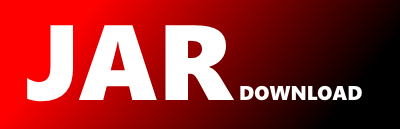
com.metaeffekt.artifact.analysis.version.curation.functions.CuratedVersionFunction Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.version.curation.functions;
import com.metaeffekt.artifact.analysis.version.curation.ExtractedCuratedVersionParts;
import com.metaeffekt.artifact.analysis.version.token.VersionToken;
import org.json.JSONObject;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.Function;
public abstract class CuratedVersionFunction {
private final String partName;
protected CuratedVersionFunction(LinkedHashMap yaml) {
this.partName = String.valueOf(yaml.get("part"));
}
public String getPartName() {
return partName;
}
public void apply(ExtractedCuratedVersionParts parts) {
switch (partName) {
case "spec":
parts.setSpecVersion(applyToPart(parts.getSpecVersion()));
break;
case "sem":
parts.setSemVersion(applyToPart(parts.getSemVersion()));
break;
case "build":
parts.setBuildVersion(applyToPart(parts.getBuildVersion()));
break;
case "modifier":
parts.setVersionModifier(applyToPart(parts.getVersionModifier()));
break;
case "other":
parts.setOtherVersionPart(applyToPart(parts.getOtherVersionPart()));
break;
case "after-all":
parts.setAfterAllPart(applyToPart(parts.getAfterAllPart()));
break;
default:
throw new IllegalArgumentException("Unsupported part: " + partName);
}
}
protected abstract VersionToken applyToPart(VersionToken specVersion);
public String getFunctionName() {
return this.getClass().getSimpleName()
.replace("CuratedVersionFunction", "")
.replaceAll("(?, CuratedVersionFunction>> CURATION_FUNCTIONS = createCurationFunctionMap();
private static Map, CuratedVersionFunction>> createCurationFunctionMap() {
final Map, CuratedVersionFunction>> map = new LinkedHashMap<>();
map.put("segments_count", CuratedVersionFunctionSegmentsCount::new);
map.put("replace", CuratedVersionFunctionReplace::new);
map.put("replace_regex", CuratedVersionFunctionReplaceRegex::new);
map.put("fill_empty", CuratedVersionFunctionFillEmpty::new);
map.put("clear_if_matches", CuratedVersionFunctionClearIfMatches::new);
return map;
}
public static void registerCurationFunction(String name, Function, CuratedVersionFunction> constructor) {
CURATION_FUNCTIONS.put(name, constructor);
}
public static void unregisterCurationFunction(String name) {
CURATION_FUNCTIONS.remove(name);
}
public static CuratedVersionFunction fromYaml(LinkedHashMap yaml) {
final String functionName = String.valueOf(yaml.get("function"));
final Function, CuratedVersionFunction> functionConstructor = CURATION_FUNCTIONS.get(functionName);
if (functionConstructor == null) {
throw new IllegalArgumentException("Unsupported curation function: " + functionName);
}
return functionConstructor.apply(yaml);
}
protected String getStringProperty(LinkedHashMap yaml, String key, String defaultValue) {
final Object value = yaml.get(key);
if (value == null) {
return defaultValue;
}
return String.valueOf(value);
}
protected String getStringPropertyOrThrow(LinkedHashMap yaml, String key) {
final Object value = yaml.get(key);
if (value == null) {
throw new IllegalArgumentException("Missing property on function " + getFunctionName() + ": " + key);
}
return String.valueOf(value);
}
protected int getIntProperty(LinkedHashMap yaml, String key, int defaultValue) {
final Object value = yaml.get(key);
if (value == null) {
return defaultValue;
}
return Integer.parseInt(String.valueOf(value));
}
protected int getIntPropertyOrThrow(LinkedHashMap yaml, String key) {
final Object value = yaml.get(key);
if (value == null) {
throw new IllegalArgumentException("Missing property on function " + getFunctionName() + ": " + key);
}
return Integer.parseInt(String.valueOf(value));
}
protected boolean getBooleanProperty(LinkedHashMap yaml, String key, boolean defaultValue) {
final Object value = yaml.get(key);
if (value == null) {
return defaultValue;
}
return Boolean.parseBoolean(String.valueOf(value));
}
protected boolean getBooleanPropertyOrThrow(LinkedHashMap yaml, String key) {
final Object value = yaml.get(key);
if (value == null) {
throw new IllegalArgumentException("Missing property on function " + getFunctionName() + ": " + key);
}
return Boolean.parseBoolean(String.valueOf(value));
}
protected LinkedHashMap getSubMap(LinkedHashMap yaml, String key) {
final Object value = yaml.get(key);
if (value == null) {
return null;
}
return (LinkedHashMap) value;
}
protected LinkedHashMap getSubMapOrThrow(LinkedHashMap yaml, String key) {
final Object value = yaml.get(key);
if (value == null) {
throw new IllegalArgumentException("Missing property on function " + getFunctionName() + ": " + key);
}
return (LinkedHashMap) value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy