previously produced inventory; we should rename the parameters to convey these
// are inputs
final File targetInventoryFile = new File(targetInventoryDir, targetInventoryPath);
final ScanFlowParam param = ScanFlowParam.build()
.using(normalizationMetaData)
.using(licenseTextProvider)
.using(analysisBaseDir)
.configuredBy(propertyProvider)
.publishResultsTo(resultTargetDir)
.withInventory(InventoryResource.fromFile(targetInventoryFile));
final ScanFlow scanFlow = new ScanFlow();
getLog().info("Scanning archives in " + archiveTargetDir);
final ScanFlowResult scanFlowResult = scanFlow.process(param);
final Inventory scanInventory = scanFlowResult.getInventory();
for (Artifact artifact : scanInventory.getArtifacts()) {
final String derivedLicenses = artifact.get("Derived Licenses");
final String identifiedTerms = artifact.get("Identified Terms");
if (derivedLicenses != null || identifiedTerms != null) {
getLog().info(artifact.getId() + "<" + artifact.getChecksum() + ">:");
getLog().info(" Associated licenses: " + (artifact.getLicense() == null ? "" : artifact.getLicense()));
getLog().info(" Identified licenses: " + (derivedLicenses == null ? "" : derivedLicenses));
getLog().info(" Identified terms/markers: " + (identifiedTerms == null ? "" : identifiedTerms));
}
}
getLog().info("Updating information in " + targetInventoryFile);
new InventoryWriter().writeInventory(scanInventory, targetInventoryFile);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy