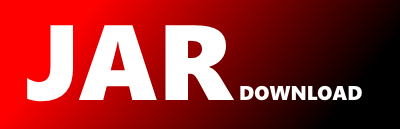
com.metaeffekt.artifact.analysis.dashboard.Sheet Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.dashboard;
import j2html.tags.DomContent;
import j2html.tags.Tag;
import j2html.tags.specialized.DivTag;
import j2html.tags.specialized.SpanTag;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import static j2html.TagCreator.*;
public class Sheet {
private String id;
private SpanTag title = span();
private final NavigationRow navigationRow = new NavigationRow();
private final List paragraphs = new ArrayList<>();
public void setId(String id) {
this.id = id;
}
public String getId() {
return id;
}
public void setTitle(String title) {
this.title = span(title);
}
public void setTitle(Tag> title) {
this.title = span(title);
}
public void addTitle(String title) {
this.title.with(text(title));
}
public void addTitle(Tag> title) {
this.title.with(title);
}
public SpanTag getEditableTitle() {
return title;
}
public NavigationRow getNavigationRow() {
return navigationRow;
}
public void addNavigationEntry(String key, DomContent value) {
navigationRow.addEntry(key, value);
}
public void addNavigationEntry(String key, Object value) {
navigationRow.addEntry(key, text(String.valueOf(value)));
}
public void addNavigationEntry(String key, DomContent value, Object additionalInfo) {
navigationRow.addEntry(key, value, additionalInfo);
}
public void addNavigationEntry(String key, Object value, Object additionalInfo) {
navigationRow.addEntry(key, text(String.valueOf(value)), additionalInfo);
}
public void addParagraph(SheetParagraph paragraph) {
if (paragraph == null) return;
this.paragraphs.add(paragraph);
}
public List getParagraphs() {
return paragraphs;
}
public DivTag getContent() {
DivTag content = div().withClasses("content-sheet", "card-design", "scrollbox");
content.with(h1(title).withStyle("margin:-8px 0px 0px 0px;"), br());
for (SheetParagraph paragraph : paragraphs) {
if (paragraph != paragraphs.get(0))
content.with(hr().withClass(paragraph.getParagraphClassIdentifier()));
content.with(paragraph.getContent());
}
content.with(
span(IntStream.range(0, 450).mapToObj(m -> "-").collect(Collectors.joining()).replaceAll("(.{108})", "$1 "))
.withStyle("color:rgba(0,0,0,0);line-height:0px;display:block;")
);
return content;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy