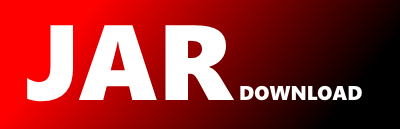
com.metaeffekt.artifact.analysis.utils.Vector Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.utils;
public class Vector implements Cloneable {
public double x, y;
public Vector() {
this(0, 0);
}
public Vector(double x, double y) {
this.x = x;
this.y = y;
}
public Vector set(double x, double y) {
this.x = x;
this.y = y;
return this;
}
public Vector set(Vector vector) {
this.x = vector.x;
this.y = vector.y;
return this;
}
public Vector add(double x, double y) {
this.x += x;
this.y += y;
return this;
}
public Vector add(Vector vector) {
this.x += vector.x;
this.y += vector.y;
return this;
}
public Vector subtract(double x, double y) {
this.x -= x;
this.y -= y;
return this;
}
public Vector subtract(Vector vector) {
this.x -= vector.x;
this.y -= vector.y;
return this;
}
public Vector divide(double n) {
this.x /= n;
this.y /= n;
return this;
}
public Vector divide(double x, double y) {
this.x /= x;
this.y /= y;
return this;
}
public Vector divide(Vector vector) {
this.x /= vector.x;
this.y /= vector.y;
return this;
}
public Vector multiply(double n) {
this.x *= n;
this.y *= n;
return this;
}
public Vector multiply(double x, double y) {
this.x *= x;
this.y *= y;
return this;
}
public Vector multiply(Vector vector) {
this.x *= vector.x;
this.y *= vector.y;
return this;
}
public Vector rotate(double angle) {
final double newX = Math.cos(Math.toRadians(angle)) * x - Math.sin(Math.toRadians(angle)) * y;
final double newY = Math.sin(Math.toRadians(angle)) * x + Math.cos(Math.toRadians(angle)) * y;
x = newX;
y = newY;
return this;
}
public double length() {
return Math.sqrt(x * x + y * y);
}
public Vector normalize() {
final double length = length();
if (length != 0) {
x /= length;
y /= length;
}
return this;
}
public boolean equals(Object o) {
if (o instanceof Vector) {
Vector v = (Vector) o;
return x == v.x && y == v.y;
}
return false;
}
public int hashCode() {
return (int) (x * 31 + y);
}
public static Vector calculateInterceptionPoint(Vector s1, Vector s2, Vector d1, Vector d2) {
double a1 = s2.y - s1.y;
double b1 = s1.x - s2.x;
double c1 = a1 * s1.x + b1 * s1.y;
double a2 = d2.y - d1.y;
double b2 = d1.x - d2.x;
double c2 = a2 * d1.x + b2 * d1.y;
double delta = a1 * b2 - a2 * b1;
return new Vector((b2 * c1 - b1 * c2) / delta, (a1 * c2 - a2 * c1) / delta);
}
@Override
public String toString() {
return "[" + x + ", " + y + "]";
}
@Override
public Vector clone() {
return new Vector(x, y);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy