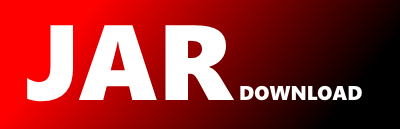
com.metaeffekt.artifact.analysis.version.curation.VersionContext Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.version.curation;
import com.metaeffekt.artifact.analysis.vulnerability.CommonEnumerationUtil;
import org.json.JSONObject;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import us.springett.parsers.cpe.Cpe;
import java.util.Collection;
import java.util.Collections;
import java.util.stream.Collectors;
public class VersionContext {
private final Collection vulnerabilities;
private final Collection eolIds;
private final Collection ghsaProducts;
private final Collection cpes;
private final Collection artifacts;
public VersionContext(Collection vulnerabilities, Collection eolIds, Collection ghsaProducts, Collection cpes, Collection artifacts) {
this.vulnerabilities = vulnerabilities;
this.eolIds = eolIds;
this.ghsaProducts = ghsaProducts;
this.cpes = cpes;
this.artifacts = artifacts;
}
public Collection getVulnerabilities() {
return vulnerabilities;
}
public Collection getEolIds() {
return eolIds;
}
public Collection getGhsaProducts() {
return ghsaProducts;
}
public Collection getCpes() {
return cpes;
}
public Collection getArtifacts() {
return artifacts;
}
public JSONObject toJson() {
JSONObject jsonObject = new JSONObject();
if (vulnerabilities != null && !vulnerabilities.isEmpty()) {
jsonObject.put("vulnerabilities", vulnerabilities);
}
if (cpes != null && !cpes.isEmpty()) {
jsonObject.put("cpes", CommonEnumerationUtil.toCpe22UriOrFallbackToCpe23FS(cpes));
}
if (artifacts != null && !artifacts.isEmpty()) {
jsonObject.put("artifacts", artifacts.stream().map(Artifact::getId).collect(Collectors.toList()));
}
if (eolIds != null && !eolIds.isEmpty()) {
jsonObject.put("eolIds", eolIds);
}
if (ghsaProducts != null && !ghsaProducts.isEmpty()) {
jsonObject.put("ghsaProducts", ghsaProducts);
}
return jsonObject;
}
@Override
public String toString() {
return toJson().toString();
}
public static VersionContext fromVulnerability(String vulnerability) {
return new VersionContext(Collections.singleton(vulnerability), Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), Collections.emptyList());
}
public static VersionContext fromEolId(String eolId) {
return new VersionContext(Collections.emptyList(), Collections.singleton(eolId), Collections.emptyList(), Collections.emptyList(), Collections.emptyList());
}
public static VersionContext fromCpe(Cpe cpe) {
return new VersionContext(Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), cpe == null ? Collections.emptyList() : Collections.singleton(cpe), Collections.emptyList());
}
public static VersionContext fromArtifact(Artifact artifact) {
return new VersionContext(Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), artifact == null ? Collections.emptyList() : Collections.singleton(artifact));
}
public static VersionContext fromGhsaProduct(String ghsaProduct) {
return new VersionContext(Collections.emptyList(), Collections.emptyList(), ghsaProduct == null ? Collections.emptyList() : Collections.singleton(ghsaProduct), Collections.emptyList(), Collections.emptyList());
}
public static VersionContext fromVulnerabilities(Collection vulnerabilities) {
return new VersionContext(vulnerabilities, Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), Collections.emptyList());
}
public static VersionContext fromCpes(Collection cpes) {
return new VersionContext(Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), cpes, Collections.emptyList());
}
public static VersionContext fromArtifacts(Collection artifacts) {
return new VersionContext(Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), artifacts);
}
public static VersionContext fromArtifactAndCpe(Artifact artifact, Cpe cpe) {
return new VersionContext(Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), Collections.singleton(cpe), Collections.singleton(artifact));
}
public final static VersionContext EMPTY = new VersionContext(Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), Collections.emptyList(), Collections.emptyList());
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy