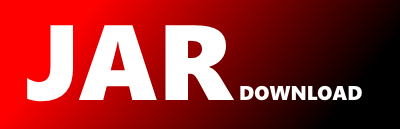
com.metaeffekt.artifact.analysis.spdxbom.context.SpdxDocumentContext Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.spdxbom.context;
import com.metaeffekt.artifact.analysis.spdxbom.SpdxDocumentSpec;
import org.apache.commons.lang3.StringUtils;
import org.spdx.library.InvalidSPDXAnalysisException;
import org.spdx.library.ModelCopyManager;
import org.spdx.library.SpdxConstants;
import org.spdx.library.model.SpdxCreatorInformation;
import org.spdx.library.model.SpdxDocument;
import org.spdx.library.model.SpdxModelFactory;
import org.spdx.storage.IModelStore;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class SpdxDocumentContext {
/**
* Wraps the document with all information.
* Also serves as the helper object for element (package etc) creation in the spdx api.
*/
private final SpdxDocument spdxDocument;
/**
* Custom spec holder with data required for document creation (such as a URI).
*/
private final SpdxDocumentSpec spdxDocumentSpec;
public SpdxDocumentContext(SpdxDocumentSpec spdxDocumentSpec, IModelStore modelStore)
throws InvalidSPDXAnalysisException {
// create document. also serves as the helper object for element (package etc) creation in spdx api
this.spdxDocument = SpdxModelFactory.createSpdxDocument(
modelStore,
spdxDocumentSpec.getDocumentUri(),
new ModelCopyManager()
);
// name / comment
spdxDocument.setName(spdxDocumentSpec.getDocumentName());
spdxDocument.setComment(spdxDocumentSpec.getDescription());
// generate creation info
final SimpleDateFormat spdxDateFormat = new SimpleDateFormat(SpdxConstants.SPDX_DATE_FORMAT);
final String dateCreated = spdxDateFormat.format(new Date());
// fill basic document information
// FIXME: allow more dynamics
final List creatorInfoEnties = new ArrayList<>();
if (StringUtils.isNotBlank(spdxDocumentSpec.getOrganization())) {
creatorInfoEnties.add("Organization: " + spdxDocumentSpec.getOrganization());
}
if (StringUtils.isNotBlank(spdxDocumentSpec.getPerson())) {
creatorInfoEnties.add("Person: " + spdxDocumentSpec.getPerson());
}
if (StringUtils.isNotBlank(spdxDocumentSpec.getTool())) {
creatorInfoEnties.add("Tool: " + spdxDocumentSpec.getTool());
}
final SpdxCreatorInformation creatorInfo = spdxDocument.createCreationInfo(creatorInfoEnties, dateCreated);
// FIXME: we need this information from the universe
creatorInfo.setLicenseListVersion("3.25");
spdxDocument.setCreationInfo(creatorInfo);
final String comment = spdxDocumentSpec.getComment();
if (StringUtils.isNotBlank(comment)) {
creatorInfo.setComment(comment);
}
this.spdxDocumentSpec = spdxDocumentSpec;
}
public SpdxDocument getSpdxDocument() {
return spdxDocument;
}
public SpdxDocumentSpec getSpdxDocumentSpec() {
return spdxDocumentSpec;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy