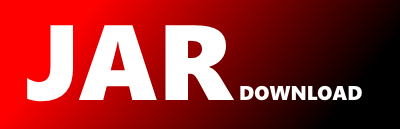
com.metaeffekt.artifact.analysis.report.ResultInventory Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.report;
import org.apache.commons.lang3.Validate;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.metaeffekt.core.inventory.processor.reader.InventoryReader;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.lang.NonNull;
import java.io.File;
import java.io.IOException;
public class ResultInventory implements Comparable {
public final static Logger LOG = LoggerFactory.getLogger(ResultInventory.class);
private Inventory inventory;
private long timestamp;
public ResultInventory from(File inventoryFile) throws IOException {
Validate.notNull(inventoryFile, "Inventory file must not be ");
Validate.isTrue(inventoryFile.exists(), String.format("Inventory file [%s] must exist.", inventoryFile.getPath()));
final String inventoryName = extractInventoryName(inventoryFile);
this.timestamp = extractTimestamp(inventoryFile, inventoryName);
this.inventory = new InventoryReader().readInventory(inventoryFile);
return this;
}
protected long extractTimestamp(File inventoryFile, String inventoryName) {
// use last modified date as default
long timestamp = inventoryFile.lastModified();
// parse timestamp from inventory name
try {
timestamp = Long.parseLong(inventoryName);
} catch (NumberFormatException e) {
LOG.error("Expecting file name to contain timestamp of creation, but was [{}].", inventoryName);
}
return timestamp;
}
protected String extractInventoryName(File inventoryFile) {
String inventoryName = inventoryFile.getName();
int endIndex = inventoryName.toLowerCase().lastIndexOf(".xls");
if (endIndex > 0) {
inventoryName = inventoryName.substring(0, endIndex);
}
return inventoryName;
}
public static ResultInventory fromFile(final File inventoryFile) throws IOException {
return new ResultInventory().from(inventoryFile);
}
public Inventory getInventory() {
return inventory;
}
public long getTimestamp() {
return timestamp;
}
@Override
public int compareTo(@NonNull ResultInventory o) {
return Long.compare(timestamp, o.timestamp);
}
@Override
public String toString() {
return "ResultInventory{" +
"artifacts=" + inventory.getArtifacts().size() +
", timestamp=" + timestamp +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy