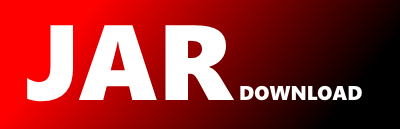
com.metaeffekt.mirror.query.CustomVulnerabilityIndexQuery Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.query;
import com.metaeffekt.mirror.contents.store.VulnerabilityTypeIdentifier;
import com.metaeffekt.mirror.contents.store.VulnerabilityTypeStore;
import com.metaeffekt.mirror.contents.vulnerability.Vulnerability;
import com.metaeffekt.mirror.contents.vulnerability.VulnerableSoftwareTreeNode;
import com.metaeffekt.mirror.contents.vulnerability.VulnerableSoftwareVersionRangeCpe;
import org.apache.commons.lang3.tuple.Pair;
import us.springett.parsers.cpe.Cpe;
import java.io.File;
import java.util.*;
import java.util.stream.Collectors;
public class CustomVulnerabilityIndexQuery extends VulnerabilityIndexQuery {
final Map vulnerabilitiesByName = new HashMap<>();
final Map> vulnerabilitiesByCpeVendorProduct = new HashMap<>();
public CustomVulnerabilityIndexQuery(Collection vulnerabilityFiles) {
super(null, null);
for (File file : vulnerabilityFiles) {
Vulnerability.fromCustomVulnerabilityFileOrDir(file).forEach(this::addVulnerability);
}
}
public CustomVulnerabilityIndexQuery(File vulnerabilityFile) {
super(null, null);
Vulnerability.fromCustomVulnerabilityFileOrDir(vulnerabilityFile).forEach(this::addVulnerability);
}
public CustomVulnerabilityIndexQuery() {
super(null, null);
}
@Override
public VulnerabilityTypeIdentifier> getVulnerabilityType() {
// TODO: Source will have to be passed into the constructor
// but this is a low prio right now, since no one uses custom vulnerabilities as of now.
return VulnerabilityTypeStore.get().fromNameAndImplementation("CUSTOM", "CUSTOM");
}
public void addVulnerability(Vulnerability vulnerability) {
vulnerabilitiesByName.put(vulnerability.getId(), vulnerability);
final List allVendorProducts = vulnerability.getVulnerableSoftwareConfigurations().stream()
.map(VulnerableSoftwareTreeNode::getAllCpes)
.flatMap(Collection::stream)
.map(cpe -> cpe.getCpe().getVendor() + ":" + cpe.getCpe().getProduct())
.collect(Collectors.toList());
for (String vp : allVendorProducts) {
vulnerabilitiesByCpeVendorProduct.computeIfAbsent(vp, k -> new ArrayList<>()).add(vulnerability);
}
}
@Override
public List findAll() {
return new ArrayList<>(vulnerabilitiesByName.values());
}
@Override
public Optional findVulnerabilityByName(String name) {
return Optional.ofNullable(vulnerabilitiesByName.get(name));
}
@Override
public List findVulnerabilitiesByFlatAffectedConfiguration(Cpe cpe) {
if (cpe == null) return new ArrayList<>();
return vulnerabilitiesByCpeVendorProduct.getOrDefault(cpe.getVendor() + ":" + cpe.getProduct(), Collections.emptyList()).stream()
.filter(v -> v.cpeFlatMatchesVulnerableSoftware(cpe))
.distinct()
.sorted(Vulnerability.COMPARE_BY_NAME)
.collect(Collectors.toList());
}
@Override
public Map findVulnerabilitiesByFlatAffectedConfigurationRetainSource(Cpe cpe) {
if (cpe == null) return new HashMap<>();
return vulnerabilitiesByCpeVendorProduct.getOrDefault(cpe.getVendor() + ":" + cpe.getProduct(), Collections.emptyList()).stream()
.map(v -> Pair.of(v, v.getCpeFlatMatchedVulnerableSoftware(cpe)))
.filter(p -> p.getRight() != null)
.collect(Collectors.toMap(Pair::getLeft, Pair::getRight));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy