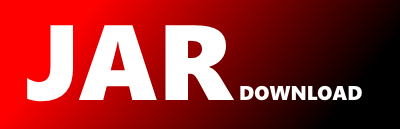
com.metaeffekt.artifact.enrichment.configurations.VulnerabilityKeywordsEnrichmentConfiguration Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.configurations;
import com.metaeffekt.artifact.analysis.vulnerability.enrichment.keywords.KeywordSet;
import com.metaeffekt.artifact.analysis.vulnerability.enrichment.keywords.VulnerabilityKeywords;
import org.metaeffekt.core.inventory.processor.configuration.ProcessConfiguration;
import org.metaeffekt.core.inventory.processor.configuration.ProcessMisconfiguration;
import org.metaeffekt.core.inventory.processor.report.configuration.CentralSecurityPolicyConfiguration;
import java.io.File;
import java.util.*;
import java.util.stream.Collectors;
public class VulnerabilityKeywordsEnrichmentConfiguration extends ProcessConfiguration {
private final List yamlFiles = new ArrayList<>();
private final List activeLabels = new ArrayList<>();
private final List addonVulnerabilityKeywords = new ArrayList<>();
private boolean failOnValidationErrors = false;
public VulnerabilityKeywordsEnrichmentConfiguration setYamlFiles(List yamlFiles) {
this.yamlFiles.clear();
this.yamlFiles.addAll(yamlFiles);
return this;
}
public VulnerabilityKeywordsEnrichmentConfiguration addYamlFile(File yamlFile) {
this.yamlFiles.add(yamlFile);
return this;
}
public VulnerabilityKeywordsEnrichmentConfiguration addActiveLabel(String... label) {
activeLabels.addAll(Arrays.asList(label));
return this;
}
public VulnerabilityKeywordsEnrichmentConfiguration addActiveLabels(Collection labels) {
activeLabels.addAll(labels);
return this;
}
public VulnerabilityKeywordsEnrichmentConfiguration setActiveLabels(Collection labels) {
activeLabels.clear();
activeLabels.addAll(labels);
return this;
}
public VulnerabilityKeywordsEnrichmentConfiguration addAddonVulnerabilityKeywords(KeywordSet addonVulnerabilityKeywords) {
this.addonVulnerabilityKeywords.add(addonVulnerabilityKeywords);
return this;
}
public VulnerabilityKeywordsEnrichmentConfiguration setFailOnValidationErrors(boolean failOnValidationErrors) {
this.failOnValidationErrors = failOnValidationErrors;
return this;
}
public VulnerabilityKeywordsEnrichmentConfiguration setAddonVulnerabilityKeywords(List addonVulnerabilityKeywords) {
this.addonVulnerabilityKeywords.clear();
this.addonVulnerabilityKeywords.addAll(addonVulnerabilityKeywords);
return this;
}
public List getYamlFiles() {
return yamlFiles;
}
public List getActiveLabels() {
return activeLabels;
}
public VulnerabilityKeywords makeKeywords(CentralSecurityPolicyConfiguration.JsonSchemaValidationErrorsHandling jsonSchemaValidationErrorsHandling) {
final VulnerabilityKeywords parsedKeywords = new VulnerabilityKeywords(yamlFiles, jsonSchemaValidationErrorsHandling);
parsedKeywords.addKeywords(addonVulnerabilityKeywords);
return parsedKeywords;
}
public boolean isFailOnValidationErrors() {
return failOnValidationErrors;
}
@Override
public LinkedHashMap getProperties() {
final LinkedHashMap properties = new LinkedHashMap<>();
properties.put("yamlFiles", yamlFiles);
properties.put("activeLabels", activeLabels);
properties.put("addonVulnerabilityKeywords", addonVulnerabilityKeywords.stream()
.filter(Objects::nonNull)
.map(KeywordSet::toFullInformationJson)
.collect(Collectors.toList()));
properties.put("failOnValidationErrors", failOnValidationErrors);
return properties;
}
@Override
public void setProperties(LinkedHashMap properties) {
super.loadListProperty(properties, "yamlFiles", obj -> new File(String.valueOf(obj)), this::setYamlFiles);
super.loadListProperty(properties, "activeLabels", String::valueOf, this::setActiveLabels);
super.loadListProperty(properties, "addonVulnerabilityKeywords", obj -> new KeywordSet().setProperties((obj instanceof Map ? new LinkedHashMap<>((Map) obj) : new LinkedHashMap<>(0))), this::setAddonVulnerabilityKeywords);
super.loadBooleanProperty(properties, "failOnValidationErrors", this::setFailOnValidationErrors);
}
@Override
protected void collectMisconfigurations(List misconfigurations) {
if (yamlFiles.isEmpty()) {
misconfigurations.add(new ProcessMisconfiguration("yamlFiles", "No YAML files configured, set enricher to inactive to avoid exception"));
}
if (yamlFiles.stream().anyMatch(Objects::isNull)) {
misconfigurations.add(new ProcessMisconfiguration("yamlFiles", "Must not contain null values"));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy