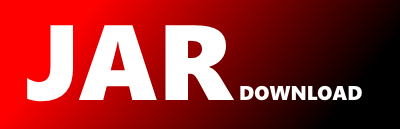
com.metaeffekt.artifact.analysis.utils.StringUtils Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.utils;
import difflib.Delta;
import difflib.DiffUtils;
import difflib.Patch;
import java.util.*;
import java.util.stream.Collectors;
/**
* General utilities for handling strings.
*/
public abstract class StringUtils {
public static final String EMPTY_STRING = "";
protected StringUtils() {
}
public static String replace(String string, Map parameter) {
for (Map.Entry entry : parameter.entrySet()) {
string = string.replace("${" + entry.getKey() + "}", entry.getValue());
}
return string;
}
public static void compare(String license, String referenceLicense) {
String lic = license.trim();
String referenceLic = referenceLicense.trim();
if (!lic.equals(referenceLic)) {
throw new IllegalArgumentException("Licenses differ.");
}
}
public static List stringToLines(String string) {
string = string.replace("\n", "***");
string = string.replace("\r", "***");
string = string.replace("******", "***");
return Arrays.asList(string.split("\\*\\*\\*"));
}
public static List compareStrings(String lhs, String rhs) {
List diff = new ArrayList<>();
Patch patch = DiffUtils.diff(stringToLines(lhs), stringToLines(rhs));
for (Delta delta : patch.getDeltas()) {
for (String line : delta.getRevised().getLines()) {
diff.add(line);
}
}
return diff;
}
/**
* Checks for empty (and blank strings)
*
* @param string String to check.
*
* @return boolean indicating whether the string contains content beyong whitespaces.
*/
public static boolean isEmpty(String string) {
return org.apache.commons.lang3.StringUtils.isBlank(string);
}
public static boolean notEmpty(String string) {
return !isEmpty(string);
}
public static String notNull(String string) {
if (string == null) return EMPTY_STRING;
return string;
}
/**
* Returns the first string being not null.
*
* @param strings The array of strings to check for null.
* @return The first string that is not null.
*/
public static String nonNull(String... strings) {
if (strings == null) return null;
for (String object : strings) {
if (object != null) return object;
}
return null;
}
public static String toString(List list) {
return list.stream().collect(Collectors.joining(", "));
}
public static List split(String s, int maxSize) {
final List split = new ArrayList<>();
String rest = s;
while (s.length() > maxSize) {
rest = s.substring(maxSize);
s = s.substring(0, maxSize);
split.add(s);
s = rest;
}
split.add(rest);
return split;
}
public static String stripArrayBoundaries(String s) {
if (s == null) return null;
s = s.trim();
if (s.startsWith("[")) s = s.substring(1, s.length() - 1);
if (s.endsWith("[")) s = s.substring(0, s.length() - 2);
return s;
}
public static boolean hasText(String s) {
return notEmpty(s);
}
public static Set splitToSet(String string, String delimiter) {
return splitToSet(string, delimiter, true);
}
public static Set splitToSet(String string, String delimiter, boolean trim) {
if (delimiter == null) {
throw new IllegalArgumentException("Delimiter must not be null.");
}
if (notEmpty(string)) {
final String[] split = string.split(delimiter);
final Set set = new LinkedHashSet<>();
for (String item : split) {
if (trim) {
set.add(item.trim());
} else {
set.add(item);
}
}
return Collections.unmodifiableSet(set);
}
return Collections.emptySet();
}
public static void addNonEmptyString(String value, Collection copyrights) {
if (StringUtils.notEmpty(value)) {
copyrights.add(value);
}
}
public static boolean equals(String lhs, String rhs) {
if (!hasText(lhs) && !hasText(rhs)) {
return true;
}
if (hasText(lhs)) {
return lhs.equals(rhs);
} else {
return rhs.equals(lhs);
}
}
public static boolean containsIgnoreCase(CharSequence seq, CharSequence searchSeq) {
return org.apache.commons.lang3.StringUtils.containsIgnoreCase(seq, searchSeq);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy