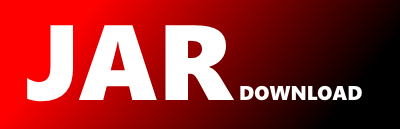
com.metaeffekt.artifact.analysis.vulnerability.enrichment.vulnerabilitystatus.validation.VulnerabilityStatusValidationEntry Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.vulnerability.enrichment.vulnerabilitystatus.validation;
import com.metaeffekt.artifact.analysis.utils.WildcardUtilities;
import com.metaeffekt.artifact.analysis.version.Version;
import com.metaeffekt.artifact.analysis.version.curation.VersionContext;
import org.metaeffekt.core.inventory.processor.model.AbstractModelBase;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import java.util.*;
import java.util.regex.Pattern;
public class VulnerabilityStatusValidationEntry {
private final String field;
private final CompareFunction function;
private final String value;
public VulnerabilityStatusValidationEntry(String field, CompareFunction function, String value) {
this.field = field;
this.function = function;
this.value = value;
}
public boolean validate(AbstractModelBase model) {
return validate(model.get(field), model);
}
/**
* Validates a field value based on a specified comparison function.
* The model is used as {@link VersionContext} when comparing versions. Optional.
*
* @param fieldValue The field value to be validated.
* @param model The model used for comparison if necessary.
* @return True if the field value is valid according to the specified comparison function, false otherwise.
* @throws IllegalStateException If the specified comparison function is unknown.
*/
public boolean validate(String fieldValue, AbstractModelBase model) {
if (fieldValue == null) {
return false;
}
switch (function) {
case EQUALS:
return value.equals(fieldValue);
case MATCHES:
return matches(fieldValue, value);
case VERSION_SMALLER:
return compareVersion(fieldValue, value, model) < 0;
case VERSION_LARGER:
return compareVersion(fieldValue, value, model) > 0;
case VERSION_LARGER_OR_EQUAL:
return compareVersion(fieldValue, value, model) >= 0;
case VERSION_SMALLER_OR_EQUAL:
return compareVersion(fieldValue, value, model) <= 0;
case NUMERIC_SMALLER:
return compareNumeric(fieldValue, value) < 0;
case NUMERIC_LARGER:
return compareNumeric(fieldValue, value) > 0;
case NUMERIC_LARGER_OR_EQUAL:
return compareNumeric(fieldValue, value) >= 0;
case NUMERIC_SMALLER_OR_EQUAL:
return compareNumeric(fieldValue, value) <= 0;
case CSV_CONTAINS:
return containsAllCsvValues(fieldValue, value);
case CSV_NOT_CONTAINS:
return !containsAllCsvValues(fieldValue, value);
default:
throw new IllegalStateException("Unknown compare function: " + function);
}
}
private boolean matches(String fieldValue, String compareValue) {
final Pattern pattern = WildcardUtilities.convertWildcardStringToPattern(compareValue);
return pattern.matcher(fieldValue).matches();
}
private int compareNumeric(String fieldValue, String compareValue) {
if (fieldValue == null || compareValue == null) {
return 0;
}
try {
return Double.compare(Double.parseDouble(fieldValue), Double.parseDouble(compareValue));
} catch (NumberFormatException e) {
return 0;
}
}
private int compareVersion(String fieldValue, String compareValue, AbstractModelBase model) {
final Artifact artifact = model instanceof Artifact ? (Artifact) model : null;
final Version fieldVersion = Version.of(fieldValue, VersionContext.fromArtifact(artifact));
final Version compareVersion = Version.of(compareValue, VersionContext.fromArtifact(artifact));
return fieldVersion.after(compareVersion) ? 1 :
fieldVersion.before(compareVersion) ? -1 :
0;
}
private boolean containsAllCsvValues(String fieldValue, String compareValue) {
final String[] fieldValues = fieldValue.split(", ");
final String[] compareValues = compareValue.split(", ");
for (String comp : compareValues) {
boolean found = false;
for (String field : fieldValues) {
if (comp.equals(field)) {
found = true;
break;
}
}
if (!found) {
return false;
}
}
return true;
}
public static List fromYamlList(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy