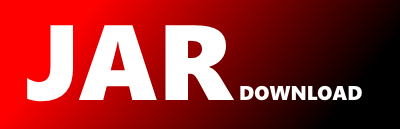
com.metaeffekt.artifact.analysis.utils.WildcardUtilities Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.utils;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public abstract class WildcardUtilities {
private final static Pattern REGEX_PATTERN_FLAGS = Pattern.compile("/[gimsuy]{1,3}$");
/**
* If the wildcard string is contained withing /.../
, the expression is converted into a regex pattern
* without escaping other characters like '.', '[', ']', etc.
* Otherwise, these characters are escaped to prevent accidental matches. In this case, these transformations are
* applied to the string:
*
* *
to .*
0+ characters
* ?
to .
1 character
*
*
*
* @param wildcardString The wildcard string to convert into a regex pattern.
* @return The converted pattern.
*/
public static Pattern convertWildcardStringToPattern(String wildcardString) {
int flags = extractWildcardFlags(wildcardString);
wildcardString = wildcardString.replaceAll(REGEX_PATTERN_FLAGS.pattern(), "/");
boolean endsInSlash = wildcardString.endsWith("/");
wildcardString = wildcardString.replaceAll("/$", "");
if (wildcardString.startsWith("/") && endsInSlash) {
return Pattern.compile(wildcardString
.replaceAll("^/", ""), flags);
} else {
return Pattern.compile(wildcardString
.replace(".", "\\.")
.replace("(", "\\(")
.replace(")", "\\)")
.replace("[", "\\[")
.replace("]", "\\]")
.replace("{", "\\{")
.replace("}", "\\}")
.replace("+", "\\+")
.replace("*", ".*")
.replace("?", "."), flags);
}
}
private static int extractWildcardFlags(String wildcardString) {
Matcher matcher = REGEX_PATTERN_FLAGS.matcher(wildcardString);
int flags = 0;
if (matcher.find()) {
for (int i = 0; i < matcher.group().length(); i++) {
if (matcher.group().charAt(i) == 'g') {
flags |= Pattern.MULTILINE;
} else if (matcher.group().charAt(i) == 'i') {
flags |= Pattern.CASE_INSENSITIVE;
} else if (matcher.group().charAt(i) == 'm') {
flags |= Pattern.MULTILINE;
} else if (matcher.group().charAt(i) == 's') {
flags |= Pattern.DOTALL;
} else if (matcher.group().charAt(i) == 'u') {
flags |= Pattern.UNICODE_CASE;
} else if (matcher.group().charAt(i) == 'y') {
flags |= Pattern.COMMENTS;
}
}
}
return flags;
}
public static boolean isWildcardPattern(String text) {
return (text.startsWith("/") && text.endsWith("/"))
|| REGEX_PATTERN_FLAGS.matcher(text).find()
|| text.contains("*")
|| text.contains("?");
}
}