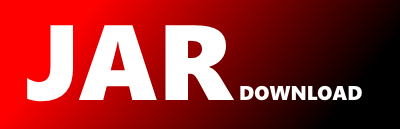
com.metaeffekt.artifact.analysis.bom.spdx.mapper.SpdxLicenseMapper Maven / Gradle / Ivy
The newest version!
package com.metaeffekt.artifact.analysis.bom.spdx.mapper;
import com.metaeffekt.artifact.analysis.bom.LicenseProcessor;
import com.metaeffekt.artifact.analysis.bom.spdx.LicenseStringConverter;
import com.metaeffekt.artifact.analysis.bom.spdx.facade.SpdxApiFacade;
import com.metaeffekt.artifact.terms.model.TermsMetaData;
import org.apache.commons.lang3.StringUtils;
import org.metaeffekt.common.notice.model.NoticeParameters;
import org.spdx.library.SpdxConstants;
import org.spdx.library.model.SpdxDocument;
import org.spdx.library.model.license.AnyLicenseInfo;
import org.spdx.library.model.license.InvalidLicenseStringException;
import java.util.*;
import java.util.stream.Collectors;
public class SpdxLicenseMapper {
public static AnyLicenseInfo deriveConcludedLicense(NoticeParameters noticeParameters,
Set referencedLicenses, SpdxDocument spdxDocument,
LicenseStringConverter licenseStringConverter, Map customLicenseMappings)
throws InvalidLicenseStringException {
if (noticeParameters != null) {
// collect effective licenses using the notice engine
List effectiveLicenses = LicenseProcessor.aggregateEffectiveLicenses(noticeParameters);
if (effectiveLicenses.isEmpty() || effectiveLicenses.stream().allMatch(StringUtils::isBlank)) {
// no effective licenses could be extracted from the notice parameter
return SpdxApiFacade.parseLicenseString(SpdxConstants.NOASSERTION_VALUE, spdxDocument);
}
return convertToLicenseInfo(spdxDocument, referencedLicenses, effectiveLicenses, licenseStringConverter, customLicenseMappings);
}
return SpdxApiFacade.parseLicenseString(SpdxConstants.NOASSERTION_VALUE, spdxDocument);
}
private static AnyLicenseInfo convertToLicenseInfo(SpdxDocument spdxDocument, Set referencedLicenses,
List effectiveLicenses, LicenseStringConverter licenseStringConverter,
Map customLicenseMappings) throws InvalidLicenseStringException {
final StringJoiner joiner = new StringJoiner(") AND (", "(", ")");
if (customLicenseMappings != null) {
Iterator iterator = effectiveLicenses.iterator();
while (iterator.hasNext()) {
String effectiveLicense = iterator.next();
if (customLicenseMappings.containsKey(effectiveLicense)) {
joiner.add("LicenseRef-" + customLicenseMappings.get(effectiveLicense));
iterator.remove(); // Safely remove the current element
}
}
}
List results = effectiveLicenses.stream()
.map(s -> licenseStringConverter.licenseStringToSpdxExpression(s))
.collect(Collectors.toList());
for (LicenseStringConverter.ToSpdxResult converterResult : results) {
// need to process intermediate results to collect
if (StringUtils.isNotBlank(converterResult.getExpression())) {
referencedLicenses.addAll(converterResult.getReferencedLicenses());
// FIXME: add support for "exception" TMD type.
// often we only find a license and some exception.
// matching them together can only work with tmd expressions, which now have "spdxExpression" fields.
// otherwise, exceptions are currently rejected by specialized checks in conversion logic and
// converted to licenseRefs so as to not crash spdx.
// see also https://github.com/spdx/spdx-spec/issues/153 but this may not help us with the core issue.
joiner.add(converterResult.getExpression());
}
}
return SpdxApiFacade.parseLicenseString(joiner.toString(), spdxDocument);
}
public static String deriveCopyrightText(NoticeParameters noticeParameters) {
if (noticeParameters != null) {
final List copyrightsList = noticeParameters.aggregateCopyrights();
if (!copyrightsList.isEmpty()) {
String copyrights = StringUtils.join(copyrightsList, "\n");
if (StringUtils.isNotBlank(copyrights)) {
return copyrights;
}
}
}
return SpdxConstants.NOASSERTION_VALUE;
}
public static AnyLicenseInfo deriveDeclaredLicense(NoticeParameters noticeParameters,
Set referencedLicenses, SpdxDocument spdxDocument,
LicenseStringConverter licenseStringConverter,
Map customLicenseMappings) throws InvalidLicenseStringException {
if (noticeParameters != null) {
// collect associated licenses using the notice parameter
List associatedLicenses = noticeParameters.aggregateAssociatedLicenses();
if (associatedLicenses.isEmpty() || associatedLicenses.stream().allMatch(StringUtils::isBlank)) {
// no effective licenses could be extracted from the notice parameter
return SpdxApiFacade.parseLicenseString(SpdxConstants.NOASSERTION_VALUE, spdxDocument);
}
return convertToLicenseInfo(spdxDocument, referencedLicenses, associatedLicenses, licenseStringConverter, customLicenseMappings);
}
return SpdxApiFacade.parseLicenseString(SpdxConstants.NOASSERTION_VALUE, spdxDocument);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy