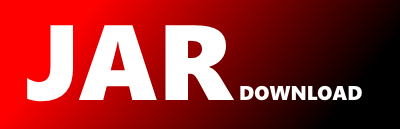
com.metaeffekt.artifact.analysis.vulnerability.enrichment.vulnerabilitystatus.VulnerabilityStatusYamlConverter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.vulnerability.enrichment.vulnerabilitystatus;
import com.metaeffekt.artifact.analysis.utils.FileUtils;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.*;
import java.util.stream.Collectors;
import static java.nio.file.StandardOpenOption.*;
import static java.nio.file.StandardOpenOption.CREATE;
import static java.nio.file.StandardOpenOption.TRUNCATE_EXISTING;
/**
* Class supporting merging vulnerability status aka assessment yaml files.
*
* The class purely operates on strings and does not operate on vulnerability status models.
*/
public class VulnerabilityStatusYamlConverter {
public static void main(String[] args) throws IOException {
final File toAggregateFiles = new File("");
final File aggregatedFiles = new File(toAggregateFiles.getParentFile(), "assessment.yaml");
aggregateAssessmentYamls(toAggregateFiles, aggregatedFiles);
}
public static void aggregateAssessmentYamls(File directory, File outputFile) throws IOException {
final List fileList = FileUtils.listFiles(directory, new String[] { "yaml" }, true)
.stream()
.sorted(Comparator.comparing(file -> file.getName().toLowerCase()))
.collect(Collectors.toList());
Files.write(Paths.get(outputFile.getAbsolutePath()), new byte[0], CREATE, TRUNCATE_EXISTING);
for (final File file : fileList) {
final List allLines = Files.readAllLines(file.toPath());
Files.write(Paths.get(outputFile.getAbsolutePath()),
("- " + allLines.get(0) + System.lineSeparator()).getBytes(), CREATE, APPEND);
for (int i = 1; i < allLines.size(); i++) {
Files.write(Paths.get(outputFile.getAbsolutePath()),
(" " + allLines.get(i) + System.lineSeparator()).getBytes(), CREATE, APPEND);
}
Files.write(Paths.get(outputFile.getAbsolutePath()), System.lineSeparator().getBytes(), CREATE, APPEND);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy