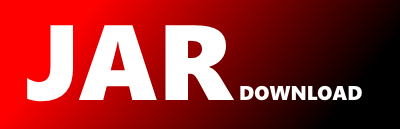
com.metaeffekt.artifact.analysis.bom.spdx.mapper.MappingUtils Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.bom.spdx.mapper;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.type.MapType;
import com.fasterxml.jackson.databind.type.TypeFactory;
import com.metaeffekt.artifact.analysis.bom.spdx.DocumentSpec;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.AssetMetaData;
import org.metaeffekt.core.inventory.processor.model.Constants;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.spdx.library.InvalidSPDXAnalysisException;
import org.spdx.library.SpdxConstants;
import org.spdx.library.model.Annotation;
import org.spdx.library.model.SpdxDocument;
import org.spdx.library.model.enumerations.AnnotationType;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MappingUtils {
/**
* Creates an annotation with a json string with the provided key-value pairs.
* This is used to represent values that aren't otherwise being mapped from our formats to spdx.
*
* @param spdxDocument context for which the annotation should be generated
* @param overflowMap the map of key-value pairs for writing
* @param documentSpec contains document metadata
*
* @return returns the created annotation for the specified document and key-value pairs
*
* @throws InvalidSPDXAnalysisException thrown if createAnnotation fails
*/
public static Annotation getOverflowAnnotation(SpdxDocument spdxDocument, DocumentSpec documentSpec,
Map overflowMap)
throws InvalidSPDXAnalysisException {
if (overflowMap.isEmpty()) {
throw new IllegalArgumentException("Map must not be empty.");
}
final MapType type = TypeFactory.defaultInstance().constructMapType(HashMap.class, String.class, String.class);
ObjectMapper objectMapper = new ObjectMapper();
String jsonString;
try {
jsonString = objectMapper.writerWithDefaultPrettyPrinter().forType(type).writeValueAsString(overflowMap);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
final SimpleDateFormat spdxDateFormat = new SimpleDateFormat(SpdxConstants.SPDX_DATE_FORMAT);
return spdxDocument.createAnnotation(
"Tool: " + documentSpec.getTool(),
AnnotationType.OTHER,
spdxDateFormat.format(new Date()),
jsonString
);
}
/**
* Helper method which assembles a map from an inventory containing assets and their correlating artifacts if there
* are any.
*
* @param inventory the inventory containing assets and artefacts
* @return the map containing asset / artifact correlations
*/
public static Map getAssetToArtifactMap(Inventory inventory) {
Map assetToArtifactMap = new HashMap<>();
List artifacts = inventory.getArtifacts();
List assetMetaDataList = inventory.getAssetMetaData();
for (Artifact artifact : artifacts) {
for (AssetMetaData assetMetaData : assetMetaDataList) {
String assetId = assetMetaData.get(AssetMetaData.Attribute.ASSET_ID);
if (artifact.get(assetId) != null && artifact.get(assetId).equals(Constants.MARKER_CROSS)) {
assetToArtifactMap.put(assetMetaData, artifact);
}
}
}
return assetToArtifactMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy