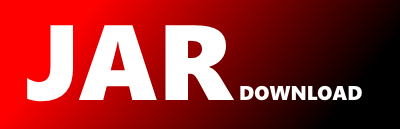
com.metaeffekt.artifact.analysis.version.curation.ConditionalCuratedVersionPartsExtractorCollection Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.version.curation;
import com.metaeffekt.artifact.analysis.dashboard.Dashboard;
import com.metaeffekt.artifact.analysis.utils.JsonSchemaValidator;
import com.metaeffekt.artifact.analysis.utils.SnakeYamlParser;
import com.networknt.schema.SpecVersion;
import org.json.JSONArray;
import org.metaeffekt.core.inventory.processor.report.configuration.CentralSecurityPolicyConfiguration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.*;
public class ConditionalCuratedVersionPartsExtractorCollection {
private final static Logger LOG = LoggerFactory.getLogger(ConditionalCuratedVersionPartsExtractorCollection.class);
private final static Map GLOBAL_NATIVE_MATCHERS = new LinkedHashMap<>();
private final List entries;
public ConditionalCuratedVersionPartsExtractorCollection(List entries) {
this.entries = entries;
}
public ConditionalCuratedVersionPartsExtractorCollection() {
this.entries = new ArrayList<>();
}
public List getEntries() {
return entries;
}
public List getMatchingExtractors(String version, VersionContext context) {
final List matchingExtractors = new ArrayList<>();
for (ConditionalCuratedVersionPartsExtractor entry : entries) {
if (entry.matches(version, context)) {
matchingExtractors.add(entry);
}
}
return matchingExtractors;
}
public JSONArray toJson() {
final JSONArray json = new JSONArray();
for (ConditionalCuratedVersionPartsExtractor entry : entries) {
json.put(entry.toJson());
}
return json;
}
@Override
public String toString() {
return toJson().toString();
}
public static ConditionalCuratedVersionPartsExtractorCollection fromYaml(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy