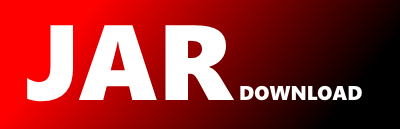
com.metaeffekt.artifact.analysis.version.curation.VersionContextMatcher Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.version.curation;
import com.metaeffekt.artifact.analysis.vulnerability.CommonEnumerationUtil;
import com.metaeffekt.artifact.analysis.vulnerability.correlation.ArtifactCorrelationEntryMatcher;
import org.json.JSONObject;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import us.springett.parsers.cpe.Cpe;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
public class VersionContextMatcher {
private final static Logger LOG = LoggerFactory.getLogger(VersionContextMatcher.class);
private final ArtifactCorrelationEntryMatcher artifactMatcher;
private final List cpeMatchers;
private final List vulnerabilities;
private final List eolIds;
private final List ghsaProducts;
public VersionContextMatcher(ArtifactCorrelationEntryMatcher artifactMatcher, List cpeMatchers, List vulnerabilities, List eolIds, List ghsaProducts) {
this.artifactMatcher = artifactMatcher;
this.cpeMatchers = cpeMatchers;
this.vulnerabilities = vulnerabilities;
this.eolIds = eolIds;
this.ghsaProducts = ghsaProducts;
}
public ArtifactCorrelationEntryMatcher getArtifactMatcher() {
return artifactMatcher;
}
public List getCpeMatchers() {
return cpeMatchers;
}
public List getVulnerabilities() {
return vulnerabilities;
}
public boolean matches(VersionContext context) {
boolean matchesArtifact = false;
if (artifactMatcher != null) {
for (Artifact artifact : context.getArtifacts()) {
if (artifactMatcher.affects(artifact)) {
matchesArtifact = true;
break;
}
}
}
boolean matchesCpe = false;
if (cpeMatchers != null) {
for (Cpe cpe : context.getCpes()) {
for (Cpe cpeMatcher : cpeMatchers) {
if (CommonEnumerationUtil.compareCpeUsingWildcards(cpe, cpeMatcher)) {
matchesCpe = true;
break;
}
}
}
}
boolean matchesVulnerability = false;
if (vulnerabilities != null) {
for (String vulnerability : context.getVulnerabilities()) {
for (String vulnerabilityMatcher : vulnerabilities) {
if (vulnerabilityMatcher.equals(vulnerability)) {
matchesVulnerability = true;
break;
}
}
}
}
boolean matchesEol = false;
if (eolIds != null) {
for (String eolId : context.getEolIds()) {
for (String eolIdMatcher : eolIds) {
if (eolIdMatcher.equals(eolId)) {
matchesEol = true;
break;
}
}
}
}
boolean matchesGhsa = false;
if (ghsaProducts != null) {
for (String ghsaProduct : context.getGhsaProducts()) {
for (String ghsaProductMatcher : ghsaProducts) {
if (ghsaProductMatcher.equals(ghsaProduct)) {
matchesGhsa = true;
break;
}
}
}
}
return (artifactMatcher == null || matchesArtifact) &&
(cpeMatchers == null || matchesCpe) &&
(vulnerabilities == null || matchesVulnerability) &&
(eolIds == null || matchesEol) &&
(ghsaProducts == null || matchesGhsa);
}
@Override
public String toString() {
return toJson().toString();
}
public static VersionContextMatcher fromYaml(Map context) {
ArtifactCorrelationEntryMatcher artifactMatcher = null;
List cpeMatchers = null;
List vulnerabilities = null;
List eolIds = null;
List ghsaProducts = null;
if (context.containsKey("artifact")) {
if (context.get("artifact") instanceof LinkedHashMap) {
artifactMatcher = ArtifactCorrelationEntryMatcher.createEntryFromYamlMap((LinkedHashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy