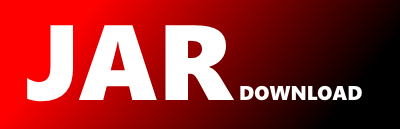
com.metaeffekt.artifact.analysis.spdxbom.hierarchy.HierarchyGraph Maven / Gradle / Ivy
package com.metaeffekt.artifact.analysis.spdxbom.hierarchy;
import org.spdx.library.model.enumerations.RelationshipType;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* This class implements a graph structure which holds an indefinite amount of nodes and edge. The graph
* maps inventory artifacts and their relationships to one another and is meant to be used in conjunction
* with the SPDX standard.
*/
public class HierarchyGraph {
public String primaryId;
private Map nodes;
private List relationships;
public HierarchyGraph() {
this.nodes = new HashMap<>();
this.relationships = new ArrayList<>();
}
public void addNode(HierarchyGraphNode node){
nodes.put(node.getId(), node);
if (node.getType() == HierarchyGraphNode.NODE_TYPE.PRIMARY){
primaryId = node.getId();
}
}
public void addRelationship(String fromId, String toId, RelationshipType relationshipType) {
HierarchyGraphNode fromNode = nodes.get(fromId);
HierarchyGraphNode toNode = nodes.get(toId);
if(fromNode != null && toNode != null){
relationships.add(new HierarchyGraphEdge(fromNode, toNode, relationshipType));
}
}
public List getDescribeRelationships() {
List describeRelationships = new ArrayList<>();
for (HierarchyGraphEdge relationship : relationships) {
if (relationship.getRelationshipType() == RelationshipType.DESCRIBES) {
describeRelationships.add(relationship);
}
}
return describeRelationships;
}
public List getRelationship(String id) {
List results = new ArrayList<>();
for (HierarchyGraphEdge edge : relationships) {
String fromNodeId = edge.getFromNode().getId();
if (fromNodeId.equals(id)) {
results.add(edge);
}
}
return results;
}
public boolean containsRelationship(String fromId, String toId, RelationshipType relationshipType) {
for (HierarchyGraphEdge edge : relationships) {
if (edge.getFromNode().getId().equals(fromId) && edge.getToNode().getId().equals(toId) && edge.getRelationshipType() == relationshipType) {
return true;
}
}
return false;
}
public List getAllRelationships() {
return relationships;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy