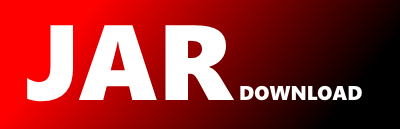
com.metaeffekt.artifact.analysis.node.NodeScanSupport Maven / Gradle / Ivy
The newest version!
package com.metaeffekt.artifact.analysis.node;
import com.metaeffekt.artifact.analysis.metascan.MetaScanSupport;
import com.metaeffekt.artifact.analysis.model.PropertyProvider;
import com.metaeffekt.artifact.analysis.utils.FileUtils;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Constants;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
public class NodeScanSupport {
private static final Logger LOG = LoggerFactory.getLogger(MetaScanSupport.class);
public static final String KEY_NAME = "name";
public static final String KEY_VERSION = "version";
public static final String VALUE_UNDEFINED = "undefined";
public static final String KEY_HOMEPAGE = "homepage";
public static final String KEY_LICENSE = "license";
public static final String KEY_TYPE = "type";
public static final String KEY_AUTHOR = "author";
public static final String KEY_AUTHOR_NAME = "name";
public static final String KEY_AUTHOR_EMAIL = "email";
private final PropertyProvider propertyProvider;
public NodeScanSupport(PropertyProvider propertyProvider) {
this.propertyProvider = propertyProvider;
}
public PropertyProvider getPropertyProvider() {
return propertyProvider;
}
public void parseJsonPackageMetaData(Artifact artifact, File analysisPath) throws IOException, JSONException {
if (analysisPath == null) return;
final boolean applyNodeJsAnalysis = getPropertyProvider().
isProperty("analyze.scan.nodejs.enabled", "true", "false");
if (!applyNodeJsAnalysis) {
return;
}
final String[] files = FileUtils.scanDirectoryForFiles(analysisPath, "*/package.json");
if (files.length == 0) {
return;
}
if (files.length > 1) {
throw new IllegalStateException(String.format("%s package.json files detected in %s.", files.length, analysisPath));
}
final File packageJsonFile = new File(analysisPath, files[0]);
final String packageJson = FileUtils.readFileToString(packageJsonFile, "UTF-8");
final JSONObject obj = new JSONObject(packageJson);
final String name = getString(obj, KEY_NAME, null);
// do not continue in case the name is not set
if (name == null) {
artifact.append("Errors", "[Json file detected, but unable to parse]", "\n");
return;
}
final String version = getString(obj, KEY_VERSION, VALUE_UNDEFINED);
artifact.set("Package Specified Id", name + "-" + version);
artifact.set("Package Specified Version", version);
artifact.set("Package Specified Type", Constants.ARTIFACT_TYPE_NODEJS_MODULE);
artifact.set("Package Specified Homepage", getString(obj, KEY_HOMEPAGE, null));
if (obj.has(KEY_LICENSE)) {
final Object licenseObj = obj.get("license");
if (licenseObj instanceof String) {
artifact.set("Package Specified Licenses", getString(obj, KEY_LICENSE, null));
} else if (obj.get(KEY_LICENSE) instanceof JSONObject) {
JSONObject licenseObjJson = obj.getJSONObject(KEY_LICENSE);
StringBuilder sb = new StringBuilder();
if (licenseObjJson.has(KEY_TYPE)) {
sb.append(getString(licenseObjJson, "type", null));
}
if (sb.length() == 0) {
artifact.append("Errors", "[Cannot parse license information from JSON license object " + licenseObj.getClass() + "]", "\n");
} else {
artifact.set("Package Specified Licenses", sb.toString());
}
} else {
artifact.append("Errors", "[Unknown JSON license type " + licenseObj.getClass() + "]", "\n");
}
}
if (obj.has("author")) {
final Object authorObject = obj.get(KEY_AUTHOR);
if (authorObject instanceof String) {
artifact.set("Package Specified Authors", getString(obj, KEY_AUTHOR, null));
} else if (authorObject instanceof JSONArray) {
JSONArray authors = obj.getJSONArray(KEY_AUTHOR);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < authors.length(); i++) {
if (sb.length() > 0) {
sb.append("|\n");
}
sb.append(authors.get(i));
}
artifact.set("Package Specified Authors", sb.toString());
} else if (authorObject instanceof JSONObject) {
JSONObject authors = obj.getJSONObject(KEY_AUTHOR);
StringBuilder author = new StringBuilder();
if (authors.has(KEY_AUTHOR_NAME)) {
author.append(getString(authors, KEY_AUTHOR_NAME, null));
}
if (authors.has("email")) {
if (author.length() > 0) {
author.append(" <");
author.append(getString(authors, KEY_AUTHOR_EMAIL, null));
author.append(">");
} else {
author.append(getString(authors, KEY_AUTHOR_EMAIL, null));
}
}
artifact.set("Package Specified Authors", author.toString());
} else {
artifact.append("Errors", "[Unknown JSON author type " + authorObject.getClass() + "]", "\n");
}
}
}
private String getString(JSONObject obj, String key, String defaultValue) {
if (obj.has(key)) {
try {
return obj.getString(key);
} catch (JSONException e) {
return defaultValue;
}
} else {
return defaultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy