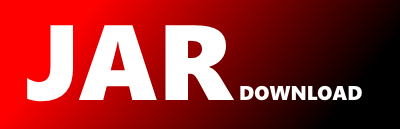
com.metaeffekt.artifact.analysis.dashboard.Modal Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.dashboard;
import j2html.tags.DomContent;
import j2html.tags.specialized.DivTag;
import j2html.tags.specialized.SpanTag;
import static j2html.TagCreator.*;
public class Modal {
private String id, title, toggleKey;
private boolean showInSidebar = true, toggleKeyActive = true;
private Size size = Size.NORMAL;
private final SpanTag content = span();
private final StringBuilder onOpenScript = new StringBuilder();
private final SidebarElement sidebarElement = new SidebarElement();
public Modal setId(String id) {
this.id = id;
this.sidebarElement.setJs("openModal('" + id + "');");
return this;
}
public String getId() {
return id;
}
public Modal setTitle(String title) {
this.title = title;
this.sidebarElement.setTitle(title);
return this;
}
public String getTitle() {
return title;
}
/**
* A JS key input name, like KeyO
*
* @param toggleKey The key.
* @return The modal instance.
*/
public Modal setToggleKey(String toggleKey) {
this.toggleKey = toggleKey;
return this;
}
public String getToggleKey() {
return toggleKey;
}
public Modal setShowInSidebar(boolean showInSidebar) {
this.showInSidebar = showInSidebar;
return this;
}
public boolean isShowInSidebar() {
return showInSidebar;
}
public Modal setToggleKeyActive(boolean toggleKeyActive) {
this.toggleKeyActive = toggleKeyActive;
return this;
}
public boolean isToggleKeyActive() {
return toggleKeyActive;
}
public Modal setSize(Size size) {
this.size = size;
return this;
}
public Size getSize() {
return size;
}
public Modal setSvgIcon(SvgIcon svgIcon) {
this.sidebarElement.setIcon(svgIcon);
return this;
}
public SidebarElement getSidebarElement() {
return sidebarElement;
}
public Modal with(DomContent... tag) {
for (DomContent addTag : tag) {
this.content.with(addTag);
}
return this;
}
public Modal with(String content) {
this.content.with(text(content));
return this;
}
public Modal withOnOpenScript(String script) {
this.onOpenScript.append(script);
return this;
}
public SpanTag getEditableContent() {
return content;
}
public DivTag generateContent() {
final String toggleKey = this.toggleKey == null ? "" : this.toggleKey;
return div().withId(id).withClass("modal").with(
div().withClasses("modal-content", "modal-content-" + size.name().toLowerCase()).with(
join(
span(
span(rawHtml("×")).attr("onclick", "closeModal('" + id + "');").withClasses("modal-close", "unselectable"),
h2(join(
title,
iff(toggleKey.length() != 0, code(" toggle key: " + toggleKey.replace("Key", "")).withStyle("font-size: 14px;"))
)),
span().withStyle("height:10px; visibility:hidden;")
),
content
)
),
script("onOpenModalScripts['" + id + "'] = () => {" + onOpenScript + "};"),
iff(!toggleKey.isEmpty() && toggleKeyActive,
script("" +
"document.addEventListener('keyup', (e) => {" +
" if (doesUserNotSelectInputElement()) {" +
" if (e.code === '" + toggleKey + "' && !e.ctrlKey && !e.metaKey) {" +
" if (!isModalVisible('" + id + "')) { openModal('" + id + "');}" +
" else { closeModal('" + id + "'); }" +
" }" +
" }" +
"});")
)
);
}
public static String makeValidJsVarName(String name) {
return name.replaceAll("[^a-zA-Z0-9]", "_");
}
public enum Size {
NORMAL,
LARGE,
ADJUST
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy