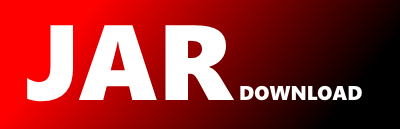
com.metaeffekt.artifact.enrichment.InventoryEnricher Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment;
import com.metaeffekt.artifact.analysis.utils.StringUtils;
import com.metaeffekt.artifact.analysis.utils.TimeUtils;
import com.metaeffekt.mirror.concurrency.ScheduledDelayedThreadPoolExecutor;
import com.metaeffekt.mirror.download.documentation.EnricherMetadata;
import com.metaeffekt.mirror.download.documentation.InventoryEnrichmentPhase;
import org.metaeffekt.core.inventory.processor.configuration.ProcessConfiguration;
import org.metaeffekt.core.inventory.processor.configuration.ProcessMisconfiguration;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.metaeffekt.core.inventory.processor.report.configuration.CentralSecurityPolicyConfiguration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
public abstract class InventoryEnricher {
private static final Logger LOG = LoggerFactory.getLogger(InventoryEnricher.class);
public final static String INVENTORY_INFO_VULNERABILITY_STATUS_KEY = "vulnerability-status";
public final static String INVENTORY_INFO_VULNERABILITY_STATUS_INVENTORY_STATUSES_KEY = "Vulnerability Inventory Status";
protected final ScheduledDelayedThreadPoolExecutor executor = new ScheduledDelayedThreadPoolExecutor(16, 0);
protected CentralSecurityPolicyConfiguration securityPolicyConfiguration;
public void performEnrichmentIfActive(Inventory inventory) {
LOG.info("");
LOG.info(formatLogHeader(getEnrichmentName()));
final long start = TimeUtils.utcNow();
if (getConfiguration().isActive()) {
LOG.info("Enriching inventory with [{} artifact{}], [{} vulnerabilit{}] and [{} advisor{}]", inventory.getArtifacts().size(), inventory.getArtifacts().size() == 1 ? "" : "s", inventory.getVulnerabilityMetaData().size(), inventory.getVulnerabilityMetaData().size() == 1 ? "y" : "ies", inventory.getAdvisoryMetaData().size(), inventory.getAdvisoryMetaData().size() == 1 ? "" : "ies");
getConfiguration().logConfiguration();
performEnrichment(inventory);
} else {
LOG.info("Skipping enrichment of inventory with [{} artifact{}], [{} vulnerabilit{}] and [{} advisor{}]", inventory.getArtifacts().size(), inventory.getArtifacts().size() == 1 ? "" : "s", inventory.getVulnerabilityMetaData().size(), inventory.getVulnerabilityMetaData().size() == 1 ? "y" : "ies", inventory.getAdvisoryMetaData().size(), inventory.getAdvisoryMetaData().size() == 1 ? "" : "ies");
}
final long duration = TimeUtils.utcNow() - start;
LOG.info(formatLogHeader("Done: " + getEnrichmentName() + " [" + TimeUtils.formatTimeDiff(duration) + "]"));
LOG.info("");
}
protected abstract void performEnrichment(Inventory inventory);
public String getEnrichmentName() {
return getMetadata().name();
}
public String getInventoryFileNameSuffix() {
return getMetadata().intermediateFileSuffix();
}
public InventoryEnrichmentPhase getPhase() {
return getMetadata().phase();
}
public boolean isDeprecated() {
return getMetadata().deprecated();
}
/**
* Set the {@link EnricherMetadata#shouldWriteIntermediateInventory} value to change default behaviour of writing
* the inventory into a file after enrichment is complete when using the {@link InventoryEnrichmentPipeline}.
* When an inventory enrichment step does not modify an inventory's content, it may not be necessary to write an
* intermediate inventory. Overwriting this method can disable this feature.
*
* @return Whether to write intermediate inventories, determined by the
* {@link EnricherMetadata#shouldWriteIntermediateInventory} value.
*/
public boolean shouldWriteIntermediateInventory() {
return getMetadata().shouldWriteIntermediateInventory();
}
public abstract ProcessConfiguration getConfiguration();
public List collectMisconfigurations() {
return getConfiguration().collectMisconfigurations();
}
public void setSecurityPolicyConfiguration(CentralSecurityPolicyConfiguration securityPolicyConfiguration) {
this.securityPolicyConfiguration = securityPolicyConfiguration;
}
public CentralSecurityPolicyConfiguration getSecurityPolicyConfiguration() {
if (securityPolicyConfiguration == null) {
this.securityPolicyConfiguration = new CentralSecurityPolicyConfiguration();
}
return securityPolicyConfiguration;
}
public boolean isSecurityPolicyConfigurationDefined() {
return securityPolicyConfiguration != null;
}
public EnricherMetadata getMetadata() {
if (getClass().isAnnotationPresent(EnricherMetadata.class)) {
return getClass().getAnnotation(EnricherMetadata.class);
} else {
throw new IllegalStateException("Enricher class " + getClass().getName() + " is missing the " + EnricherMetadata.class.getSimpleName() + " annotation.");
}
}
/* METHODS FOR MANIPULATING AND ACCESSING INVENTORY CONTENTS */
public static Set splitVulnerabilitiesCsv(String vulnerabilities) {
if (StringUtils.isEmpty(vulnerabilities)) return Collections.emptySet();
return Arrays.stream(vulnerabilities.split(", "))
.map(v -> v.replaceAll(" \\([^)]*\\)$", ""))
.map(String::trim)
.filter(StringUtils::hasText)
.collect(Collectors.toSet());
}
protected void moveInventoryData(Inventory source, Inventory destination) {
destination.setArtifacts(source.getArtifacts());
// destination.setVulnerabilityMetaData(source.getVulnerabilityMetaData());
for (String context : source.getVulnerabilityMetaDataContexts()) {
destination.setVulnerabilityMetaData(source.getVulnerabilityMetaData(context), context);
}
destination.setAdvisoryMetaData(source.getAdvisoryMetaData());
destination.setInventoryInfo(source.getInventoryInfo());
destination.setAssetMetaData(source.getAssetMetaData());
destination.setComponentNameMap(source.getComponentNameMap());
destination.setContextMap(source.getContextMap());
destination.setLicenseNameMap(source.getLicenseNameMap());
destination.setLicenseMetaData(source.getLicenseMetaData());
destination.setLicenseData(source.getLicenseData());
destination.setComponentPatternData(source.getComponentPatternData());
}
public static String formatLogHeader(String header) {
return formatLogHeader(header, 72);
}
public static String formatLogHeader(String header, int length) {
if (length < 4) {
throw new IllegalArgumentException("Length must be at least 4");
}
final StringBuilder builder = new StringBuilder();
int headerLength = header.length();
int headerLengthDiff = length - headerLength - 4;
int headerLengthDiffHalf = headerLengthDiff / 2;
for (int i = 0; i < headerLengthDiffHalf; i++) {
builder.append("-");
}
builder.append("< ").append(header).append(" >");
for (int i = 0; i < headerLengthDiffHalf; i++) {
builder.append("-");
}
while (builder.length() < length) {
builder.append("-");
}
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy