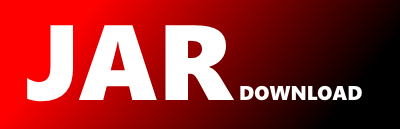
com.metaeffekt.artifact.enrichment.configurations.GhsaVulnerabilitiesEnrichmentConfiguration Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.configurations;
import com.metaeffekt.artifact.enrichment.vulnerability.ghsa.GhsaArtifactVulnerabilityMatcher;
import com.metaeffekt.artifact.enrichment.vulnerability.ghsa.GhsaEcosystem;
import com.metaeffekt.artifact.enrichment.vulnerability.ghsa.matchers.NopGhsaMatcher;
import com.metaeffekt.mirror.query.GhsaAdvisorIndexQuery;
import org.metaeffekt.core.inventory.processor.configuration.ProcessConfiguration;
import org.metaeffekt.core.inventory.processor.configuration.ProcessMisconfiguration;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import static com.metaeffekt.artifact.enrichment.vulnerability.ghsa.GhsaEcosystem.*;
public class GhsaVulnerabilitiesEnrichmentConfiguration extends ProcessConfiguration {
private boolean maven = false;
private boolean packagist = false;
private boolean rubygems = false;
private boolean githubactions = false;
private boolean pypi = false;
private boolean purl_type_swift = false;
private boolean go = false;
private boolean hex = false;
private boolean npm = false;
private boolean crates_io = false;
private boolean pub = false;
private boolean nuget = false;
private boolean githubReviewed = false;
public boolean isMaven() {
return maven;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setMaven(boolean maven) {
this.maven = maven;
return this;
}
public boolean isPackagist() {
return packagist;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setPackagist(boolean packagist) {
this.packagist = packagist;
return this;
}
public boolean isRubygems() {
return rubygems;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setRubygems(boolean rubygems) {
this.rubygems = rubygems;
return this;
}
public boolean isGithubactions() {
return githubactions;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setGithubactions(boolean githubactions) {
this.githubactions = githubactions;
return this;
}
public boolean isPypi() {
return pypi;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setPypi(boolean pypi) {
this.pypi = pypi;
return this;
}
public boolean isPurl_type_swift() {
return purl_type_swift;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setPurl_type_swift(boolean purl_type_swift) {
this.purl_type_swift = purl_type_swift;
return this;
}
public boolean isGo() {
return go;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setGo(boolean go) {
this.go = go;
return this;
}
public boolean isHex() {
return hex;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setHex(boolean hex) {
this.hex = hex;
return this;
}
public boolean isNpm() {
return npm;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setNpm(boolean npm) {
this.npm = npm;
return this;
}
public boolean isCrates_io() {
return crates_io;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setCrates_io(boolean crates_io) {
this.crates_io = crates_io;
return this;
}
public boolean isPub() {
return pub;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setPub(boolean pub) {
this.pub = pub;
return this;
}
public boolean isNuget() {
return nuget;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setNuget(boolean nuget) {
this.nuget = nuget;
return this;
}
public boolean isGithubReviewed() {
return githubReviewed;
}
public GhsaVulnerabilitiesEnrichmentConfiguration setGithubReviewed(boolean githubReviewed) {
this.githubReviewed = githubReviewed;
return this;
}
public List getActivatedEcosystems() {
final List activatedEcosystems = new ArrayList<>();
if (isMaven()) activatedEcosystems.add(MAVEN);
if (isNpm()) activatedEcosystems.add(NPM);
if (isPackagist()) activatedEcosystems.add(PACKAGIST);
if (isRubygems()) activatedEcosystems.add(RUBY_GEMS);
if (isGithubactions()) activatedEcosystems.add(GIT_HUB_ACTIONS);
if (isPypi()) activatedEcosystems.add(PY_PI);
if (isPurl_type_swift()) activatedEcosystems.add(PURL_TYPE_SWIFT);
if (isGo()) activatedEcosystems.add(GO);
if (isHex()) activatedEcosystems.add(HEX);
if (isCrates_io()) activatedEcosystems.add(CRATES_IO);
if (isPub()) activatedEcosystems.add(PUB);
if (isNuget()) activatedEcosystems.add(NU_GET);
return activatedEcosystems;
}
public List buildMatchers(GhsaAdvisorIndexQuery ghsaQuery) {
final List matchers = new ArrayList<>();
for (GhsaEcosystem ecosystem : getActivatedEcosystems()) {
matchers.add(ecosystem.createInstance(ghsaQuery, githubReviewed));
}
return matchers;
}
@Override
public LinkedHashMap getProperties() {
final LinkedHashMap properties = new LinkedHashMap<>();
properties.put("maven", maven);
properties.put("packagist", packagist);
properties.put("rubygems", rubygems);
properties.put("githubactions", githubactions);
properties.put("pypi", pypi);
properties.put("purl_type_swift", purl_type_swift);
properties.put("go", go);
properties.put("hex", hex);
properties.put("npm", npm);
properties.put("crates_io", crates_io);
properties.put("pub", pub);
properties.put("nuget", nuget);
properties.put("githubReviewed", githubReviewed);
return properties;
}
@Override
public void setProperties(LinkedHashMap properties) {
super.loadBooleanProperty(properties, "maven", this::setMaven);
super.loadBooleanProperty(properties, "packagist", this::setPackagist);
super.loadBooleanProperty(properties, "rubygems", this::setRubygems);
super.loadBooleanProperty(properties, "githubactions", this::setGithubactions);
super.loadBooleanProperty(properties, "pypi", this::setPypi);
super.loadBooleanProperty(properties, "purl_type_swift", this::setPurl_type_swift);
super.loadBooleanProperty(properties, "go", this::setGo);
super.loadBooleanProperty(properties, "hex", this::setHex);
super.loadBooleanProperty(properties, "npm", this::setNpm);
super.loadBooleanProperty(properties, "crates_io", this::setCrates_io);
super.loadBooleanProperty(properties, "pub", this::setPub);
super.loadBooleanProperty(properties, "nuget", this::setNuget);
super.loadBooleanProperty(properties, "githubReviewed", this::setGithubReviewed);
}
@Override
protected void collectMisconfigurations(List misconfigurations) {
for (GhsaEcosystem ecosystem : getActivatedEcosystems()) {
if (ecosystem.getMatcherClass() == NopGhsaMatcher.class) {
misconfigurations.add(new ProcessMisconfiguration("ecosystem", "[" + ecosystem.name() + "] ecosystem is not yet supported"));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy