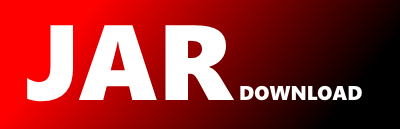
com.metaeffekt.artifact.analysis.scancode.ScanCodeLicense Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.scancode;
import java.util.List;
public class ScanCodeLicense {
private String key;
private String short_name;
private String name;
private String category;
private String owner;
private String homepage_url;
private String language;
private boolean is_exception;
private boolean is_deprecated;
private boolean is_generic;
private boolean is_unknown;
private String spdx_license_key;
private List other_spdx_license_keys;
private List text_urls;
private String faq_url;
private String osi_url;
private String osi_license_key;
private List other_urls;
private String standard_notice;
private String notes;
private String minimum_coverage;
private List ignorable_authors;
private List ignorable_copyrights;
private List ignorable_holders;
private List ignorable_urls;
private List ignorable_emails;
public void setLanguage(String language) {
this.language = language;
}
public String getLanguage() {
return language;
}
public void setIs_deprecated(boolean is_deprecated) {
this.is_deprecated = is_deprecated;
}
public boolean isIs_deprecated() {
return is_deprecated;
}
public void setIs_generic(boolean is_generic) {
this.is_generic = is_generic;
}
public boolean isIs_generic() {
return is_generic;
}
public void setOther_spdx_license_keys(List other_spdx_license_keys) {
this.other_spdx_license_keys = other_spdx_license_keys;
}
public List getOther_spdx_license_keys() {
return other_spdx_license_keys;
}
public void setOsi_license_key(String osi_license_key) {
this.osi_license_key = osi_license_key;
}
public String getOsi_license_key() {
return osi_license_key;
}
public void setOsi_url(String osi_url) {
this.osi_url = osi_url;
}
public String getOsi_url() {
return osi_url;
}
public String getMinimum_coverage() {
return minimum_coverage;
}
public void setMinimum_coverage(String minimum_coverage) {
this.minimum_coverage = minimum_coverage;
}
public void setIgnorable_emails(List ignorable_emails) {
this.ignorable_emails = ignorable_emails;
}
public List getIgnorable_emails() {
return ignorable_emails;
}
public void setIgnorable_urls(List ignorable_urls) {
this.ignorable_urls = ignorable_urls;
}
public List getIgnorable_urls() {
return ignorable_urls;
}
public List getIgnorable_holders() {
return ignorable_holders;
}
public void setIgnorable_holders(List ignorable_holders) {
this.ignorable_holders = ignorable_holders;
}
public void setIgnorable_copyrights(List ignorable_copyrights) {
this.ignorable_copyrights = ignorable_copyrights;
}
public List getIgnorable_copyrights() {
return ignorable_copyrights;
}
public void setIgnorable_authors(List ignorable_authors) {
this.ignorable_authors = ignorable_authors;
}
public List getIgnorable_authors() {
return ignorable_authors;
}
public void setFaq_url(String faq_url) {
this.faq_url = faq_url;
}
public String getFaq_url() {
return faq_url;
}
public void setNotes(String notes) {
this.notes = notes;
}
public String getNotes() {
return notes;
}
public void setStandard_notice(String standard_notice) {
this.standard_notice = standard_notice;
}
public String getStandard_notice() {
return standard_notice;
}
public void setOther_urls(List other_urls) {
this.other_urls = other_urls;
}
public List getOther_urls() {
return other_urls;
}
public List getText_urls() {
return text_urls;
}
public void setText_urls(List text_urls) {
this.text_urls = text_urls;
}
public String getSpdx_license_key() {
return spdx_license_key;
}
public void setSpdx_license_key(String spdx_license_key) {
this.spdx_license_key = spdx_license_key;
}
public void setIs_exception(boolean is_exception) {
this.is_exception = is_exception;
}
public boolean isIs_exception() {
return is_exception;
}
public void setHomepage_url(String homepage_url) {
this.homepage_url = homepage_url;
}
public String getHomepage_url() {
return homepage_url;
}
public void setOwner(String owner) {
this.owner = owner;
}
public String getOwner() {
return owner;
}
public void setCategory(String category) {
this.category = category;
}
public String getCategory() {
return category;
}
public String getShort_name() {
return short_name;
}
public void setShort_name(String short_name) {
this.short_name = short_name;
}
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public boolean isIs_unknown() {
return is_unknown;
}
public void setIs_unknown(boolean is_unknown) {
this.is_unknown = is_unknown;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy