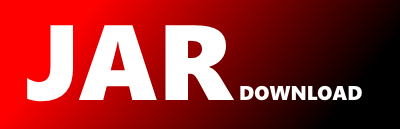
com.metaeffekt.artifact.analysis.spdxbom.mapper.NodeJsModuleArtifactToSpdxPackage Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.spdxbom.mapper;
import com.metaeffekt.artifact.analysis.spdxbom.config.StoredConfig;
import com.metaeffekt.artifact.analysis.spdxbom.context.SpdxDocumentContext;
import com.metaeffekt.artifact.analysis.spdxbom.facade.SpdxApiFacade;
import com.metaeffekt.artifact.analysis.spdxbom.holder.FillPackageHolder;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import com.metaeffekt.artifact.terms.model.TermsMetaData;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Constants;
import org.spdx.library.InvalidSPDXAnalysisException;
import org.spdx.library.SpdxConstants;
import org.spdx.library.model.SpdxDocument;
import org.spdx.library.model.SpdxPackage;
import org.spdx.library.model.enumerations.ReferenceCategory;
import org.spdx.library.model.license.SpdxNoAssertionLicense;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillAuthors;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillChecksums;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillComment;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillExternalRefWithPurl;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillHomepageFromUrl;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillOverflowKeyValueAnnotation;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillRequired;
import static com.metaeffekt.artifact.analysis.spdxbom.mapper.PackageFillers.fillVersionInfo;
public class NodeJsModuleArtifactToSpdxPackage extends AbstractArtifactMapper {
public NodeJsModuleArtifactToSpdxPackage(Map licenseStringAssessments, Set approvedAttributes, NormalizationMetaData normalizationMetaData) {
super(licenseStringAssessments, approvedAttributes, normalizationMetaData);
}
@Override
public Result getMapped(Artifact artifact, SpdxDocumentContext spdxDocumentContext) throws InvalidSPDXAnalysisException {
Set attributesWritten = new HashSet<>();
Set referencedLicenses = new HashSet<>();
final SpdxDocument spdxDocument = spdxDocumentContext.getSpdxDocument();
SpdxPackage.SpdxPackageBuilder spdxPackageBuilder = spdxDocument.createPackage(
SpdxApiFacade.getNextSpdxId(null, spdxDocumentContext),
artifact.getId(),
new SpdxNoAssertionLicense(),
SpdxConstants.NOASSERTION_VALUE,
new SpdxNoAssertionLicense()
);
// create helper to run fill methods
FillPackageHolder fillHolder = new FillPackageHolder(artifact, spdxPackageBuilder, attributesWritten);
fillRequired(fillHolder);
fillComment(fillHolder);
fillHomepageFromUrl(fillHolder);
fillExternalRefWithPurl(fillHolder, spdxDocument, this::getNodejsPurlString,
ReferenceCategory.PACKAGE_MANAGER);
fillAuthors(fillHolder);
// fill version
fillVersionInfo(fillHolder);
// fill checksums
fillChecksums(fillHolder, spdxDocument);
fillOverflowKeyValueAnnotation(fillHolder, spdxDocumentContext, this.keyValueApprovedAttributes);
SpdxPackage built = spdxPackageBuilder.build();
deriveLicensesAndCopyrights(artifact, spdxDocumentContext, attributesWritten, referencedLicenses, built);
return new Result(built, attributesWritten, referencedLicenses);
}
@Override
public StoredConfig getDefaultConfig() {
StoredConfig config = new StoredConfig();
// require id and version
config.mustMatch = new HashMap<>();
config.mustMatch.put(Artifact.Attribute.ID.getKey(), "[\\s\\S]+");
config.mustMatch.put(Artifact.Attribute.VERSION.getKey(), "[\\s\\S]+");
// require type indicating nodejs-module
// TODO: replace hardcoded attribute key string "Type" with Attribute or getter once implemented
// FIXME: we need to support several / also legacy types
config.mustMatch.put(Constants.KEY_TYPE, Constants.ARTIFACT_TYPE_NODEJS_MODULE);
config.mapperToUse = this.getClass().getSimpleName();
config.specificity = 100;
return config;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy