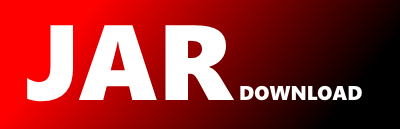
com.metaeffekt.artifact.analysis.spdxbom.facade.SpdxApiFacade Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.spdxbom.facade;
import com.metaeffekt.artifact.analysis.spdxbom.context.SpdxDocumentContext;
import org.spdx.library.InvalidSPDXAnalysisException;
import org.spdx.library.model.SpdxDocument;
import org.spdx.library.model.SpdxPackage;
import org.spdx.library.model.license.AnyLicenseInfo;
import org.spdx.library.model.license.ExtractedLicenseInfo;
import org.spdx.library.model.license.InvalidLicenseStringException;
import org.spdx.library.model.license.LicenseInfoFactory;
import org.spdx.storage.IModelStore;
import java.util.List;
import java.util.Optional;
public class SpdxApiFacade {
/**
* Get the next id using the document's model store and its getNextId method.
*
* @param suffix optional suffix to provide some detail about the object.
* @param context required for creation
*
* @return returns an id
*
* @throws InvalidSPDXAnalysisException throws whenever the document's modelStore feels like it
*/
public static String getNextSpdxId(String suffix, SpdxDocumentContext context) throws InvalidSPDXAnalysisException {
final SpdxDocument spdxDocument = context.getSpdxDocument();
String nextId = spdxDocument.getModelStore().getNextId(IModelStore.IdType.SpdxId, spdxDocument.getDocumentUri());
if (suffix != null) {
nextId += "-" + suffix;
}
return nextId;
}
/**
* Parses an object "correctly" by also passing the full context.
* Note that as of 1.1.7/1.1.8, parsing a license string may affect the state of your ModelStore.
*
* @param licenseString the licenseString to be parsed
* @param context context required to get a good parse
*
* @return returns the parsed object
*
* @throws InvalidLicenseStringException throws whenever the spdx library decides to (undocumented as of year 2023).
*/
public static AnyLicenseInfo parseLicenseString(String licenseString, SpdxDocumentContext context)
throws InvalidLicenseStringException {
return LicenseInfoFactory.parseSPDXLicenseString(
licenseString,
context.getSpdxDocument().getModelStore(),
context.getSpdxDocument().getDocumentUri(),
context.getSpdxDocument().getCopyManager()
);
}
/**
* Creates an {@link ExtractedLicenseInfo} using the context's document.
*
* Once implemented: @see SpdxDocument#createExtractedLicense(String, String)
*
* @param licenseId is an id (name) of a licenseRef
* @param text is license text to set in the returned object
* @param context needed for correctly creating the object
*
* @return returns a constructed object
*
* @throws InvalidSPDXAnalysisException throws whenever the spdx library decides to (undocumented as of year 2023).
*/
public static ExtractedLicenseInfo createExtractedLicenseInfo(String licenseId,String text,
SpdxDocumentContext context) throws InvalidSPDXAnalysisException {
// FIXME: when SpdxDocument#createExtractedLicense is available, use it instead of this constructor hack
ExtractedLicenseInfo eis = new ExtractedLicenseInfo(
context.getSpdxDocument().getModelStore(),
context.getSpdxDocument().getDocumentUri(),
licenseId,
context.getSpdxDocument().getCopyManager(),
true
);
eis.setExtractedText(text);
return eis;
}
/**
* Small helper method which fetches a spdx element by its name-field from a document
*
* @param spdxPackages a list of all spdx elements in the document
* @param name field contained in the spdx element
*
* @return the spdx element corresponding to the name or null if not present
*
* @throws InvalidSPDXAnalysisException throws whenever the spdx library decides to (undocumented as of year 2023).
*/
public static SpdxPackage getSpdxElementByName(List spdxPackages, String name) throws InvalidSPDXAnalysisException {
SpdxPackage result = null;
for (SpdxPackage spdxPackage : spdxPackages) {
final Optional optionalName = spdxPackage.getName();
final String packageName = optionalName.orElse(null);
if (packageName != null && packageName.equals(name)) {
result = spdxPackage;
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy