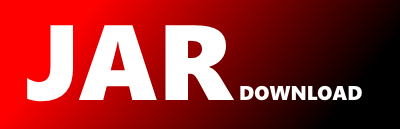
com.metaeffekt.artifact.analysis.dashboard.elements.TableBuilder Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.dashboard.elements;
import j2html.tags.DomContent;
import j2html.tags.specialized.TableTag;
import java.util.*;
import static j2html.TagCreator.*;
public class TableBuilder {
private final String tableClass;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy