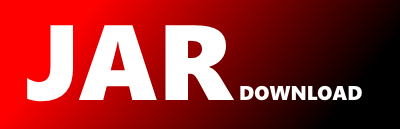
com.metaeffekt.artifact.analysis.spdxbom.config.ConfigAssembler Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.spdxbom.config;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import com.metaeffekt.artifact.analysis.spdxbom.mapper.*;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.util.*;
import java.util.regex.Pattern;
public class ConfigAssembler {
private static final Logger LOG = LoggerFactory.getLogger(ConfigAssembler.class);
protected Map nameToMapper = new HashMap<>();
protected ObjectMapper objectMapper = new ObjectMapper(new YAMLFactory());
/**
* Specifies the default "mappers", used for mapping ae artifacts to spdx items.
*/
public static final List> defaultMappers =
Arrays.asList(
MavenJarArtifactToSpdxPackage.class,
GenericArtifactToSpdxPackage.class,
NodeJsModuleArtifactToSpdxPackage.class
);
/**
* Creates a new configuration assembler.
*
* @param spdxToLicenseAssessments Map of assessments used for converting license expressions to license strings
* @param keyValueApprovedAttributes attributes approved for inclusion by key-value map
* @param normalizationMetaData required for translation of license strings
* @param mappers Mappers to use. Set to null to use default mappers, otherwise provide a complete list
*/
public ConfigAssembler(Map spdxToLicenseAssessments,
Set keyValueApprovedAttributes,
NormalizationMetaData normalizationMetaData,
List> mappers) {
if (mappers == null) {
// set default mappers if not customized
mappers = defaultMappers;
}
if (mappers.isEmpty()) {
// can't convert anything without mappers
throw new IllegalArgumentException("No mappers were provided.");
}
initMappers(spdxToLicenseAssessments, keyValueApprovedAttributes, normalizationMetaData, mappers);
}
protected void initMappers(Map spdxToLicenseStringAssessments,
Set keyValueApprovedAttributes,
NormalizationMetaData normalizationMetaData,
List> constructors) {
// register default mappers here
for (Class extends AbstractArtifactMapper> mapperClass : constructors) {
LOG.trace("Assembling mapper [{}]", mapperClass.getCanonicalName());
try {
// construct new mappers with the given classes
ArtifactMapper mapper = mapperClass
.getConstructor(
Map.class,
Set.class,
NormalizationMetaData.class)
.newInstance(
spdxToLicenseStringAssessments,
keyValueApprovedAttributes,
normalizationMetaData);
nameToMapper.put(mapper.getClass().getSimpleName(), mapper);
} catch (Exception e) {
throw new RuntimeException("Error initializing mapper '"
+ mapperClass.getSimpleName() + "'", e);
}
}
}
public List loadDefaultConfigs() {
List assembledConfigs = new ArrayList<>();
for (ArtifactMapper mapper : this.nameToMapper.values()) {
if (mapper.getDefaultConfig() != null) {
AssembledConfig assembled = assembleConfig(mapper.getDefaultConfig());
assembledConfigs.add(assembled);
}
}
return assembledConfigs;
}
protected AssembledConfig assembleConfig(StoredConfig stored) {
HashMap compiledPatterns = new HashMap<>();
for (Map.Entry entry : stored.mustMatch.entrySet()) {
compiledPatterns.put(entry.getKey(), Pattern.compile(entry.getValue()));
}
return new AssembledConfig(compiledPatterns,
nameToMapper.get(stored.mapperToUse),
stored.specificity);
}
public StoredConfig loadConfig(File file) {
try {
return objectMapper.readValue(file, StoredConfig.class);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public AssembledConfig getConfig(File file) {
return assembleConfig(loadConfig(file));
}
public void writeConfig(StoredConfig storedConfig, File file) {
try {
objectMapper.writeValue(file, storedConfig);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public void writeConfig(AssembledConfig config, File file) {
StoredConfig storedConfig = new StoredConfig();
storedConfig.mustMatch = new HashMap<>();
for (Map.Entry compiledElement : config.getMustMatch().entrySet()) {
storedConfig.mustMatch.put(compiledElement.getKey(), compiledElement.getValue().toString());
}
storedConfig.mapperToUse = config.getMapperToUse().getClass().getSimpleName();
storedConfig.specificity = config.getSpecificity();
writeConfig(storedConfig, file);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy